PhoneGap and OAuth Integration: Secure User Authentication
In today’s digital landscape, security is paramount, especially when it comes to user authentication in mobile applications. PhoneGap, a popular open-source framework for building cross-platform mobile apps, provides a versatile platform for creating apps that can run on various devices and platforms. To ensure the security of your users’ data, it’s essential to implement OAuth for user authentication in your PhoneGap app.
This blog post will guide you through the process of integrating OAuth for secure user authentication in your PhoneGap mobile applications. We’ll explore what OAuth is, why it’s crucial for security, and provide step-by-step instructions with code samples to help you get started.
1. Understanding OAuth
1.1. What is OAuth?
OAuth, which stands for “Open Authorization,” is a secure and standardized protocol that allows users to grant limited access to their resources (like profile information or data) to another application without sharing their credentials (e.g., username and password). OAuth is widely used for user authentication and authorization in modern web and mobile applications.
1.2. Why Use OAuth?
OAuth is a crucial component of secure user authentication for several reasons:
- Enhanced Security: OAuth eliminates the need for users to share their passwords with third-party applications, reducing the risk of unauthorized access.
- User Convenience: Users can grant and revoke access to their data without sharing their credentials, giving them more control over their information.
- Standardized Protocol: OAuth is a well-documented and widely adopted protocol, making it a trusted choice for secure authentication.
- Single Sign-On (SSO): OAuth can enable single sign-on across multiple applications, improving the user experience.
Now that we understand why OAuth is essential let’s dive into the integration process for PhoneGap.
2. PhoneGap OAuth Integration
2.1. Prerequisites
Before we start integrating OAuth into your PhoneGap app, make sure you have the following prerequisites in place:
- PhoneGap Development Environment: Ensure you have PhoneGap set up and running on your development machine. You can follow the official PhoneGap documentation for installation instructions.
- OAuth Provider: Choose an OAuth provider that you want to use for authentication. Popular choices include Google, Facebook, and GitHub. You’ll need to create an OAuth application with your chosen provider to obtain the necessary credentials.
2.2. Step-by-Step Integration
Step 1: Create a New PhoneGap Project
Begin by creating a new PhoneGap project or using an existing one. You can use the PhoneGap CLI to create a new project:
bash phonegap create my-oauth-app cd my-oauth-app
Step 2: Install OAuth Plugin
To simplify OAuth integration in your PhoneGap app, you can use an OAuth plugin. Several plugins are available for different OAuth providers. Here’s an example of how to install the cordova-plugin-googleplus plugin for Google OAuth:
bash phonegap plugin add cordova-plugin-googleplus --save --variable REVERSED_CLIENT_ID=my-reversed-client-id
Replace my-reversed-client-id with your actual reversed client ID obtained from your Google OAuth application.
Step 3: Configure OAuth Credentials
In your PhoneGap project, navigate to the configuration file (usually config.xml) and add the OAuth credentials provided by your OAuth provider. For example, for Google OAuth, you would add:
xml <plugin name="cordova-plugin-googleplus"> <variable name="REVERSED_CLIENT_ID" value="your-reversed-client-id" /> </plugin>
Step 4: Implement OAuth Authentication
Now, it’s time to implement OAuth authentication in your PhoneGap app. Create a login button or page where users can initiate the OAuth authentication flow. Here’s a simplified JavaScript example:
javascript document.getElementById('login-button').addEventListener('click', function() { window.plugins.googleplus.login( { webClientId: 'your-web-client-id', offline: true, }, function(obj) { // Authentication successful console.log('Logged in as: ' + obj.displayName); console.log('Email: ' + obj.email); console.log('ID: ' + obj.userId); // You can now use the user's data for your app }, function(msg) { // Authentication failed console.error('Login failed: ' + msg); } ); });
Replace ‘your-web-client-id’ with the actual web client ID obtained from your Google OAuth application.
Step 5: Securely Handle User Data
After successful OAuth authentication, you’ll receive user data. Handle this data securely, and consider encrypting it if necessary. You can then use this data to create user profiles, personalize experiences, or grant access to protected resources within your app.
Step 6: Implement Logout
Don’t forget to provide a logout mechanism in your app. Users should have the option to revoke access and log out from their connected OAuth account.
3. Best Practices for OAuth Integration
To ensure the highest level of security in your PhoneGap app’s OAuth integration, consider the following best practices:
3.1. Use HTTPS
Always use HTTPS to secure communication between your app and the OAuth provider’s server. This prevents man-in-the-middle attacks and ensures data integrity.
3.2. Protect Client Secrets
Keep OAuth client secrets and keys secure. Never expose them in your app’s source code or client-side JavaScript.
3.3. Implement OAuth 2.0
OAuth 2.0 is the recommended version for most applications. Ensure that your chosen OAuth provider supports OAuth 2.0.
3.4. Handle Token Expiry
OAuth tokens have a limited lifespan. Implement token refresh mechanisms to ensure seamless user experiences without requiring frequent reauthentication.
3.5. User Consent
Always seek user consent before initiating the OAuth authentication flow. Explain what data your app will access and why.
Conclusion
Integrating OAuth for secure user authentication in your PhoneGap mobile applications is a vital step in ensuring the privacy and security of your users’ data. By following the steps outlined in this guide and adhering to best practices, you can create a seamless and secure authentication experience for your users while protecting their sensitive information.
Remember that OAuth integration can vary depending on the OAuth provider you choose, so refer to the provider’s documentation for specific details. Stay updated on security best practices and regularly review your app’s authentication process to address any potential vulnerabilities.
In a world where data security and privacy are paramount, PhoneGap and OAuth integration provide a robust solution for building secure mobile apps that users can trust.
Start implementing OAuth in your PhoneGap app today, and enhance the security and user experience of your mobile application.
Happy coding!
Table of Contents
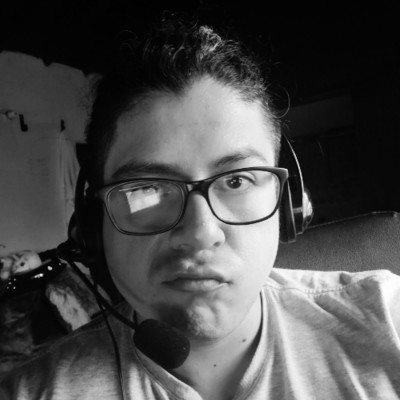
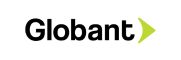