Developing Real-Time Collaboration Tools with Node.js and WebSockets
In the age of remote work and digital collaboration, real-time collaboration tools have become essential for efficient teamwork. Node.js, with its non-blocking architecture, combined with WebSockets, offers a powerful solution for creating interactive and synchronized applications. This blog explores how Node.js and WebSockets can be utilized to develop real-time collaboration tools, providing practical examples to illustrate their use.
Understanding Real-Time Collaboration Tools
Real-time collaboration tools enable multiple users to interact and work together simultaneously within the same application. These tools are used in various applications, from online document editing to live chat and gaming. The key challenge in developing these tools is ensuring that all users receive updates in real-time without significant delays.
Using Node.js for Real-Time Applications
Node.js is well-suited for real-time applications due to its event-driven, non-blocking I/O model. This architecture allows Node.js to handle numerous simultaneous connections efficiently. When combined with WebSockets, Node.js can facilitate real-time communication between clients and servers.
1. Setting Up a Basic Node.js Server
To get started, you’ll need to set up a basic Node.js server. This server will handle WebSocket connections and manage real-time interactions.
Example: Creating a Simple Node.js Server
```javascript const http = require('http'); const WebSocket = require('ws'); const server = http.createServer(); const wss = new WebSocket.Server({ server }); wss.on('connection', ws => { console.log('Client connected'); ws.on('message', message => { console.log(`Received message: ${message}`); ws.send(`Echo: ${message}`); }); ws.on('close', () => { console.log('Client disconnected'); }); }); server.listen(3000, () => { console.log('Server is listening on port 3000'); }); ```
2. Implementing Real-Time Communication with WebSockets
WebSockets provide a persistent connection between the client and server, allowing for real-time data exchange. This is crucial for applications like chat systems or collaborative editing tools.
Example: Real-Time Chat Application
```javascript // Client-side JavaScript const socket = new WebSocket('ws://localhost:3000'); socket.onopen = () => { console.log('Connected to server'); }; socket.onmessage = event => { console.log(`Message from server: ${event.data}`); }; document.getElementById('sendButton').addEventListener('click', () => { const message = document.getElementById('messageInput').value; socket.send(message); }); ```
3. Synchronizing Data Across Clients
To ensure all users see the same data, you’ll need to synchronize updates across clients. This involves sending changes from one client to the server and broadcasting those changes to all connected clients.
Example: Collaborative Text Editor
```javascript // Server-side code wss.on('connection', ws => { ws.on('message', message => { // Broadcast message to all clients wss.clients.forEach(client => { if (client !== ws && client.readyState === WebSocket.OPEN) { client.send(message); } }); }); }); ```
4. Enhancing User Experience with WebSocket Features
WebSockets support advanced features such as binary data transmission and connection handling. These features can enhance the user experience by providing smoother interactions and handling more complex data.
Example: Handling Binary Data
```javascript // Client-side code const binarySocket = new WebSocket('ws://localhost:3000'); binarySocket.binaryType = 'arraybuffer'; binarySocket.onmessage = event => { const arrayBuffer = event.data; // Process binary data }; ```
5. Scaling and Deploying Real-Time Applications
As your application grows, you may need to scale your WebSocket server to handle a larger number of connections. Techniques such as load balancing and using distributed systems can help manage increased traffic.
Example: Using Redis for Pub/Sub
```javascript const redis = require('redis'); const publisher = redis.createClient(); const subscriber = redis.createClient(); subscriber.subscribe('channel'); subscriber.on('message', (channel, message) => { wss.clients.forEach(client => { if (client.readyState === WebSocket.OPEN) { client.send(message); } }); }); publisher.publish('channel', 'Hello, world!'); ```
Conclusion
Node.js and WebSockets provide a robust foundation for developing real-time collaboration tools. By leveraging Node.js’s event-driven architecture and WebSockets’ real-time communication capabilities, you can build interactive and synchronized applications that enhance teamwork and productivity. Whether you’re creating a chat application, collaborative editor, or any other real-time tool, these technologies offer powerful solutions for modern collaboration needs.
Further Reading:
Table of Contents
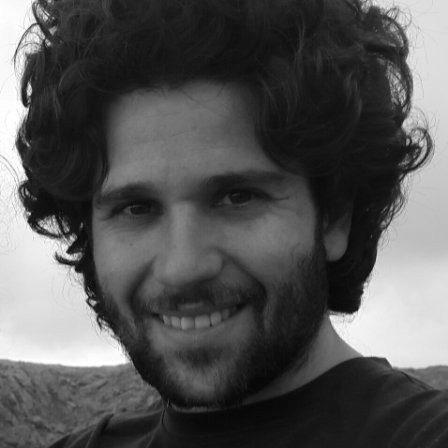
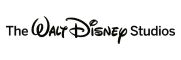