Node.js Q & A
How do you write unit tests in Node.js?
Unit testing in Node.js involves testing individual units or components of code in isolation to ensure they function correctly. This helps identify bugs early in the development process, maintain code quality, and facilitate refactoring. Several testing frameworks and libraries are available for writing unit tests in Node.js, including Mocha, Jest, Jasmine, and Ava.
Here’s a basic example of how to write unit tests using Mocha and Chai:
Install Testing Dependencies:
- bash
- Copy code
npm install mocha chai --save-dev
Write Unit Tests:
-
- Create a test file (e.g., calculator.test.js) containing your unit tests.
- javascript
- Copy code
const { expect } = require('chai'); const { add, subtract } = require('./calculator'); describe('Calculator', () => { it('should add two numbers', () => { expect(add(2, 3)).to.equal(5); }); it('should subtract two numbers', () => { expect(subtract(5, 3)).to.equal(2); }); });
Write Implementation Code:
-
- Implement the functions being tested (e.g., calculator.js).
- javascript
- Copy code
function add(a, b) { return a + b; } function subtract(a, b) { return a - b; } module.exports = { add, subtract };
Run Tests:
- Execute the tests using Mocha.
- bash
- Copy code
npx mocha
Review Test Results:
- Analyze the test results to ensure all tests pass, indicating that the units of code are functioning correctly.
By writing unit tests in Node.js, developers can verify the behavior of individual functions or modules, catch regressions, and build confidence in the correctness of their code.
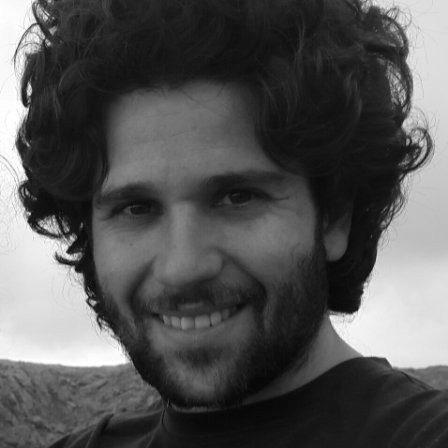
Previously at
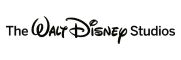
Experienced Principal Engineer and Fullstack Developer with a strong focus on Node.js. Over 5 years of Node.js development experience.