Node.js Q & A
How do you handle WebSocket timeouts in Node.js?
WebSocket timeouts in Node.js can occur due to various reasons such as network latency, client disconnects, server unavailability, or idle connections. Handling WebSocket timeouts involves implementing timeout mechanisms, monitoring connection status, and gracefully managing timeouts to ensure robust WebSocket communication. Here’s how you can handle WebSocket timeouts in Node.js:
- Implement Connection Keep-Alive:Ge
-
-
- Keep WebSocket connections alive by sending ping messages (ping-pong mechanism) at regular intervals to detect idle connections and prevent timeouts. Use WebSocket library features or custom implementations to send ping frames periodically and receive pong responses from clients.
-
- Set Timeout Thresholds:
-
-
- Set timeout thresholds for WebSocket connections based on application requirements and expected response times. Use configuration options or environment variables to specify timeout values for connection establishment, data transfer, and idle connections.
-
- Handle Connection Errors:
-
- Implement error handling mechanisms to catch and handle WebSocket connection errors, including timeouts, connection failures, and network errors. Listen for WebSocket events such as ‘error’ and ‘close’ to handle connection errors gracefully and take appropriate actions such as reconnecting, logging errors, or notifying users about connection issues.
- Retry Mechanism:
-
-
- Implement a retry mechanism to automatically reconnect WebSocket connections in case of timeouts or connection failures. Use exponential backoff strategies to gradually increase the delay between reconnection attempts and avoid overwhelming the server with connection requests.
-
- Timeout Detection:
-
-
- Monitor WebSocket connections for timeouts by tracking the time elapsed since the last message exchange or ping-pong interaction. Use timers or timeouts to detect idle connections or unexpected delays in communication and trigger timeout handling logic accordingly.
-
- Graceful Timeout Handling:
-
-
- Handle WebSocket timeouts gracefully by closing and reopening connections, notifying clients about the timeout event, or performing cleanup operations before terminating the connection. Provide appropriate error messages or status codes to indicate timeout errors to clients.
-
- Custom Timeout Handling:
-
-
- Implement custom timeout handling logic based on application-specific requirements and use cases. Consider factors such as network conditions, latency tolerance, and expected response times when defining timeout thresholds and handling timeout events.
-
- Client-Side Timeout Handling:
-
-
- Implement timeout handling logic on the client side to detect and handle WebSocket timeouts proactively. Set client-side timeouts for WebSocket operations and implement error handling mechanisms to handle timeout errors gracefully in client applications.
-
- Monitoring and Alerting:
-
-
- Monitor WebSocket connections and track timeout events using logging, metrics, and monitoring tools. Set up alerts or notifications to notify administrators or developers about WebSocket timeouts, excessive retries, or connection issues in real-time.
-
- Load Testing and Optimization:
-
- Perform load testing and optimization of WebSocket connections to identify performance bottlenecks, scalability limits, and potential causes of timeouts. Optimize server configuration, network settings, and WebSocket implementation to minimize timeouts and improve connection reliability.
By implementing robust timeout handling mechanisms, monitoring WebSocket connections proactively, and optimizing network performance, developers can ensure reliable and uninterrupted WebSocket communication in Node.js applications, enhancing real-time interaction, collaboration, and data exchange capabilities.
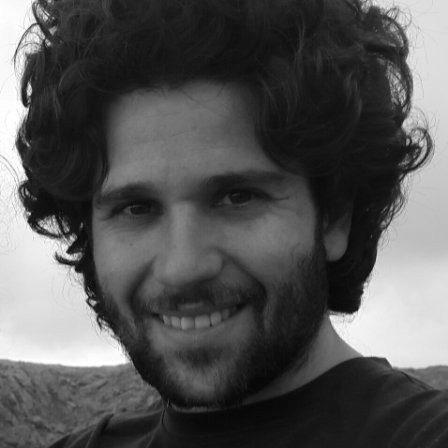
Previously at
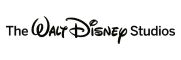
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.