PhoneGap and Offline Maps: Navigating Without Internet Connection
In today’s fast-paced digital world, staying connected is almost second nature to us. We rely on the internet for various aspects of our lives, including navigation. However, what happens when you find yourself in a location with poor or no internet connectivity? This is where offline maps come to the rescue, and PhoneGap, a popular mobile app development framework, can help you harness their power.
1. Introduction to PhoneGap
Before delving into offline maps, let’s briefly introduce PhoneGap. PhoneGap is an open-source framework that allows developers to build cross-platform mobile applications using HTML, CSS, and JavaScript. It enables you to create mobile apps for multiple platforms, including iOS, Android, and Windows Phone, using a single codebase.
PhoneGap simplifies the app development process by providing access to device features and APIs through JavaScript, making it an excellent choice for developers who want to create mobile apps quickly and efficiently.
2. The Importance of Offline Maps
Offline maps have become indispensable for travelers, outdoor enthusiasts, and anyone who needs to navigate in areas with limited or no internet connectivity. Here are some reasons why offline maps are crucial:
2.1. No Dependency on Internet Connection
Offline maps work entirely without an internet connection. This means you can access maps and navigate even in remote areas or places with poor network coverage.
2.2. Saving Data Costs
Using online maps can consume a significant amount of mobile data, which can be costly, especially when roaming. Offline maps help you avoid these data charges by pre-downloading maps to your device.
2.3. Faster Load Times
Offline maps load quickly because they are stored locally on your device. There’s no need to wait for maps to load over a slow internet connection.
2.4. Enhanced Privacy
When you use offline maps, your location data isn’t sent to remote servers, enhancing your privacy and security.
3. Building Offline Maps with PhoneGap
Now that we understand the importance of offline maps, let’s explore how PhoneGap can be used to build offline map functionality in your mobile app. We’ll break down the process into several steps:
Step 1: Set Up Your PhoneGap Project
To get started, make sure you have PhoneGap installed on your development machine. You can install it using npm (Node Package Manager) with the following command:
bash npm install -g phonegap
Once PhoneGap is installed, create a new project using the following command:
bash phonegap create myOfflineMapApp
Replace myOfflineMapApp with your desired project name.
Step 2: Add a Map Library
To work with maps in your PhoneGap app, you’ll need a mapping library. One of the most popular choices is Leaflet, a lightweight and versatile JavaScript library for interactive maps. You can include Leaflet in your project using npm:
bash npm install leaflet
Step 3: Design the User Interface
Design the user interface of your app, including the map view. You can use HTML, CSS, and JavaScript to create a visually appealing and user-friendly map interface. Here’s a basic HTML structure for your map:
html <!DOCTYPE html> <html> <head> <title>Offline Map App</title> <!-- Include Leaflet CSS and JavaScript --> <link rel="stylesheet" href="node_modules/leaflet/dist/leaflet.css"> <script src="node_modules/leaflet/dist/leaflet.js"></script> </head> <body> <div id="map" style="height: 100vh;"></div> <!-- Add your map controls and features here --> </body> </html>
Step 4: Initialize the Map
In your JavaScript code, initialize the map using Leaflet. Specify the map’s center coordinates and zoom level. You can also add markers, layers, and other features as needed. Here’s a basic example:
javascript // Initialize the map var map = L.map('map').setView([51.505, -0.09], 13); // Add a tile layer (you can use different map providers) L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', { attribution: '© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors' }).addTo(map); // Add markers or other map features here
Step 5: Preload Map Data
To make your map available offline, you need to preload map data. You can either download map tiles for specific regions or use pre-existing offline map packages. Here’s how to preload map data:
javascript // Define a list of offline map URLs var offlineMapUrls = [ 'offline-maps/region1/{z}/{x}/{y}.png', 'offline-maps/region2/{z}/{x}/{y}.png', // Add more URLs for other regions ]; // Create an offline map layer var offlineMap = L.tileLayer.offline(offlineMapUrls, { attribution: 'Offline Map Data', minZoom: 10, // Minimum zoom level to display offline maps maxZoom: 18, // Maximum zoom level to display offline maps }); // Add the offline map layer to the map offlineMap.addTo(map);
Step 6: Implement Offline Capabilities
To enable offline functionality, you’ll need to implement features such as map caching, map updates, and handling map events when the device is offline. PhoneGap provides plugins and APIs for working with device connectivity status, which you can use to manage offline functionality.
Here’s an example of how to check the device’s online status:
javascript document.addEventListener('deviceready', function () { // Check if the device is online var isOnline = navigator.connection.type !== Connection.NONE; if (isOnline) { // Device is online, handle online behavior } else { // Device is offline, handle offline behavior } });
Step 7: Test and Deploy
Once you’ve implemented offline maps in your PhoneGap app, it’s essential to thoroughly test it on different devices and under various network conditions. Ensure that map data is preloaded correctly and that offline functionality works as expected.
After successful testing, you can deploy your app to app stores or distribute it to your target audience.
Conclusion
In a world where internet connectivity is not always guaranteed, offline maps are a valuable addition to your mobile app. PhoneGap, with its cross-platform capabilities, allows you to create powerful apps that can navigate without an internet connection. By following the steps outlined in this guide, you can integrate offline maps into your PhoneGap project and provide a seamless navigation experience for your users, regardless of their internet connectivity.
Remember that offline maps not only improve user experience but also reduce data costs and enhance privacy. So, whether you’re building a travel app, a hiking guide, or a delivery tracking system, offline maps are a feature that can make a significant difference in your app’s success.
Table of Contents
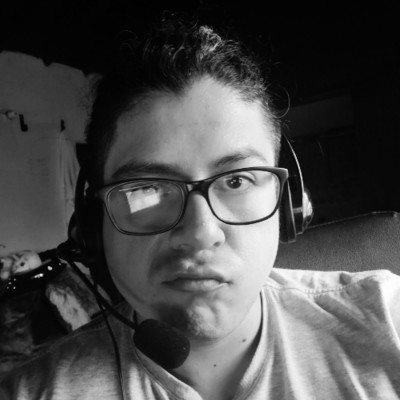
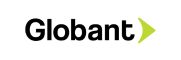