Harnessing the Camera in PhoneGap: Building Photo and Video Apps
In today’s digital age, smartphones have become an indispensable part of our lives, with built-in cameras serving as a gateway to capturing and sharing our experiences. PhoneGap, a popular open-source framework, offers developers a way to build cross-platform mobile applications using HTML, CSS, and JavaScript. One of its standout features is its ability to access the device’s camera, enabling the creation of dynamic and engaging photo and video apps. In this blog post, we’ll delve into how to harness the camera in PhoneGap and build compelling multimedia applications.
1. Understanding PhoneGap and Its Camera Capabilities:
1.1. What is PhoneGap?
PhoneGap, now known as Apache Cordova, is an open-source framework that allows developers to create mobile applications using web technologies like HTML, CSS, and JavaScript. With its native plugin architecture, PhoneGap facilitates interaction between web code and device features, such as the camera.
1.2. Camera Capabilities in PhoneGap:
PhoneGap provides a Camera plugin that grants developers access to the device’s camera hardware, enabling them to capture photos and record videos directly from their applications. This opens up a realm of possibilities for creating apps that heavily rely on multimedia content.
2. Setting Up Your PhoneGap Project:
2.1. Installation and Configuration:
To get started, you need to install Node.js, PhoneGap, and the Cordova CLI. Once set up, create a new PhoneGap project and add the Camera plugin using the command-line interface.
Code Sample:
bash # Install PhoneGap and Cordova npm install -g phonegap cordova # Create a new PhoneGap project phonegap create my-photo-app # Navigate to the project directory cd my-photo-app # Add the Camera plugin cordova plugin add cordova-plugin-camera
2.2. Permissions and Device Compatibility:
Ensure that your application’s configuration includes the necessary permissions to access the camera. Additionally, consider the varying camera capabilities across different devices and platforms. Adjust your app’s user interface and features accordingly.
3. Capturing Photos with PhoneGap:
3.1. Initializing the Camera:
To initiate the camera, first check if the plugin is ready and if the device supports camera features. Use the navigator.camera object to control camera functionality.
Code Sample:
javascript // Check if the Camera plugin is available if (navigator.camera) { // Camera-related code here } else { console.log("Camera plugin not available."); }
3.2. Handling Photo Capture:
To capture a photo, call the navigator.camera.getPicture() method. This function allows you to specify various options such as quality, source type (camera or photo library), and destination type (data URL or file path).
Code Sample:
javascript // Capture a photo from the camera navigator.camera.getPicture( function(imageData) { // Handle the captured photo var imageElement = document.getElementById("captured-photo"); imageElement.src = "data:image/jpeg;base64," + imageData; }, function(error) { console.log("Camera error: " + error); }, { quality: 80, sourceType: Camera.PictureSourceType.CAMERA, destinationType: Camera.DestinationType.DATA_URL, } );
3.3. Fine-tuning Capture Settings:
Adjust the quality and resolution of captured photos according to your app’s requirements. Explore available options like allowEdit, correctOrientation, and more to enhance user experience.
4. Recording Videos with PhoneGap:
4.1. Configuring Video Recording:
Similar to photo capture, video recording in PhoneGap involves configuring options and calling the appropriate method. The MediaCapture plugin is used for video recording.
Code Sample:
javascript // Check if the MediaCapture plugin is available if (navigator.device.capture) { // Video capture code here } else { console.log("MediaCapture plugin not available."); }
4.2. Implementing Video Capture Functionality:
Use the navigator.device.capture.captureVideo() method to initiate video recording. Specify options like duration and quality.
Code Sample:
javascript // Capture a video var options = { limit: 1, duration: 30 }; navigator.device.capture.captureVideo( function(mediaFiles) { var videoElement = document.getElementById("captured-video"); videoElement.src = mediaFiles[0].fullPath; }, function(error) { console.log("Video capture error: " + error); }, options );
4.3. Enhancing Video Recording Experience:
Consider incorporating features like video trimming, post-processing, and custom controls to enhance the user’s video recording experience.
5. Advanced Features and Tips:
5.1. Adding Filters and Effects:
Elevate your photo and video apps by integrating filters and effects. Utilize libraries like CamanJS or CSS filters to apply real-time transformations to multimedia content.
5.2. Integrating Gallery and Media Management:
Incorporate features for managing captured media, such as creating albums, categorizing content, and allowing users to edit or delete media files.
5.3. Cross-platform Considerations:
Be mindful of platform-specific differences in camera behavior, UI design, and user expectations. Test your app on different devices and operating systems to ensure a consistent experience.
6. Testing and Deployment:
6.1. Testing Your App:
Thoroughly test your app’s camera functionality on a variety of devices to identify and address potential issues. Use remote debugging tools and device emulators to streamline the testing process.
6.2. Platform-specific Considerations:
When deploying your app to different app stores, adhere to platform-specific guidelines for app submission and approval. Provide clear explanations for why your app requires camera access.
Conclusion
Harnessing the camera in PhoneGap enables developers to create captivating photo and video apps that engage users and offer unique experiences. By understanding the Camera plugin, setting up projects, implementing capture functionalities, and exploring advanced features, you can unlock the potential of multimedia content in your cross-platform mobile applications. As technology continues to evolve, mastering camera integration in PhoneGap empowers you to stay at the forefront of mobile app development and deliver impactful user experiences.
Table of Contents
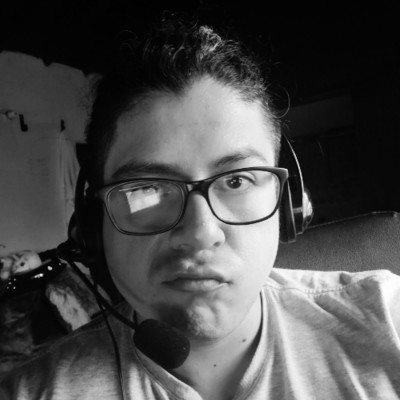
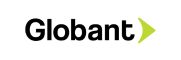