PhoneGap and RESTful APIs: Building Data-Driven Mobile Apps
Mobile applications have become an integral part of our daily lives, and their demand continues to soar. Whether it’s for business, entertainment, or personal productivity, people rely on mobile apps for various tasks. To meet this ever-growing demand, developers need efficient tools and technologies to build robust mobile applications quickly. PhoneGap and RESTful APIs are two such tools that, when combined, empower developers to create data-driven mobile apps with ease. In this guide, we’ll explore the synergy between PhoneGap and RESTful APIs, providing you with the knowledge and code samples you need to get started.
1. Understanding PhoneGap
1.1 What is PhoneGap?
PhoneGap, also known as Apache Cordova, is an open-source mobile app development framework that allows developers to build cross-platform mobile applications using web technologies such as HTML, CSS, and JavaScript. This means you can write your app once and deploy it on multiple platforms, including iOS, Android, and Windows.
1.2 Key Features of PhoneGap
1. Cross-Platform Compatibility
PhoneGap eliminates the need to write separate codebases for different platforms. Developers can create a single codebase that works seamlessly on various devices and operating systems.
2. Access to Native Device Features
PhoneGap provides plugins that allow developers to access native device features like the camera, GPS, and accelerometer, enhancing the functionality and user experience of their apps.
3. Large Community and Plugin Ecosystem
The PhoneGap community is vast, and there are numerous plugins available, simplifying the integration of various functionalities into your app.
4. Web-Based Development
Developers can leverage their web development skills to create mobile apps, making it easier for web developers to transition into mobile app development.
2. Leveraging RESTful APIs
2.1 What are RESTful APIs?
RESTful (Representational State Transfer) APIs are a set of architectural constraints that guide the design of networked applications. They use HTTP requests to perform CRUD (Create, Read, Update, Delete) operations on resources, making them ideal for building web services and mobile app backends.
2.2 Key Features of RESTful APIs
1. Stateless Communication
RESTful APIs are stateless, meaning each request from a client to the server must contain all the information needed to understand and process the request. This simplicity makes them highly scalable and easy to maintain.
2. Standardized Communication
HTTP methods like GET, POST, PUT, and DELETE are used to interact with resources, making RESTful APIs straightforward and easy to work with.
3. Scalability and Performance
RESTful APIs can handle a large number of clients and are designed to be efficient, making them suitable for serving data to mobile apps.
4. Flexibility
Developers have the flexibility to structure their APIs to fit their application’s needs, allowing for efficient data retrieval and manipulation.
3. Integrating PhoneGap and RESTful APIs
Now that we understand the basics of PhoneGap and RESTful APIs, let’s explore how they can work together to build data-driven mobile apps. To illustrate this, we’ll walk through a simple example of creating a mobile app that fetches and displays data from a RESTful API.
3.1 Prerequisites
Before we dive into coding, ensure you have the following:
- Node.js and npm installed on your system.
- PhoneGap CLI installed globally.
- A RESTful API with endpoints for retrieving data.
Step 1: Create a PhoneGap Project
First, create a new PhoneGap project by running the following command:
bash phonegap create my-data-driven-app
This command will generate a new PhoneGap project folder named “my-data-driven-app.”
Step 2: Add Platforms
Navigate to your project folder:
bash cd my-data-driven-app
Next, add the platforms you want to target. For example, to add Android and iOS platforms:
bash phonegap platform add android ios
Step 3: Install Required Plugins
To interact with RESTful APIs and access device features, you’ll need to install some plugins. Let’s start with the Network Information and Whitelist plugins:
bash phonegap plugin add cordova-plugin-network-information cordova-plugin-whitelist
Step 4: Create the User Interface
In your project folder, locate the “www” directory, and create an HTML file (e.g., index.html) for your app’s user interface. Here’s a simple example:
html <!DOCTYPE html> <html> <head> <title>Data-Driven Mobile App</title> </head> <body> <h1>Data-Driven Mobile App</h1> <ul id="data-list"></ul> <script src="js/app.js"></script> </body> </html>
Step 5: Fetch Data from the RESTful API
Create a JavaScript file (e.g., app.js) in the “www/js” directory to fetch and display data from the RESTful API:
javascript document.addEventListener('deviceready', onDeviceReady, false); function onDeviceReady() { // Detect network connection var networkState = navigator.connection.type; if (networkState === Connection.NONE) { alert('No network connection. Please check your internet connection.'); } else { fetchData(); } } function fetchData() { var apiUrl = 'https://api.example.com/data'; // Replace with your API endpoint var dataList = document.getElementById('data-list'); fetch(apiUrl) .then(function (response) { return response.json(); }) .then(function (data) { // Process and display data data.forEach(function (item) { var listItem = document.createElement('li'); listItem.textContent = item.name; dataList.appendChild(listItem); }); }) .catch(function (error) { console.error('Error fetching data:', error); }); }
Step 6: Configure the Content Security Policy
In your project’s “config.xml” file, make sure you configure the Content Security Policy to allow communication with your API. Add the following lines inside the <widget> element:
xml <access origin="*" /> <allow-intent href="http://*/*" /> <allow-intent href="https://*/*" /> <allow-navigation href="http://*/*" /> <allow-navigation href="https://*/*" />
Step 7: Build and Run Your App
You can now build your app for your target platforms:
bash phonegap build android phonegap build ios
Once the build process is complete, you can run your app on an emulator or a physical device:
bash phonegap run android phonegap run ios
Your app will fetch data from the RESTful API and display it on the screen. This is a basic example, but it demonstrates the fundamental concepts of integrating PhoneGap and RESTful APIs.
4. Best Practices for Building Data-Driven Mobile Apps
While the above example gives you a glimpse of how PhoneGap and RESTful APIs can work together, building a robust data-driven mobile app requires adhering to best practices. Here are some tips to keep in mind:
4.1. Authentication and Security
Ensure that your RESTful API is secure and uses proper authentication mechanisms, such as OAuth or API keys. Protect sensitive data and user information by implementing encryption and secure communication protocols.
4.2. Error Handling
Handle errors gracefully in your mobile app. Provide meaningful error messages to users when something goes wrong and implement proper error logging on the server side to troubleshoot issues.
4.3. Offline Support
Consider implementing offline support for your app. Use local storage to cache data and allow users to interact with the app even when they have no internet connection. Sync data with the server when a connection is available.
4.4. Performance Optimization
Optimize your app’s performance by minimizing network requests, compressing data, and lazy-loading resources. Use pagination and limit the amount of data retrieved from the API to reduce load times.
4.5. User Experience
Focus on providing an exceptional user experience. Ensure that your app is responsive, intuitive, and visually appealing. Test your app on different devices and screen sizes to ensure compatibility.
Conclusion
PhoneGap and RESTful APIs are powerful tools for building data-driven mobile apps. By combining the cross-platform capabilities of PhoneGap with the versatility of RESTful APIs, developers can create mobile applications that fetch and display data from remote servers, opening up endless possibilities for innovation.
In this guide, we’ve explored the basics of PhoneGap and RESTful APIs, walked through a step-by-step example, and discussed best practices for building data-driven mobile apps. Armed with this knowledge and the provided code samples, you’re well-equipped to embark on your journey to create feature-rich and dynamic mobile applications that connect to remote data sources. So, go ahead and start turning your app ideas into reality with PhoneGap and RESTful APIs. Happy coding!
Table of Contents
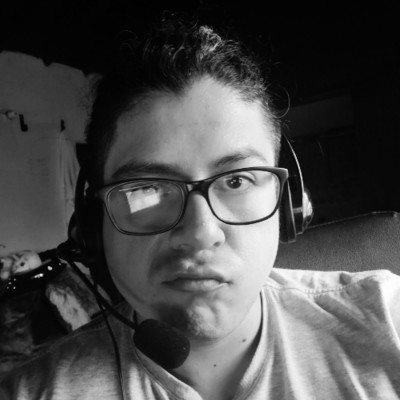
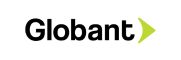