PhoneGap Performance Optimization: Tips for Faster Hybrid Apps
In the world of mobile app development, performance is paramount. Users expect snappy, responsive apps that deliver a seamless experience. However, when building hybrid apps with frameworks like PhoneGap (now known as Apache Cordova), achieving optimal performance can be a challenge due to the bridge between web and native code. Fear not, though! In this blog, we’ll explore essential tips and techniques to supercharge your PhoneGap apps for lightning-fast performance.
1. Profile Your App
Before diving into optimizations, it’s crucial to understand where your app is struggling. Profiling your app helps identify bottlenecks and performance hotspots. PhoneGap provides tools like GapDebug and Weinre that allow you to inspect and profile your app on real devices or emulators. These tools help you spot memory leaks, slow JavaScript functions, and other issues.
Code Sample:
javascript // Sample code to start profiling in PhoneGap if (window.cordova && window.cordova.plugins && window.cordova.plugins.GapDebug) { cordova.plugins.GapDebug.startProfiling(); }
2. Optimize JavaScript Code
JavaScript performance is a cornerstone of PhoneGap app performance. Follow these best practices to optimize your code:
2.1. Minify and Bundle
Minifying your JavaScript code reduces its size by removing unnecessary whitespace and renaming variables. Use tools like UglifyJS or Terser to minify your code. Additionally, bundle your JavaScript files to reduce HTTP requests and load times.
Code Sample:
bash # Minify JavaScript using UglifyJS uglifyjs your_script.js -o your_script.min.js
2.2. Lazy Load Resources
Load resources like images, scripts, and stylesheets lazily to speed up the initial page load. Consider using the defer and async attributes for script tags to control when they are executed.
Code Sample:
html <script src="your_script.js" defer></script>
2.3. Optimize Loops
Avoid heavy computations within loops. Opt for efficient algorithms and data structures, and consider using Web Workers to offload CPU-intensive tasks to separate threads.
Code Sample:
javascript // Inefficient loop for (let i = 0; i < array.length; i++) { // Heavy computation } // Optimized loop const length = array.length; for (let i = 0; i < length; i++) { // Efficient computation }
3. Leverage Hardware Acceleration
PhoneGap allows you to access native device features through JavaScript plugins. However, not all plugins are created equal in terms of performance. When selecting plugins, prioritize those that utilize hardware acceleration and native code to maximize performance.
3.1. Use Crosswalk Webview
Crosswalk is a WebView replacement that provides consistent performance across Android devices. It embeds the Chromium engine, ensuring your app runs smoothly on older Android versions that might have slower default WebViews.
Code Sample:
xml <!-- Specify Crosswalk Webview in your Android configuration --> <preference name="xwalkVersion" value="xwalk_core_library_version" />
3.2. Choose Lightweight Plugins
Opt for lightweight plugins that have minimal impact on your app’s performance. Review the documentation and community feedback to gauge a plugin’s impact on performance.
4. Implement Caching Strategies
Caching can significantly improve the speed and responsiveness of your PhoneGap app. By reducing server requests and local data fetching, you can enhance the user experience.
4.1. Local Storage
Use the localStorage API to store small amounts of data locally, such as user preferences and app settings. This reduces the need to fetch data from the network repeatedly.
Code Sample:
javascript // Store data in local storage localStorage.setItem('key', 'value'); // Retrieve data from local storage const data = localStorage.getItem('key');
4.2. App Cache
Implement the App Cache (now deprecated in favor of Service Workers) to store static assets, enabling your app to work offline and load resources faster.
Code Sample:
html <!-- Include the App Cache manifest in your HTML file --> <html manifest="appcache.manifest">
4.3. Service Workers
Utilize Service Workers to manage caching and improve the offline experience. Service Workers allow you to cache dynamic content and update it in the background.
Code Sample:
javascript // Register a service worker in your JavaScript if ('serviceWorker' in navigator) { navigator.serviceWorker.register('service-worker.js') .then(registration => { // Service Worker registered successfully }) .catch(error => { // Registration failed }); }
5. Optimize UI/UX
A responsive and visually appealing user interface contributes to the perception of app performance. Keep these UI/UX optimization tips in mind:
5.1. Reduce DOM Manipulation
Excessive DOM manipulation can slow down your app. Use libraries like jQuery wisely and minimize unnecessary updates to the DOM.
Code Sample:
javascript // Inefficient DOM manipulation $('#element').addClass('active'); // Optimized DOM manipulation const element = document.getElementById('element'); element.classList.add('active');
5.2. Implement Smooth Transitions
Smooth animations and transitions create a polished user experience. Utilize CSS transitions and animations for visual effects.
Code Sample:
css /* CSS for smooth transition */ .element { transition: transform 0.3s ease-in-out; } /* JavaScript to trigger transition */ const element = document.querySelector('.element'); element.style.transform = 'translateX(100px)';
5.3. Lazy Load Images
Load images as they come into the viewport to reduce initial page load times. Implement techniques like the loading=”lazy” attribute for images.
Code Sample:
html <img src="image.jpg" alt="Lazy-loaded image" loading="lazy">
6. Test on Real Devices
Testing your PhoneGap app on real devices is crucial to uncover performance issues that might not surface in emulators or simulators. Different devices and operating systems can behave differently, so be sure to test across a variety of hardware.
7. Update PhoneGap and Plugins
Frequent updates to PhoneGap and its plugins often include performance improvements and bug fixes. Keeping your development environment up to date ensures you’re benefiting from the latest enhancements.
Conclusion
Performance optimization is an ongoing process in app development, especially when building hybrid apps with PhoneGap. By profiling your app, optimizing JavaScript code, leveraging hardware acceleration, implementing caching strategies, and fine-tuning your UI/UX, you can create a seamless and responsive user experience. Remember that testing and staying up to date with the latest tools and updates are essential for maintaining peak performance in your PhoneGap apps. With these tips and techniques in your toolkit, you’re well-equipped to create faster and more efficient hybrid apps that users will love.
Table of Contents
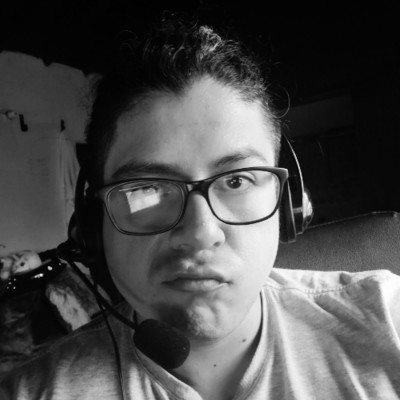
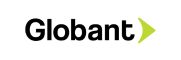