Building Offline Apps with PhoneGap: Harnessing the Power of Local Storage
In today’s fast-paced digital landscape, creating apps that work seamlessly even without a reliable internet connection has become a critical requirement. This is where the power of PhoneGap comes into play, allowing developers to build cross-platform mobile applications that can operate offline. One of the key components that facilitate this offline functionality is local storage. In this blog, we will dive deep into the world of offline apps and explore how PhoneGap, combined with local storage, can provide users with a smooth and uninterrupted experience.
Understanding the Need for Offline Apps
Mobile apps have transformed the way we live, work, and communicate. However, they often rely on an internet connection to fetch data, perform updates, or interact with remote servers. This dependence on the internet can lead to frustrating user experiences in areas with poor connectivity or during network outages. This is where offline apps shine. They enable users to access essential features and data even when they are disconnected from the web.
Introducing PhoneGap: A Cross-Platform Development Tool
PhoneGap, now known as Apache Cordova, is an open-source mobile development framework that allows developers to build apps using HTML, CSS, and JavaScript. One of the standout features of PhoneGap is its ability to package web applications as native apps for various platforms, including iOS, Android, and Windows. This flexibility makes PhoneGap an ideal choice for creating apps that function both online and offline.
Getting Started with PhoneGap
To get started, ensure you have Node.js and the PhoneGap CLI installed. If not, you can install them using the following commands:
bash npm install -g phonegap
With PhoneGap installed, you can create a new app by executing:
bash phonegap create myOfflineApp
This will generate the basic structure of your app, including necessary files and directories.
Exploring the Power of Local Storage
Local storage is a critical component when it comes to building offline apps. It allows you to store data locally on the user’s device, enabling the app to function even without an active internet connection. Local storage is essentially a key-value store where data is stored as strings. While it’s not suitable for storing large amounts of data, it’s perfect for saving essential information such as user preferences, session data, and small configuration settings.
Working with Local Storage in PhoneGap
PhoneGap provides a straightforward way to interact with local storage using the localStorage object. This object exposes methods that allow you to store, retrieve, and remove data easily. Here’s a quick example of how you can use local storage in a PhoneGap app:
javascript // Storing data in local storage localStorage.setItem('username', 'john_doe'); localStorage.setItem('theme', 'dark'); // Retrieving data from local storage const username = localStorage.getItem('username'); const theme = localStorage.getItem('theme'); console.log(`Username: ${username}, Theme: ${theme}`); // Removing data from local storage localStorage.removeItem('theme');
In this example, we store a username and a theme preference in local storage. We then retrieve and log this data to the console. Finally, we remove the theme preference from local storage.
Building Offline Capabilities with PhoneGap and Local Storage
Now that we understand the basics of PhoneGap and local storage, let’s explore how we can use these tools to build offline capabilities into our apps.
Caching Resources for Offline Access
One common approach to creating offline-capable apps is by caching essential resources. This involves storing important assets like HTML, CSS, JavaScript files, and images locally on the user’s device. This way, even if the user loses internet connectivity, they can still access and interact with the app.
PhoneGap makes it relatively easy to cache resources using the Application Cache API. This API allows you to define a cache manifest file that lists the resources you want to store locally. Here’s a simplified example:
index.html:
html <!DOCTYPE html> <html manifest="cache.manifest"> <head> <!-- Your head content here --> </head> <body> <!-- Your app content here --> </body> </html>
cache.manifest:
plaintext CACHE MANIFEST # Version 1.0 index.html styles.css app.js logo.png
In this example, the index.html file includes a reference to the cache.manifest file. The manifest file lists the resources that should be cached for offline access. Whenever these resources are accessed while the app is online, PhoneGap will store them locally. So, when the app goes offline, these cached resources will still be available.
Implementing Offline Data Synchronization
While caching resources is essential, handling data synchronization is equally crucial for offline apps. Users might perform actions or input data while offline, and it’s important to ensure that these changes are synchronized with the server once a connection is reestablished.
One approach to this is using a local database to store user-generated data. PhoneGap supports plugins like SQLite that enable you to create and manage a local database within your app. You can store data locally, perform CRUD (Create, Read, Update, Delete) operations, and then synchronize the changes with the server when an internet connection is available.
Sample Code: Using SQLite in PhoneGap for Offline Data
javascript // Install the SQLite plugin cordova plugin add cordova-sqlite-storage // Open or create a local database const db = window.sqlitePlugin.openDatabase({ name: 'myOfflineDB.db', location: 'default', }); // Execute a query to create a table db.transaction(function(tx) { tx.executeSql('CREATE TABLE IF NOT EXISTS tasks (id INTEGER PRIMARY KEY, title TEXT)'); }); // Insert data into the local database db.transaction(function(tx) { tx.executeSql('INSERT INTO tasks (title) VALUES (?)', ['Complete offline feature']); }); // Retrieve data from the local database db.transaction(function(tx) { tx.executeSql('SELECT * FROM tasks', [], function(tx, result) { const tasks = result.rows; for (let i = 0; i < tasks.length; i++) { console.log(`Task ID: ${tasks[i].id}, Title: ${tasks[i].title}`); } }); });
In this example, we use the SQLite plugin to interact with a local database. We create a table for tasks, insert a task, and then retrieve all tasks from the database.
Conclusion
In a world where connectivity isn’t always guaranteed, creating apps that work offline can greatly enhance the user experience and increase app usability. With PhoneGap and local storage, developers have powerful tools at their disposal to create such offline-capable applications. By caching resources, managing local databases, and synchronizing data with remote servers, developers can deliver seamless user experiences regardless of internet availability. As you embark on your journey to build offline apps, remember that harnessing the power of local storage is a crucial step towards ensuring your users’ satisfaction and engagement. So go ahead, start building offline-capable apps with PhoneGap, and make a lasting impact in the world of mobile development.
Table of Contents
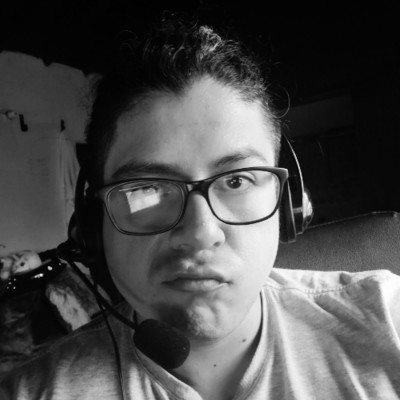
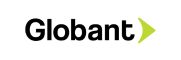