PhoneGap Debugging and Testing: Tips and Best Practices
Mobile app development can be an exciting journey, but it’s not without its challenges. One of the most critical aspects of creating a successful mobile app is debugging and testing. This becomes even more crucial when you’re using a framework like PhoneGap to develop your applications. PhoneGap allows you to build cross-platform mobile apps using web technologies like HTML, CSS, and JavaScript. While it simplifies the development process, it also presents unique challenges when it comes to debugging and testing.
In this blog post, we will explore some essential tips and best practices for debugging and testing PhoneGap applications. Whether you’re a seasoned developer or just starting with PhoneGap, these insights will help you ensure your app runs smoothly on various devices and platforms.
1. Understanding the PhoneGap Workflow
Before diving into debugging and testing, it’s essential to have a solid understanding of the PhoneGap workflow. PhoneGap (now known as Apache Cordova) enables you to write your app’s code using web technologies and then packages it as a native app for multiple platforms. This workflow involves several key components:
1.1. HTML, CSS, and JavaScript
PhoneGap apps are essentially web applications that run within a native container. You write your app’s user interface using HTML and style it with CSS. The logic and functionality are implemented using JavaScript.
1.2. Native Plugins
To access device-specific features like the camera, accelerometer, or file system, PhoneGap provides a plugin system. These plugins bridge the gap between your web code and native device functionality.
1.3. Platform-Specific Code
While PhoneGap allows you to write most of your code once and use it across platforms, you may still need to write platform-specific code for certain features or optimizations.
1.4. Build and Deployment
Once your code is ready, you use the PhoneGap CLI (Command Line Interface) or online services to build platform-specific app packages (e.g., APK for Android, IPA for iOS). These packages can then be deployed to app stores or distributed directly to users.
Understanding this workflow is crucial because it affects how you approach debugging and testing. Now, let’s delve into some tips and best practices to streamline these processes.
2. Debugging PhoneGap Applications
Debugging is the process of identifying and fixing issues in your code. When it comes to PhoneGap, you can use various tools and techniques to make this process more efficient.
2.1. Use Browser Developer Tools
Since PhoneGap apps are essentially web applications, you can take advantage of browser developer tools for debugging. Most modern browsers offer robust developer tools that allow you to inspect HTML elements, modify CSS styles, and debug JavaScript.
Here’s how you can remotely debug a PhoneGap app on an Android device using Chrome:
html <!-- Include this in your index.html file --> <script src="http://cdn.rawgit.com/remy/roid/master/roid.js"></script>
- Connect your Android device to your computer via USB.
- Open Chrome on your computer and navigate to chrome://inspect.
- Under the “Devices” section, you should see your connected Android device.
- Click “Inspect” to open the developer tools for your app running on the device.
You can set breakpoints, step through your code, and inspect network requests, making it easier to identify and fix issues.
2.2. Use Console Logging
Console logging is a simple yet effective way to debug JavaScript in PhoneGap apps. By strategically placing console.log statements in your code, you can track the flow of your application, monitor variable values, and identify errors.
Here’s an example of how to use console.log:
javascript function calculateTotal(price, quantity) { console.log('Calculating total...'); // Your calculation logic here const total = price * quantity; console.log('Total:', total); return total; }
You can view the logged messages in the browser’s developer console or in the device’s remote debugging console.
2.3. Remote Debugging with Weinre
Weinre, short for “Web Inspector Remote,” is a remote debugging tool specifically designed for PhoneGap and Cordova apps. It allows you to inspect and debug your app on remote devices, making it easier to identify issues on actual smartphones and tablets.
To use Weinre:
- Install Weinre globally using npm: npm install -g weinre
- Start Weinre: weinre –boundHost -all-
- Open your PhoneGap app on a device.
In your HTML file, include the following script:
html <script src="http://localhost:8080/target/target-script-min.js#anonymous"></script>
Open your browser and navigate to http://localhost:8080/client/#anonymous.
You’ll now be able to inspect and debug your PhoneGap app remotely using Weinre.
2.4. Check for JavaScript Errors
In addition to runtime errors, be on the lookout for JavaScript syntax errors and logic issues. Tools like JSHint and ESLint can help you catch syntax errors early in your development process. Integrating these tools into your development workflow can save you a lot of debugging time.
To use ESLint, for example, you can install it globally using npm:
bash npm install -g eslint
Then, create an ESLint configuration file and define your coding standards. Finally, run ESLint on your codebase to catch potential issues.
bash eslint your_js_file.js
2.5. Test on Multiple Devices and Platforms
Testing your PhoneGap app on a variety of devices and platforms is essential to ensure cross-compatibility. Different devices may have varying screen sizes, resolutions, and hardware capabilities, which can lead to layout and performance issues.
Consider creating a test plan that includes both Android and iOS devices with different screen sizes. Emulators and simulators are useful for initial testing, but real devices provide the most accurate results.
3. Testing PhoneGap Applications
Testing goes hand in hand with debugging, as it helps you ensure your app works correctly across different scenarios and devices. Here are some best practices for testing PhoneGap applications:
3.1. Automated Testing with Frameworks
Automated testing can significantly streamline your testing process, especially for regression testing and repetitive tasks. There are several frameworks available for testing PhoneGap apps, including:
- Appium: An open-source, cross-platform mobile application automation framework.
- Cypress: A JavaScript end-to-end testing framework for web applications, which can be adapted for PhoneGap apps.
- Calabash: An open-source acceptance testing framework for Android and iOS.
Using these frameworks, you can write test scripts that simulate user interactions and validate app behavior.
3.2. User Interface Testing
User interface (UI) testing is crucial to ensure that your app’s layout and design look and behave as expected on different devices and orientations. Consider the following tips for UI testing:
- Test your app on various screen sizes and resolutions.
- Verify that UI elements are responsive and adapt to different orientations (portrait and landscape).
- Check for consistency in font sizes, colors, and spacing.
- Ensure that touch gestures and interactions work smoothly.
3.3. Performance Testing
Performance testing is essential to identify bottlenecks and optimize your PhoneGap app for speed and responsiveness. Use tools like the Chrome Developer Tools’ Performance tab to profile your app’s performance and analyze its bottlenecks.
Here’s how you can use the Chrome Performance tab:
- Open your app on Chrome with remote debugging enabled (as mentioned earlier).
- Go to the “Performance” tab in Chrome Developer Tools.
- Click the “Record” button to start recording performance data.
- Interact with your app to simulate user interactions.
- Click the “Stop” button to end the recording.
- Analyze the performance timeline to identify issues.
3.4. Offline Testing
Many mobile apps, including PhoneGap applications, need to function offline or in low-network conditions. To ensure your app performs well in such scenarios:
- Test your app with limited or no network connectivity.
- Verify that data synchronization works correctly when the network is restored.
- Implement error-handling mechanisms for network-related issues.
3.5. Security Testing
Security is a critical aspect of mobile app development. Perform security testing to identify vulnerabilities, such as:
4. Insecure data storage.
Inadequate authentication and authorization mechanisms.
Unencrypted network communication.
Consider using automated security testing tools and conducting manual security assessments.
5. Best Practices for Cross-Platform Compatibility
PhoneGap is known for its cross-platform capabilities, allowing you to write code once and deploy it on multiple platforms. However, to ensure a seamless experience for users on different devices and operating systems, consider these best practices:
5.1. Use Responsive Design
Responsive design ensures that your app’s user interface adapts to various screen sizes and orientations. Utilize CSS frameworks like Bootstrap or media queries to create responsive layouts.
css @media (max-width: 768px) { /* CSS styles for smaller screens */ }
5.2. Test on Multiple Browsers
Although PhoneGap wraps your app in a native container, the underlying webview may differ across platforms and versions. Test your app in different mobile browsers (e.g., Chrome, Safari) to ensure compatibility.
5.3. Handle Platform-Specific Code
While PhoneGap allows you to write cross-platform code, there may still be cases where you need to implement platform-specific features or optimizations. Use conditional statements to execute platform-specific code blocks.
javascript if (device.platform === 'iOS') { // iOS-specific code } else if (device.platform === 'Android') { // Android-specific code }
5.4. Regularly Update Dependencies
Keep your PhoneGap and plugin dependencies up to date to benefit from bug fixes and performance improvements. Outdated libraries can introduce compatibility issues.
6. Continuous Integration and Deployment
To maintain the quality and reliability of your PhoneGap app, consider integrating continuous integration and deployment (CI/CD) pipelines into your development workflow. CI/CD automates the building, testing, and deployment of your app, ensuring that every code change is thoroughly tested and delivered to users efficiently.
Popular CI/CD tools for mobile app development include Jenkins, Travis CI, and CircleCI. These tools can be configured to trigger automated tests whenever code changes are pushed to your version control repository.
Conclusion
Debugging and testing PhoneGap applications is a crucial part of the mobile app development process. By following the tips and best practices outlined in this blog post, you can identify and fix issues more efficiently, ensure cross-platform compatibility, and deliver a high-quality app to your users. Remember that mobile app development is an iterative process, and continuous testing and debugging are key to maintaining a successful app in the long run.
Table of Contents
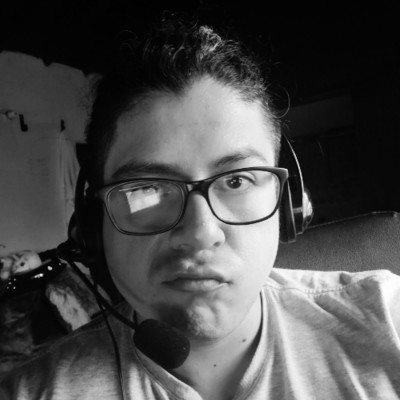
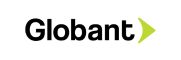