What is the purpose of the zlib module in Node.js?
The zlib module in Node.js provides compression and decompression functionalities using zlib, a widely used data compression library. It allows developers to compress data to reduce its size for efficient storage and transmission over the network and decompress compressed data for processing or display.
Key functionalities of the zlib module include:
- Compression:
-
- The zlib.gzip() method compresses input data using the gzip compression algorithm, reducing its size for efficient storage or transmission over the network.
- Example:
- javascript
- Copy code
const zlib = require('zlib'); const fs = require('fs'); const input = fs.createReadStream('input.txt'); const output = fs.createWriteStream('input.txt.gz'); input.pipe(zlib.createGzip()).pipe(output);
- Decompression:
-
- The zlib.gunzip() method decompresses gzip-compressed data, restoring it to its original uncompressed form for processing or display.
- Example:
- javascript
- Copy code
const zlib = require('zlib'); const fs = require('fs'); const input = fs.createReadStream('input.txt.gz'); const output = fs.createWriteStream('input.txt'); input.pipe(zlib.createGunzip()).pipe(output);
- Stream Compression:
-
-
- The zlib.createGzip() and zlib.createGunzip() methods return writable and readable stream instances, allowing developers to perform stream-based compression and decompression operations efficiently.
-
- Deflate and Inflate:
-
-
- The zlib.deflate() and zlib.inflate() methods provide lower-level interfaces for compression and decompression using the deflate algorithm, which is also used by gzip.
-
- Buffer Compression:
-
-
- The zlib.deflateSync() and zlib.inflateSync() methods perform synchronous compression and decompression of data stored in buffers, allowing developers to compress or decompress data without using streams.
-
- Compression Levels and Options:
-
- The `zlib module supports various compression levels and options for fine-tuning compression parameters such as compression level, window size, and strategy. Developers can specify compression options when creating compression streams or invoking compression functions.
- Error Handling:
-
-
- The zlib module provides error handling mechanisms to handle compression and decompression errors gracefully. Developers can listen for error events on compression/decompression streams or handle errors returned by compression/decompression functions.
-
- Integration with Streams and File System:
-
- The zlib module seamlessly integrates with Node.js streams and file system APIs, allowing developers to compress or decompress data stored in files, buffers, or streams efficiently.
The zlib module is commonly used in Node.js applications for compressing static assets (e.g., HTML, CSS, JavaScript files), transmitting compressed data over the network (e.g., HTTP responses), and reducing storage space for large datasets. By leveraging zlib’s compression and decompression capabilities, developers can optimize performance, reduce bandwidth usage, and improve the efficiency of data storage and transmission in Node.js applications.
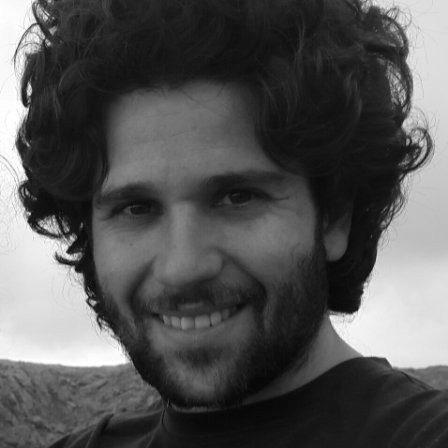
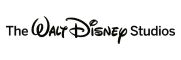