PhoneGap and Offline Sync: Building Robust Offline-First Apps
In today’s fast-paced world, users expect their mobile apps to work seamlessly, even when they’re offline. Whether it’s a travel app for navigating remote areas or a note-taking app for capturing ideas in places with spotty connectivity, the demand for offline functionality is ever-increasing. PhoneGap, a powerful framework for building cross-platform mobile applications, offers an ideal solution for creating robust offline-first apps. In this comprehensive guide, we’ll explore the concept of offline-first development and show you how to implement it effectively using PhoneGap.
1. Understanding the Offline-First Approach
1.1 What is Offline-First?
The offline-first approach is a mobile app development strategy that prioritizes seamless functionality in the absence of an internet connection. Instead of treating offline functionality as an afterthought, offline-first apps are designed to work offline by default, providing a consistent user experience regardless of connectivity status. This approach enhances reliability, user satisfaction, and the overall usability of the app.
1.2 Why Offline-First Matters
- Improved User Experience: Users can continue using the app without disruptions, fostering a positive user experience.
- Increased Accessibility: Offline-first apps can reach users in remote or low-connectivity areas where traditional online-only apps may fail.
- Data Integrity: Data is stored locally and synchronized when a connection is available, reducing the risk of data loss.
- Reduced Server Load: By offloading tasks to the client-side, the server experiences reduced traffic, improving scalability.
Now that we understand the significance of offline-first development, let’s dive into how to implement it using PhoneGap.
2. Getting Started with PhoneGap
2.1 What is PhoneGap?
PhoneGap, also known as Apache Cordova, is an open-source framework for building cross-platform mobile applications using web technologies such as HTML, CSS, and JavaScript. It enables developers to write code once and deploy it across multiple platforms, including iOS, Android, and Windows.
To start building offline-first apps with PhoneGap, follow these steps:
- Installation: Install PhoneGap on your development machine by following the installation instructions on the official PhoneGap website.
- Project Setup: Create a new PhoneGap project using the command-line tools or an integrated development environment (IDE) of your choice.
- Coding: Write your app’s frontend using HTML, CSS, and JavaScript, just like you would for a web application.
- Plugins: Utilize PhoneGap plugins to access device features and add offline functionality.
3. Implementing Offline Sync with PhoneGap
3.1 Data Synchronization Strategy
Before diving into code, it’s essential to plan your data synchronization strategy. Consider the following factors:
- Data Priority: Decide which data should be synchronized immediately and which can be synchronized in the background.
- Conflict Resolution: Determine how conflicts between locally edited data and server updates will be resolved.
- Offline Storage: Choose a suitable local storage mechanism for storing data offline, such as IndexedDB or WebSQL.
Code Example: Offline Data Storage
javascript // Initialize a local database for offline storage var db = window.openDatabase("myapp_db", "1.0", "My App Database", 200000); // Create a table for storing offline data db.transaction(function(tx) { tx.executeSql( "CREATE TABLE IF NOT EXISTS items (id unique, name)" ); });
Code Example: Data Synchronization
javascript // Check for internet connectivity function isOnline() { return navigator.onLine; } // Sync data with the server when online function syncData() { if (isOnline()) { // Fetch local data db.transaction(function(tx) { tx.executeSql("SELECT * FROM items", [], function(tx, results) { var data = []; for (var i = 0; i < results.rows.length; i++) { data.push(results.rows.item(i)); } // Send data to the server and handle synchronization logic sendDataToServer(data); }); }); } } // Trigger data synchronization when the app regains connectivity document.addEventListener("online", syncData);
In the code examples above, we initialize a local database for offline storage and create a table for storing data. The syncData function checks for internet connectivity and synchronizes data with the server when online. You can customize the synchronization logic to fit your app’s requirements.
4. Handling Offline-First UI
Creating an effective user interface for offline-first apps is crucial for providing a seamless user experience. Here are some tips for designing a user-friendly offline UI:
- Offline Indicators: Clearly communicate the app’s offline status to users. Use visual cues such as icons or messages to inform them when the app is working offline.
- Caching: Implement caching strategies to store previously loaded content. This allows users to access previously viewed data even when offline.
- Offline Forms: Enable users to interact with forms and submit data offline. Queue and submit form submissions when connectivity is restored.
Code Example: Offline Indicator
javascript // Display an offline indicator function showOfflineIndicator() { var offlineIndicator = document.getElementById("offline-indicator"); offlineIndicator.style.display = "block"; } // Hide the offline indicator when online function hideOfflineIndicator() { var offlineIndicator = document.getElementById("offline-indicator"); offlineIndicator.style.display = "none"; } // Listen for changes in connectivity document.addEventListener("offline", showOfflineIndicator); document.addEventListener("online", hideOfflineIndicator);
In the code above, we show and hide an offline indicator based on changes in connectivity status. You can customize the indicator’s appearance and behavior to match your app’s design.
5. Handling Data Synchronization Conflicts
Conflicts can arise when the same data is modified both locally and on the server. To handle conflicts gracefully, follow these steps:
- Conflict Detection: Implement a conflict detection mechanism to identify conflicting changes.
- Conflict Resolution: Define rules for resolving conflicts, such as preferring the server’s version or allowing the user to choose.
- Conflict Logging: Keep a log of conflict resolutions for auditing purposes.
Code Example: Conflict Resolution
javascript // Handle conflicts by preferring server data function resolveConflict(localData, serverData) { // Compare timestamps or other relevant criteria if (localData.timestamp > serverData.timestamp) { // Keep local data return localData; } else { // Prefer server data return serverData; } }
In the code snippet above, we resolve conflicts by comparing timestamps and favoring the data with the most recent timestamp. You can adapt this approach to your app’s specific conflict resolution strategy.
6. Testing and Debugging Offline-First Apps
Testing offline functionality can be challenging, but it’s essential to ensure your app works as expected in various scenarios. Here are some testing and debugging tips:
- Emulate Offline Mode: Use browser developer tools or emulator settings to simulate offline conditions during development.
- Logging: Implement extensive logging to track synchronization and offline-related events for debugging purposes.
- Real-World Testing: Test the app in real-world scenarios with varying levels of connectivity, including poor and intermittent connections.
7. Best Practices for Building Robust Offline-First Apps
To summarize, here are some best practices for building robust offline-first apps with PhoneGap:
- Prioritize Offline Functionality: Design your app to work offline by default, ensuring a consistent user experience.
- Plan Data Synchronization: Carefully plan your data synchronization strategy, including conflict resolution and offline storage.
- User-Friendly UI: Create an intuitive UI that communicates offline status and provides offline access to cached data.
- Conflict Resolution: Implement conflict resolution mechanisms to handle data conflicts gracefully.
- Thorough Testing: Test your app thoroughly in various offline and online scenarios to identify and address issues.
By following these best practices and leveraging the power of PhoneGap, you can build reliable and user-friendly offline-first apps that meet the demands of today’s mobile users.
Conclusion
The offline-first approach is becoming increasingly important as users expect mobile apps to work seamlessly regardless of connectivity. PhoneGap offers a powerful platform for building robust offline-first apps, enabling developers to create reliable and user-friendly experiences. By understanding the principles of offline-first development, implementing data synchronization strategies, and following best practices, you can harness the full potential of PhoneGap to deliver exceptional offline-first mobile applications. Start building your offline-first app today and empower your users with uninterrupted access to their favorite content and features.
Table of Contents
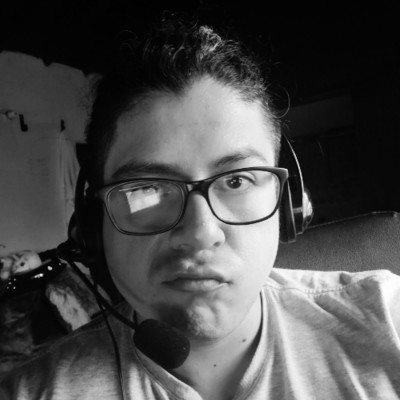
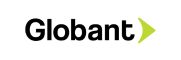