PhoneGap and Bluetooth: Building Connected Apps
In today’s fast-paced digital world, the demand for connected apps that can seamlessly communicate with external devices is on the rise. Whether you want to create a fitness app that syncs with a heart rate monitor, a home automation app that controls smart devices, or any other app that needs to interact with external hardware, PhoneGap combined with Bluetooth can be a powerful solution.
PhoneGap, a popular open-source framework for building cross-platform mobile apps using HTML, CSS, and JavaScript, offers an excellent platform for developing connected apps. Bluetooth, on the other hand, is a versatile wireless technology that allows devices to communicate wirelessly over short distances. When you combine the capabilities of PhoneGap and Bluetooth, you open up a world of possibilities for creating innovative and interactive applications.
In this comprehensive guide, we’ll delve into the world of PhoneGap and Bluetooth, exploring how you can leverage these technologies to build connected apps. We’ll cover everything from the basics of Bluetooth communication to practical code examples and best practices for creating robust and reliable apps. Let’s get started.
1. Understanding Bluetooth Technology
Before diving into the development process, it’s essential to have a basic understanding of Bluetooth technology. Bluetooth is a wireless communication standard that allows devices to exchange data over short distances using radio waves. It operates in the 2.4 GHz frequency band and is commonly used for connecting various devices, such as smartphones, tablets, headphones, keyboards, and IoT devices.
1.1. Bluetooth Versions
Bluetooth has gone through several iterations, with each version introducing improvements in terms of speed, range, and power efficiency. The most relevant Bluetooth versions for app development are:
- Bluetooth Classic (BR/EDR): This is the traditional Bluetooth version primarily used for audio streaming (e.g., connecting your phone to a Bluetooth speaker) and data transfer.
- Bluetooth Low Energy (BLE): Also known as Bluetooth Smart, BLE is designed for low-power applications, making it ideal for battery-operated devices like fitness trackers, sensors, and beacons.
1.2. Bluetooth Profiles
Bluetooth profiles define the functionality and characteristics of devices. There are various Bluetooth profiles available, each tailored for specific use cases. Some common profiles include:
- Serial Port Profile (SPP): Used for serial communication between devices.
- Hands-Free Profile (HFP): Enables communication between smartphones and hands-free car kits.
- Generic Attribute Profile (GATT): Essential for BLE communication, allowing devices to expose services and characteristics for data exchange.
1.3. Pairing and Bonding
When two Bluetooth devices want to communicate securely, they go through a process called pairing. During pairing, devices exchange encryption keys to establish a secure connection. Bonding is the process of saving these keys for future connections, eliminating the need to re-pair each time.
2. Setting Up Your Development Environment
To begin developing PhoneGap apps with Bluetooth capabilities, you’ll need to set up your development environment. Here’s what you need:
2.1. PhoneGap/Cordova Installation
If you haven’t already installed PhoneGap (also known as Apache Cordova), you can do so using npm (Node Package Manager):
bash npm install -g phonegap
Once installed, you can create a new PhoneGap project using the following command:
bash phonegap create my-app
2.2. Bluetooth Plugin
To access Bluetooth functionality in your PhoneGap app, you’ll need a Bluetooth plugin. One popular choice is the cordova-plugin-ble-central plugin, which provides BLE (Bluetooth Low Energy) support. You can add this plugin to your project like this:
bash phonegap plugin add cordova-plugin-ble-central
2.3. Development IDE
Choose a development environment that you’re comfortable with. You can use any code editor or integrated development environment (IDE) that supports web development. Popular choices include Visual Studio Code, Sublime Text, and WebStorm.
Now that you have your development environment set up, let’s dive into the practical aspects of using PhoneGap and Bluetooth to build connected apps.
3. Scanning for Bluetooth Devices
One of the fundamental tasks in building a connected app is discovering nearby Bluetooth devices. This is essential for establishing connections and interacting with external hardware. To scan for Bluetooth devices in a PhoneGap app, you can use the cordova-plugin-ble-central plugin mentioned earlier.
Here’s a step-by-step guide on how to scan for nearby Bluetooth devices:
3.1. Initialize Bluetooth
First, you need to initialize the Bluetooth plugin in your app. You can do this by adding the following code to your JavaScript file:
javascript // Wait for the device to be ready document.addEventListener('deviceready', onDeviceReady, false); // Function to run when the device is ready function onDeviceReady() { // Initialize the Bluetooth plugin ble.scan([], 5, onDiscoverDevice, onError); } // Callback function when a device is discovered function onDiscoverDevice(device) { // Handle discovered device here console.log('Discovered device:', device); } // Callback function for errors function onError(error) { // Handle errors here console.error('Error:', error); }
This code waits for the device to be ready (the ‘deviceready’ event) and then initializes the Bluetooth plugin using ble.scan. The ble.scan function takes three parameters: an array of service UUIDs to filter devices, a scan duration in seconds, and two callback functions for success and error handling.
3.2. Handle Discovered Devices
When the app discovers a nearby Bluetooth device, the onDiscoverDevice callback function is called. In this function, you can handle the discovered device. Typically, you would update the UI to display a list of available devices or perform further actions, such as connecting to the device.
3.3. Error Handling
The onError function handles errors that may occur during the scanning process. It’s crucial to implement robust error handling to provide a seamless user experience.
4. Connecting to Bluetooth Devices
Once you’ve scanned for nearby Bluetooth devices and the user selects a device to connect to, the next step is establishing a connection. In PhoneGap, you can use the cordova-plugin-ble-central plugin to connect to Bluetooth devices.
Here’s a step-by-step guide on how to connect to a Bluetooth device:
4.1. Select a Device
Before connecting to a device, you need to allow the user to select a device from the list of discovered devices. You can achieve this by creating a UI element (e.g., a list) and handling the user’s selection.
4.2. Establish a Connection
Once the user selects a device, you can use the ble.connect function to establish a connection:
javascript // Device object received from onDiscoverDevice callback var selectedDevice = ... // Connect to the selected device ble.connect(selectedDevice.id, onConnect, onError);
The ble.connect function takes the device’s ID, a success callback (onConnect), and an error callback (onError). When the connection is successfully established, the onConnect callback is invoked.
4.3. Handle Connection Events
After establishing a connection, you can communicate with the connected device by reading from and writing to its characteristics. You can also subscribe to notifications to receive updates from the device when data changes.
javascript // Callback function when the device is connected function onConnect(device) { // Handle successful connection console.log('Connected to device:', device); // Read or write to characteristics, subscribe to notifications, etc. }
5. Data Exchange with Bluetooth Devices
Once you’ve connected to a Bluetooth device, you can exchange data with it. The specific data exchange methods depend on the device and its capabilities. However, here are some common data exchange scenarios:
5.1. Reading Characteristics
You can use the ble.read function to read data from a characteristic on the connected device:
javascript // Device object received from onConnect callback var connectedDevice = ... // UUID of the characteristic to read var characteristicUUID = ... // Read data from the characteristic ble.read(connectedDevice.id, serviceUUID, characteristicUUID, onRead, onError);
5.2. Writing Characteristics
To send data to a characteristic on the device, you can use the ble.write function:
javascript // Device object received from onConnect callback var connectedDevice = ... // UUID of the characteristic to write to var characteristicUUID = ... // Data to send (as an ArrayBuffer) var dataToSend = ... // Write data to the characteristic ble.write(connectedDevice.id, serviceUUID, characteristicUUID, dataToSend, onWrite, onError);
5.3. Subscribing to Notifications
Some Bluetooth devices can send notifications when certain events occur. To receive notifications from a characteristic, you can use the ble.startNotification function:
javascript // Device object received from onConnect callback var connectedDevice = ... // UUID of the characteristic to subscribe to var characteristicUUID = ... // Start receiving notifications ble.startNotification(connectedDevice.id, serviceUUID, characteristicUUID, onNotification, onError); // Callback function when a notification is received function onNotification(data) { // Handle received notification console.log('Notification received:', data); }
6. Best Practices for Building Connected Apps
Building connected apps using PhoneGap and Bluetooth can be a rewarding experience, but it comes with its own set of challenges. Here are some best practices to keep in mind:
6.1. User-Friendly Device Selection
Make the device selection process user-friendly. Provide clear and concise information about the available devices and their capabilities. Consider using images or icons to identify devices.
6.2. Robust Error Handling
Implement robust error handling to gracefully handle unexpected situations, such as device disconnections or communication errors. Provide informative error messages to guide users.
6.3. Battery Efficiency
If your app uses Bluetooth Low Energy (BLE), prioritize battery efficiency. Minimize unnecessary scans and connections, and optimize data transfer to reduce power consumption.
6.4. Security
Ensure that your app follows best practices for Bluetooth security. Implement proper authentication and encryption mechanisms, especially if your app deals with sensitive data.
6.5. Thorough Testing
Thoroughly test your app on different devices and operating systems. Ensure that it functions correctly with various Bluetooth devices and scenarios.
6.6. Documentation
Provide clear and comprehensive documentation for developers who may want to integrate their hardware with your app. Explain how to use your app’s Bluetooth features and provide code examples.
Conclusion
PhoneGap and Bluetooth are a powerful combination for building connected apps that can interact with a wide range of external devices. By following the steps outlined in this guide and adhering to best practices, you can create seamless and reliable apps that take advantage of Bluetooth technology.
As you embark on your journey to build connected apps, remember that patience and perseverance are key. Bluetooth development can be challenging, but the rewards of creating innovative, connected experiences for users are well worth the effort. So, start coding, testing, and connecting with the world of Bluetooth-powered apps today!
Table of Contents
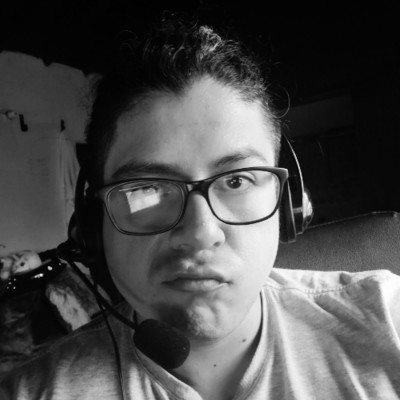
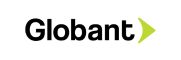