PhoneGap and Data Visualization: Creating Informative Charts
In today’s data-driven world, presenting information in a visually appealing and easily digestible manner is paramount. Data visualization plays a crucial role in conveying complex data in a comprehensible format. If you’re looking to harness the capabilities of PhoneGap for developing cross-platform mobile applications with impressive data visualization, you’re in the right place. In this blog post, we’ll walk you through the process of creating informative charts using PhoneGap.
1. Why Choose PhoneGap for Data Visualization?
Before delving into the details, it’s essential to understand why PhoneGap is an excellent choice for creating informative charts in your mobile applications.
1.1. Cross-Platform Compatibility
PhoneGap, now known as Apache Cordova, is an open-source framework that allows you to build mobile applications using web technologies such as HTML, CSS, and JavaScript. One of its standout features is its cross-platform compatibility. With PhoneGap, you can develop applications that work seamlessly on both Android and iOS devices, saving you time and effort.
1.2. Familiar Web Technologies
Developers familiar with web development will find PhoneGap intuitive to work with. You can leverage your existing knowledge of HTML, CSS, and JavaScript to create stunning data visualizations without the need to learn a new programming language or framework.
1.3. Extensive Plugin Ecosystem
PhoneGap offers a vast library of plugins that extend its functionality. This includes plugins for data visualization libraries, making it easier to integrate charts and graphs into your mobile applications.
1.4. Cost-Efficiency
By using PhoneGap, you can significantly reduce development costs since you only need to write code once to target multiple platforms. This can be a game-changer for startups and businesses looking to make the most of their resources.
2. Getting Started with PhoneGap and Data Visualization
Now that you understand why PhoneGap is an excellent choice for data visualization, let’s dive into the steps to get started with creating informative charts in your mobile application.
2.1. Setting Up Your Development Environment
Before you can start building your PhoneGap application with data visualization, you’ll need to set up your development environment. Here’s a quick guide:
2.1.1. Install Node.js
PhoneGap relies on Node.js for its development environment. You can download and install Node.js from the official website: Node.js Download.
2.1.2. Install PhoneGap CLI
Once Node.js is installed, open your command prompt or terminal and run the following command to install the PhoneGap CLI:
shell npm install -g phonegap@latest
2.1.3. Create a New PhoneGap Project
With the PhoneGap CLI installed, you can create a new project by running:
shell phonegap create mychartapp
This command will create a new PhoneGap project named “mychartapp.”
2.2. Choosing a Data Visualization Library
There are several data visualization libraries available for JavaScript, each with its unique features and capabilities. Some popular options include:
2.2.1. Chart.js
Chart.js is a simple yet flexible JavaScript charting library that allows you to create interactive and responsive charts. It supports various chart types, including bar charts, line charts, and pie charts.
2.2.2. D3.js
D3.js is a powerful library for creating data-driven documents. It provides a high level of customization and control over your visualizations but has a steeper learning curve.
2.2.3. Highcharts
Highcharts is a commercial charting library known for its ease of use and beautiful design. It offers a wide range of chart types and excellent documentation.
For this tutorial, we’ll use Chart.js for its simplicity and versatility.
2.3. Integrating Chart.js into Your PhoneGap Project
To integrate Chart.js into your PhoneGap project, follow these steps:
2.3.1. Add Chart.js to Your HTML
Download the Chart.js library from the official website and include it in your project’s HTML file:
html <script src="path/to/chart.js"></script>
2.3.2. Create a Canvas Element
Add a <canvas> element to your HTML file where you want the chart to be displayed. Give it an id so you can reference it later:
html <canvas id="myChart"></canvas>
2.3.3. Initialize the Chart
In your JavaScript file, initialize the chart by selecting the <canvas> element and configuring your chart type and data:
javascript var ctx = document.getElementById('myChart').getContext('2d'); var data = { labels: ['January', 'February', 'March', 'April', 'May'], datasets: [{ label: 'Monthly Sales', data: [50, 60, 70, 65, 80], backgroundColor: 'rgba(75, 192, 192, 0.2)', borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1 }] }; var config = { type: 'bar', data: data, options: {} }; var myChart = new Chart(ctx, config);
This code sets up a basic bar chart using Chart.js. You can customize the chart’s appearance and behavior by modifying the data and config objects.
2.4. Displaying Dynamic Data
In real-world applications, you’ll often need to display dynamic data in your charts. To do this, you can use JavaScript to fetch data from an API or a database and update your chart accordingly. Here’s a simplified example:
2.4.1. Fetch Data from an API
Suppose you have an API that provides monthly sales data. You can use the fetch API to retrieve this data and update your chart:
javascript // Fetch data from the API fetch('https://api.example.com/sales-data') .then(response => response.json()) .then(data => { // Update the chart's data myChart.data.datasets[0].data = data; myChart.update(); }) .catch(error => { console.error('Error fetching data:', error); });
This code fetches the sales data from an API, updates the chart’s data, and calls the update method to redraw the chart with the new data.
2.5. Making Your Chart Interactive
Interactive charts enhance the user experience. Chart.js provides built-in interactivity features, such as tooltips and legends. You can also add custom interactions using event listeners.
2.5.1. Tooltips
To enable tooltips that display additional information when users hover over data points, add the following options to your chart configuration:
javascript var config = { // ... options: { plugins: { tooltip: { enabled: true, mode: 'index', intersect: false } } } };
2.5.2. Legends
You can add a legend to your chart by configuring the legend option:
javascript var config = { // ... options: { plugins: { legend: { display: true, position: 'top' } } } };
2.5.3. Custom Interactions
To implement custom interactions, such as clicking on a data point to view more details, you can add event listeners to your chart:
javascript myChart.canvas.addEventListener('click', function (event) { var activePoint = myChart.getElementsAtEvent(event)[0]; if (activePoint) { var data = activePoint._chart.data; var datasetIndex = activePoint._datasetIndex; var value = data.datasets[datasetIndex].data[activePoint._index]; alert('Clicked on value: ' + value); } });
This code adds a click event listener to the chart and displays an alert with the clicked data point’s value.
Conclusion
PhoneGap, with its cross-platform compatibility and support for web technologies, is an excellent choice for creating mobile applications with informative charts and data visualizations. By integrating a library like Chart.js, you can effortlessly add interactive and dynamic charts to your apps.
As you embark on your journey to master PhoneGap and data visualization, don’t hesitate to explore other data visualization libraries, experiment with different chart types, and push the boundaries of what you can achieve in your mobile applications. With the right tools and creativity, you can transform raw data into compelling visual stories that engage and inform your users.
Start creating informative charts with PhoneGap today and elevate your mobile app development to the next level. Happy coding!
Table of Contents
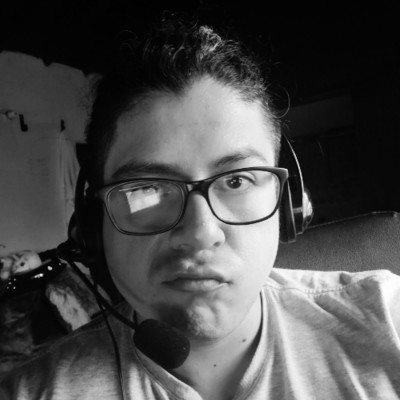
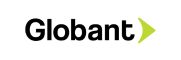