PhoneGap and Firebase Cloud Messaging: Push Notifications Made Easy
Push notifications have become an integral part of our daily digital experience. Whether it’s a new message on your favorite messaging app or a breaking news alert, push notifications keep us informed and engaged with the apps we love. If you’re developing a mobile app, integrating push notifications is a crucial step to keep your users engaged and informed.
In this blog post, we’ll explore how to implement push notifications in your PhoneGap mobile app using Firebase Cloud Messaging (FCM). PhoneGap is a popular open-source framework that allows you to build cross-platform mobile applications using web technologies such as HTML, CSS, and JavaScript. FCM, on the other hand, is a cloud-based messaging platform by Google that enables you to send notifications to iOS, Android, and web users.
Let’s dive into the world of PhoneGap and FCM and see how they make implementing push notifications a breeze.
1. Prerequisites
Before we get started, make sure you have the following prerequisites in place:
- PhoneGap Development Environment: Ensure you have PhoneGap installed and configured on your development machine. You can follow the official documentation to set up PhoneGap.
- Firebase Account: You’ll need a Firebase account to use Firebase Cloud Messaging. If you don’t have one, you can create a Firebase project for free.
2. Setting Up Firebase Cloud Messaging
Step 1: Create a Firebase Project
- Log in to your Firebase account and click on “Add Project.”
- Enter a name for your project and choose your preferred Google Analytics settings. Click “Continue.”
- Follow the on-screen instructions to complete the project creation process.
Step 2: Add an App to Your Project
- After creating the project, click on “Add app” from the project dashboard.
- Select the platform for your app (iOS, Android, or web). In our case, we’ll focus on Android and iOS.
- Follow the setup instructions, which may include adding configuration files to your app.
Step 3: Get Your FCM Credentials
- In the Firebase Console, navigate to Project Settings > General.
- Scroll down to the “Your apps” section and find the app you created earlier.
- Under the app’s name, you’ll find configuration snippets for Android and iOS. We’ll use the Android configuration for this example.
Step 4: Configure PhoneGap for FCM
- To configure your PhoneGap project to use Firebase Cloud Messaging, follow these steps:
- Install the “phonegap-plugin-push” plugin using the following command:
bash cordova plugin add phonegap-plugin-push
Next, add your FCM configuration to the PhoneGap project’s config.xml file. Replace the placeholders with your actual FCM credentials:
xml <plugin name="phonegap-plugin-push" spec="^2.3.0"> <variable name="FCM_VERSION" value="19.0.0" /> <variable name="SENDER_ID" value="YOUR_SENDER_ID" /> <variable name="ANDROID_RESOURCE_NAME" value="your_notification_icon" /> </plugin>
Make sure to replace YOUR_SENDER_ID with the actual Sender ID from your FCM project.
You can also specify your own custom notification icon by replacing “your_notification_icon” with the name of your notification icon resource.
Step 5: Initialize FCM in Your PhoneGap App
Now that PhoneGap is configured to use FCM, you need to initialize FCM in your app’s JavaScript code. You can do this in your app’s main JavaScript file. Here’s a basic example:
javascript document.addEventListener('deviceready', onDeviceReady, false); function onDeviceReady() { // Initialize FCM const push = PushNotification.init({ android: { senderID: 'YOUR_SENDER_ID', icon: 'your_notification_icon', sound: true, vibrate: true, }, ios: { senderID: 'YOUR_SENDER_ID', alert: 'true', badge: 'true', sound: 'true', }, }); // Handle incoming notifications push.on('notification', function (data) { console.log('Received Notification:', data); // Handle the notification data here }); // Handle registration push.on('registration', function (data) { console.log('Device Registered:', data.registrationId); // Send the registration ID to your server if needed }); // Handle errors push.on('error', function (e) { console.error('Error:', e.message); }); }
In this code:
- We initialize FCM using the PushNotification.init method, specifying configuration options for Android and iOS.
- We listen for incoming notifications, device registration events, and errors.
- You can customize the handling of notifications and registration based on your app’s requirements.
3. Sending Push Notifications
Now that you’ve set up push notifications in your PhoneGap app, it’s time to send notifications from your server using Firebase Cloud Messaging.
3.1. Sending a Notification from Firebase Console
- Go to the Firebase Console and select your project.
- In the left sidebar, click on “Cloud Messaging.”
- Click the “New Notification” button.
- Enter the message details, such as the notification title and body.
- You can also specify the target audience by choosing a user segment or topic.
- Click “Next” and review the notification summary.
- Finally, click “Publish” to send the notification.
3.2. Sending a Notification via Firebase Cloud Functions
If you want to send notifications programmatically, you can use Firebase Cloud Functions. Here’s a basic example of how to send a notification using Cloud Functions:
javascript const functions = require('firebase-functions'); const admin = require('firebase-admin'); admin.initializeApp(); exports.sendNotification = functions.https.onRequest((req, res) => { const message = { notification: { title: 'New Message', body: 'You have a new message from John Doe.', }, topic: 'chat', }; admin.messaging().send(message) .then(() => { res.status(200).send('Notification sent successfully'); }) .catch((error) => { console.error('Error sending notification:', error); res.status(500).send('Error sending notification'); }); });
In this example:
- We define a Firebase Cloud Function called sendNotification that sends a notification to a topic called “chat.”
- The notification message includes a title and body.
- You can trigger this function whenever a new message needs to be sent.
4. Handling Notifications
When your PhoneGap app receives a push notification, it will trigger the event handler you defined earlier. You can customize the behavior of your app in response to notifications.
For example, you might display the notification message to the user, navigate to a specific page, or update the app’s data. Here’s an example of how to handle incoming notifications and display them to the user:
javascript push.on('notification', function (data) { console.log('Received Notification:', data); // Display the notification to the user const notification = new Notification(data.title, { body: data.message, }); notification.onclick = function () { // Handle notification click event // You can navigate to a specific page or perform an action here }; });
Conclusion
Integrating push notifications into your PhoneGap app using Firebase Cloud Messaging can significantly enhance the user experience. With the power of FCM, you can engage and inform your users with timely updates and messages.
In this guide, we covered the essential steps to set up Firebase Cloud Messaging in a PhoneGap app, including configuring FCM, initializing it in your app’s JavaScript code, and sending notifications both from the Firebase Console and programmatically using Firebase Cloud Functions. We also discussed how to handle incoming notifications in your app.
By following these steps and customizing the notification handling to suit your app’s needs, you can create a seamless and engaging user experience that keeps your users informed and connected.
Push notifications are a powerful tool for mobile app developers, and with PhoneGap and Firebase Cloud Messaging, implementing them has never been easier. So go ahead, enhance your app, and keep your users engaged with timely and relevant notifications. Happy coding!
Table of Contents
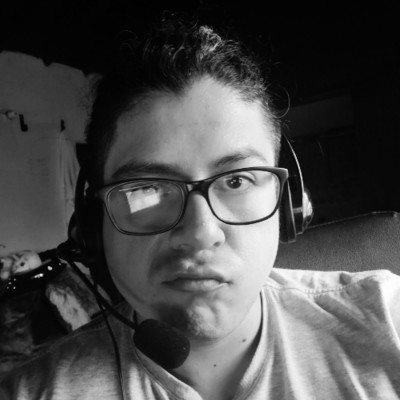
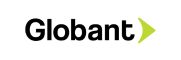