PhoneGap and App Theming: Creating Customizable Interfaces
In the fast-paced world of mobile app development, creating apps that not only look appealing but also offer a personalized user experience is paramount. One way to achieve this is through app theming, allowing users to customize the app’s interface to match their preferences. In this blog post, we will delve into the world of PhoneGap and explore how to create customizable interfaces using theming techniques.
1. What is PhoneGap?
PhoneGap, now known as Apache Cordova, is an open-source mobile development framework that allows developers to build cross-platform mobile apps using web technologies such as HTML, CSS, and JavaScript. This framework enables developers to write code once and deploy it on multiple platforms, including iOS, Android, and Windows.
2. The Importance of App Theming
Before we dive into the technical details of theming PhoneGap apps, let’s understand why app theming is essential:
2.1. User Personalization
App theming allows users to tailor the app’s appearance to their liking. Users can select colors, fonts, and other visual elements that resonate with their preferences, making the app feel like it’s uniquely theirs.
2.2. Brand Consistency
For businesses and organizations, theming ensures that the app aligns with their branding guidelines. This consistency strengthens brand identity and recognition, enhancing the overall user experience.
2.3. Improved Accessibility
Customizable interfaces can improve accessibility. Users with specific visual impairments or preferences can adjust the app’s appearance to better suit their needs.
Now that we understand why app theming is essential, let’s explore how to implement it in PhoneGap.
3. Getting Started with PhoneGap Theming
To create customizable interfaces in PhoneGap, you’ll need a good understanding of HTML, CSS, and JavaScript. Here are the steps to get started:
3.1. Set Up Your PhoneGap Project
If you haven’t already, install PhoneGap and create a new project. You can do this using the following commands:
bash npm install -g phonegap phonegap create my-app cd my-app
3.2. Create a Theming System
To implement theming, start by creating a theming system. This system should allow users to select different themes or customize individual UI elements like colors, fonts, and backgrounds.
Here’s a basic example of a theming system using JavaScript:
javascript // Define a default theme const defaultTheme = { primaryColor: '#3498db', secondaryColor: '#2ecc71', fontFamily: 'Arial, sans-serif', }; // Function to apply the theme function applyTheme(theme) { document.body.style.backgroundColor = theme.primaryColor; document.body.style.color = theme.secondaryColor; document.body.style.fontFamily = theme.fontFamily; }
In this example, we’ve defined a default theme and a function called applyTheme that applies the theme’s properties to the app’s elements.
3.3. Create a Settings Page
To allow users to customize the app’s appearance, create a settings page where they can select different themes or customize specific elements. You can use HTML forms and input elements for this purpose.
html <!DOCTYPE html> <html> <head> <!-- Include your CSS and JavaScript files --> </head> <body> <h1>App Settings</h1> <form id="theme-form"> <label for="primary-color">Primary Color:</label> <input type="color" id="primary-color" name="primary-color"><br><br> <label for="secondary-color">Secondary Color:</label> <input type="color" id="secondary-color" name="secondary-color"><br><br> <label for="font-family">Font Family:</label> <select id="font-family" name="font-family"> <option value="Arial, sans-serif">Arial</option> <option value="Helvetica, sans-serif">Helvetica</option> <option value="Times New Roman, serif">Times New Roman</option> </select><br><br> <button type="submit">Apply Theme</button> </form> </body> </html>
3.4. JavaScript for Theme Application
Now, let’s add JavaScript to handle theme selection and application:
javascript // Get the theme form const themeForm = document.getElementById('theme-form'); // Add a submit event listener themeForm.addEventListener('submit', function (e) { e.preventDefault(); // Get the selected values const primaryColor = document.getElementById('primary-color').value; const secondaryColor = document.getElementById('secondary-color').value; const fontFamily = document.getElementById('font-family').value; // Create a theme object const customTheme = { primaryColor, secondaryColor, fontFamily, }; // Apply the custom theme applyTheme(customTheme); });
This JavaScript code listens for form submissions, collects user-selected theme values, and applies the custom theme using the applyTheme function.
3.5. Applying Theming Across the App
Now that you have a theming system in place, you can apply the selected theme throughout your PhoneGap app. Here’s how you can update the app’s UI elements dynamically:
javascript // Function to apply the theme function applyTheme(theme) { document.body.style.backgroundColor = theme.primaryColor; document.body.style.color = theme.secondaryColor; document.body.style.fontFamily = theme.fontFamily; // You can extend this function to update other UI elements }
In this example, we’re updating the background color, text color, and font family of the document.body. Depending on your app’s complexity, you may need to update other elements such as buttons, headers, and paragraphs.
4. Advanced Theming Techniques
Creating a basic theming system is a great start, but you can take app theming to the next level with some advanced techniques:
4.1. Theming Variables
Use CSS variables to define theme-related properties. This makes it easier to manage and update themes. For example:
css :root { --primary-color: #3498db; --secondary-color: #2ecc71; --font-family: Arial, sans-serif; } body { background-color: var(--primary-color); color: var(--secondary-color); font-family: var(--font-family); }
4.2. Theme Storage
Allow users to save their selected themes. You can use localStorage to store and retrieve theme settings, ensuring that users see their chosen theme every time they open the app.
javascript // Save theme settings function saveThemeSettings(theme) { localStorage.setItem('theme', JSON.stringify(theme)); } // Load theme settings function loadThemeSettings() { const savedTheme = localStorage.getItem('theme'); if (savedTheme) { const theme = JSON.parse(savedTheme); applyTheme(theme); } }
Call loadThemeSettings when your app initializes to load the user’s saved theme.
4.3. Theme Presets
Offer pre-designed theme presets that users can choose from. This simplifies the customization process for users who don’t want to fine-tune every detail.
4.4. Dynamic Component Theming
If your app uses custom components, make sure they can adapt to different themes. Define CSS classes for each component’s theme and update them dynamically.
Conclusion
Creating customizable interfaces for PhoneGap apps through theming is a powerful way to enhance user engagement and satisfaction. By following the steps outlined in this guide and exploring advanced theming techniques, you can provide users with a personalized experience that aligns with their preferences and your app’s branding.
In the competitive world of mobile app development, offering customization options can set your app apart from the rest. So, start experimenting with app theming in PhoneGap today and watch your user base grow as they appreciate the flexibility and personalization your app provides.
Remember, the key to successful theming is not just providing options but also ensuring a consistent and visually appealing user experience. Balance flexibility with aesthetics, and your app will shine in the eyes of your users.
If you have any questions or want to share your experiences with PhoneGap theming, feel free to leave a comment below. Happy theming!
Table of Contents
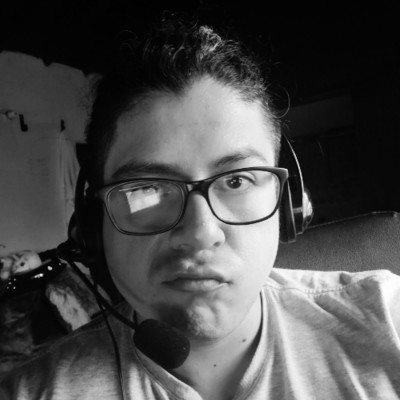
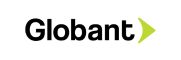