PhoneGap and Encryption: Protecting Sensitive Data in Apps
In today’s digital age, data security is paramount. Mobile applications often handle sensitive information, making them a prime target for cyberattacks. As a developer, it’s your responsibility to safeguard user data and maintain their trust. One crucial technique in achieving this is encryption. In this comprehensive guide, we’ll delve into PhoneGap and encryption, equipping you with the knowledge and tools to protect sensitive data in your apps effectively.
1. Why Encryption Matters in Mobile Apps
Before we dive into the intricacies of PhoneGap and encryption, let’s understand why encryption is crucial in mobile app development.
1.1. Protecting Sensitive Information
Mobile apps often deal with a plethora of sensitive data, including personal information, financial details, and login credentials. Without encryption, this data is vulnerable to interception and theft, leading to privacy breaches and financial losses for users.
1.2. Regulatory Compliance
Many countries have stringent data protection laws, such as the General Data Protection Regulation (GDPR) in Europe. Failure to encrypt sensitive data can result in legal consequences and fines for non-compliance.
1.3. Building User Trust
By implementing robust encryption measures, you can assure your users that their data is safe. Building trust is crucial for the success and longevity of your app.
2. PhoneGap: Bridging the Gap Between Web and Mobile
PhoneGap, now known as Apache Cordova, is a popular open-source framework for building cross-platform mobile applications using web technologies like HTML, CSS, and JavaScript. It allows developers to write code once and deploy it on multiple platforms, making it a cost-effective and efficient choice for mobile app development. However, this versatility also comes with security challenges, which encryption can help address.
2.1. Encryption Fundamentals
Before we delve into PhoneGap-specific encryption techniques, let’s review some encryption fundamentals.
2.1.1. Symmetric vs. Asymmetric Encryption
Symmetric Encryption: In this method, the same key is used for both encryption and decryption. It’s efficient for data that needs to be quickly processed but requires a secure key exchange mechanism.
Asymmetric Encryption: This approach uses a pair of keys, a public key for encryption and a private key for decryption. It’s ideal for secure communication and key exchange but is computationally intensive.
2.1.2. Encryption Algorithms
There are various encryption algorithms available, such as Advanced Encryption Standard (AES), RSA, and Elliptic Curve Cryptography (ECC). The choice of algorithm depends on factors like security requirements and performance.
2.1.3. Data Integrity
Encryption not only secures data but also ensures its integrity. Hash functions like SHA-256 can be used to verify that data has not been tampered with during transmission.
2.2. Implementing Encryption in PhoneGap
Now that we’ve covered the basics, let’s explore how to implement encryption in your PhoneGap apps.
2.2.1. Cordova Secure Storage Plugin
One of the simplest ways to secure sensitive data in PhoneGap is by using the Cordova Secure Storage plugin. This plugin provides a secure storage mechanism that encrypts data before storing it on the device. Here’s how to use it:
javascript // Install the plugin cordova plugin add cordova-plugin-secure-storage // Initialize the secure storage var secureStorage = new cordova.plugins.SecureStorage( function () { console.log("Success"); }, function (error) { console.log("Error: " + error); }, 'my_app' ); // Store sensitive data secureStorage.set( function (key) { console.log("Key stored"); }, function (error) { console.log("Error: " + error); }, 'username', 'my_secret_username' ); // Retrieve sensitive data secureStorage.get( function (value) { console.log("Value: " + value); }, function (error) { console.log("Error: " + error); }, 'username' );
This plugin encrypts data using the device’s native encryption mechanisms, providing robust security for your app’s sensitive information.
2.2.2. Web Crypto API
PhoneGap allows you to access the Web Crypto API, which provides a range of cryptographic functionalities directly within your app. This API supports both symmetric and asymmetric encryption. Here’s a basic example of using the Web Crypto API for symmetric encryption:
javascript // Generate a random encryption key window.crypto.subtle.generateKey( { name: "AES-GCM", length: 256 }, true, ["encrypt", "decrypt"] ) .then(function (key) { // Encrypt data var data = new TextEncoder().encode("Sensitive information"); window.crypto.subtle.encrypt( { name: "AES-GCM", iv: window.crypto.getRandomValues(new Uint8Array(12)) }, key, data ) .then(function (encrypted) { console.log("Encrypted data: " + new Uint8Array(encrypted)); }) .catch(function (error) { console.error("Encryption error: " + error); }); }) .catch(function (error) { console.error("Key generation error: " + error); });
This example demonstrates how to generate an encryption key and use it to encrypt sensitive data using the AES-GCM algorithm.
2.2.3. SSL/TLS for Network Communication
Encrypting data at rest is essential, but it’s equally important to secure data in transit. PhoneGap applications often communicate with remote servers, and using Secure Sockets Layer (SSL) or Transport Layer Security (TLS) protocols is crucial for securing network communication. Ensure that your app’s server uses HTTPS to encrypt data during transmission.
3. Best Practices for PhoneGap Encryption
Implementing encryption is just the beginning. To ensure maximum security for your PhoneGap app, follow these best practices:
3.1. Key Management
Secure Key Storage: Store encryption keys securely and consider using hardware-based solutions for added protection.
Key Rotation: Periodically rotate encryption keys to mitigate the risk of a compromised key.
3.2. Data Classification
Identify and classify sensitive data within your app. Not all data requires the same level of encryption, so tailor your encryption strategy accordingly.
3.3. Regular Updates
Keep your PhoneGap framework, plugins, and encryption libraries up to date to address security vulnerabilities promptly.
3.4. Penetration Testing
Conduct regular penetration testing to identify and fix security weaknesses in your app’s encryption implementation.
3.5. User Education
Educate your users about the security measures you’ve implemented to instill confidence in your app.
Conclusion
In the ever-evolving landscape of mobile app development, data security is non-negotiable. PhoneGap, with its versatility and ease of use, can be a powerful platform for building cross-platform apps. However, it also comes with security challenges that demand attention.
Encryption, as we’ve explored in this guide, is a fundamental tool for protecting sensitive data in PhoneGap applications. Whether you choose to leverage plugins like Cordova Secure Storage or harness the capabilities of the Web Crypto API, integrating encryption into your development process is essential.
By implementing encryption best practices, staying informed about emerging threats, and prioritizing data security, you can build robust and trustworthy PhoneGap apps that safeguard user information. Remember, in the world of mobile app development, encryption isn’t just a feature—it’s a responsibility.
Start strengthening the security of your PhoneGap apps today, and ensure your users’ data remains confidential and secure in an increasingly interconnected world.
Table of Contents
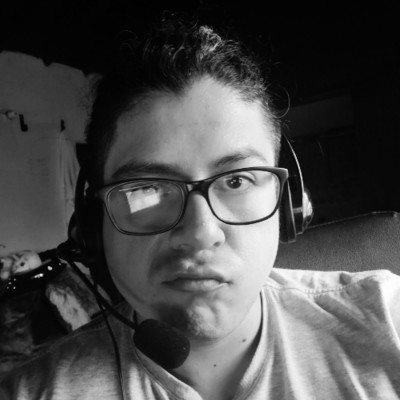
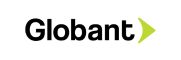