PhoneGap Security Best Practices: Keeping User Data Safe
In today’s digital age, mobile applications have become an integral part of our lives. They help us shop, communicate, navigate, and manage our daily tasks. However, with this increased reliance on mobile apps, the security of user data has become a paramount concern. Developers must ensure that the sensitive information entrusted to their applications remains safe and protected.
PhoneGap, a popular open-source mobile development framework, allows developers to build cross-platform mobile applications using web technologies like HTML, CSS, and JavaScript. While it simplifies the development process, it’s crucial to understand and implement security best practices to keep user data safe.
In this blog, we will explore PhoneGap security best practices and provide you with actionable insights to protect user data effectively. Let’s dive in!
1. Why PhoneGap Security Matters
1.1 Understanding the Risks
Security in mobile applications is not just a feature; it’s a necessity. Failing to secure user data can lead to severe consequences, including legal issues, reputation damage, and financial losses. Some of the common risks associated with mobile app security include:
- Data Breaches: Unauthorized access to sensitive user information, such as personal details, payment data, or login credentials, can result in data breaches. This can lead to identity theft and financial fraud.
- Malware and Malicious Code: Mobile apps can become vectors for malware and other malicious code if not adequately protected. Malware can steal data, manipulate the app’s behavior, or compromise the device itself.
- Privacy Violations: Apps that collect and transmit user data without proper consent or encryption may violate user privacy rights, leading to legal consequences and reputational damage.
1.2 Consequences of Data Breaches
Data breaches can have far-reaching consequences for both developers and users. For developers, the fallout from a breach can include:
- Loss of Trust: Users may lose trust in your app and company, leading to a decrease in user base and revenue.
- Legal Consequences: Depending on the nature of the breach, you may face legal action, fines, or penalties for not protecting user data adequately.
- Reputation Damage: News of a data breach can spread quickly, damaging your brand’s reputation.
For users, the consequences can be even more severe:
- Identity Theft: Stolen personal information can be used for identity theft, leading to financial losses and emotional distress.
- Financial Losses: If payment data is compromised, users may experience unauthorized transactions and financial losses.
- Privacy Invasion: Users expect their data to be treated with respect and kept private. A breach can be a significant invasion of privacy.
Given these risks, it’s clear that phoneGap security should be a top priority for developers. Now, let’s delve into the best practices to safeguard user data effectively.
2. Authentication and Authorization
Authentication and authorization are the first lines of defense when it comes to securing user data. Here are some essential practices to implement:
2.1 Implementing Secure Authentication
javascript // Sample code for secure authentication function authenticateUser(username, password) { // Validate user credentials if (isValidCredentials(username, password)) { // Generate a secure authentication token const authToken = generateAuthToken(); // Store the token securely secureStorage.set('authToken', authToken); return true; } else { return false; } }
- Strong Password Policies: Enforce strong password policies, including length and complexity requirements, to protect user accounts from brute-force attacks.
- Token-Based Authentication: Implement token-based authentication to secure API requests. Tokens should have short lifetimes and be securely stored.
2.2 Role-Based Access Control
javascript // Sample code for role-based access control function hasPermission(user, resource, action) { // Check user's role and permissions const userRole = getUserRole(user); const resourcePermissions = getResourcePermissions(resource); return resourcePermissions.includes(action) && userRole === 'admin'; }
- Role-Based Access Control (RBAC): Assign roles and permissions to users to ensure they can only access the data and features they need. Restrict access to sensitive operations.
- Least Privilege Principle: Follow the principle of least privilege, ensuring that users have only the permissions necessary for their tasks.
3. Data Encryption
Data encryption is a critical aspect of mobile app security. It involves protecting data both in transit and at rest.
3.1 Securing Data in Transit
javascript // Sample code for securing data in transit using HTTPS const secureConnection = new SecureConnection('https://api.example.com'); secureConnection.sendData(encryptedData);
- Use HTTPS: Always use HTTPS to encrypt data transmitted between the app and the server. Avoid sending sensitive information over unencrypted connections.
- Transport Layer Security (TLS): Implement the latest TLS protocols to ensure secure communication between the app and the server.
3.2 Data at Rest Encryption
javascript // Sample code for encrypting data at rest in local storage const encryptedData = encryptData(userSensitiveData); localStorage.setItem('userData', encryptedData);
- Local Data Encryption: Encrypt sensitive data stored on the device, such as in local storage or databases. Use strong encryption algorithms and secure key management.
- Data Purging: Implement mechanisms to securely delete data that is no longer needed to minimize the risk of data exposure.
4. Secure Communication
Secure communication is essential to prevent man-in-the-middle attacks and other interception techniques.
4.1 Using HTTPS
javascript // Sample code for enforcing HTTPS connections in PhoneGap if (location.protocol !== 'https:') { location.replace(`https:${location.href.substring(location.protocol.length)}`); }
- Enforce HTTPS: Ensure that your app only communicates with servers over HTTPS. Implement mechanisms to redirect HTTP requests to HTTPS.
- Certificate Validation: Perform certificate validation to verify the authenticity of the server. Avoid disabling certificate validation for convenience.
4.2 Avoiding Mixed Content
html <!-- Sample HTML to avoid mixed content --> <script src="https://secure.example.com/script.js"></script>
- Avoid Mixed Content: Do not load non-HTTPS resources within an HTTPS page. Mixed content can introduce security vulnerabilities.
- Content Security Policy (CSP): Implement CSP headers to define which sources of content are considered valid for your app. This helps prevent content injection attacks.
5. Input Validation
Input validation is crucial to prevent common security vulnerabilities such as SQL injection and Cross-Site Scripting (XSS).
5.1 Preventing Injection Attacks
javascript // Sample code for preventing SQL injection function queryDatabase(username) { const sanitizedUsername = sanitizeInput(username); const query = `SELECT * FROM users WHERE username = '${sanitizedUsername}'`; // Execute the query securely }
- Sanitize Input: Always validate and sanitize user inputs before processing them, especially when dealing with databases. Use parameterized queries to prevent SQL injection.
5.2 Validating User Input
javascript // Sample code for validating user input function validateEmail(email) { const emailRegex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,4}$/; return emailRegex.test(email); }
- Client-Side Validation: Implement client-side validation to check user inputs for correctness and completeness before submitting data to the server.
- Server-Side Validation: Additionally, perform server-side validation to ensure data integrity and security. Never rely solely on client-side validation.
6. Secure Storage
Securely storing data on the device is crucial to protect user information from unauthorized access.
6.1 Protecting Local Storage
javascript // Sample code for protecting data in local storage localStorage.setItem('userToken', encryptData(authToken));
- Encryption: Encrypt sensitive data stored in local storage to prevent unauthorized access even if the device is compromised.
- Clear Text Avoidance: Avoid storing plaintext passwords or sensitive information in local storage. Use secure tokens or encrypted data instead.
6.2 Leveraging Encrypted Databases
javascript // Sample code for using encrypted databases in PhoneGap const db = window.openDatabase('mydb', '1.0', 'My Database', 2 * 1024 * 1024); db.transaction(function (tx) { tx.executeSql('CREATE TABLE IF NOT EXISTS users (id unique, name, password)'); tx.executeSql('INSERT INTO users (name, password) VALUES (?, ?)', [name, password]); });
- SQLite with Encryption: If your app uses a local database (e.g., SQLite), consider using encrypted databases to protect sensitive data stored on the device.
- Key Management: Implement robust key management practices to safeguard encryption keys. Keys should not be hard-coded within the app.
7. Code Obfuscation
Code obfuscation helps make it more challenging for attackers to reverse engineer your app.
7.1 Obfuscating JavaScript Code
javascript // Sample code obfuscation techniques function obfuscateCode() { // Implement code obfuscation here }
- Minification and Mangling: Minify and mangle your JavaScript code to reduce its readability. Tools like UglifyJS can help with this.
- Code Splitting: Split your code into smaller modules to make it harder for attackers to understand the entire codebase.
7.2 Minimizing Code Exposure
javascript // Sample code for encapsulating sensitive functionality (function () { // Encapsulated sensitive functionality })();
- Encapsulation: Encapsulate sensitive functionality within closures or modules to limit its exposure and access.
- Access Control: Control access to critical parts of your app by implementing appropriate access controls and permissions.
8. Regular Updates and Patching
Staying up-to-date with dependencies and addressing vulnerabilities is vital for app security.
8.1 Staying Current with Dependencies
javascript // Sample code to check for and update dependencies npm outdated npm update
- Dependency Monitoring: Regularly check for updates to your app’s dependencies, including PhoneGap and any third-party libraries. Vulnerabilities can be discovered and patched over time.
- Automated Scanning: Use automated scanning tools to identify vulnerable dependencies and receive notifications when updates are available.
8.2 Addressing Vulnerabilities
javascript // Sample code to address vulnerabilities in PhoneGap plugins cordova plugin update <plugin-name>
- Timely Patching: When a security vulnerability is identified in a dependency or PhoneGap plugin, take immediate action to update or patch it.
- Security Notifications: Subscribe to security mailing lists and notifications from relevant authorities to stay informed about emerging threats and vulnerabilities.
9. User Education and Awareness
Don’t underestimate the importance of educating your users about security best practices.
9.1 Promoting Strong Passwords
html <!-- Sample password strength meter in registration form --> <input type="password" id="password" onkeyup="checkPasswordStrength(this.value)"> <span id="password-strength"></span>
- Password Strength Meter: Implement a password strength meter to guide users in creating strong passwords.
- Password Policies: Educate users about the importance of using unique passwords for different services and regularly updating them.
9.2 Educating Users on Security
javascript // Sample code for in-app security tips function showSecurityTips() { const securityTips = [ "Never share your password with anyone.", "Enable two-factor authentication for added security.", "Regularly log out of your account on shared devices.", ]; // Display security tips to users }
- In-App Tips: Provide security tips within your app to educate users about best practices for protecting their accounts and data.
- FAQ and Help Center: Maintain a comprehensive FAQ or help center within your app to address common security-related queries and concerns.
Conclusion
PhoneGap is a powerful tool for developing cross-platform mobile applications, but it’s only as secure as the practices you implement. Protecting user data is not optional; it’s a responsibility. By following these PhoneGap security best practices, you can significantly reduce the risks of data breaches, privacy violations, and other security threats in your mobile apps.
Remember that security is an ongoing process. Stay vigilant, keep your app and dependencies up-to-date, and remain informed about emerging threats in the mobile app landscape. By doing so, you can create a safer and more trustworthy mobile experience for your users.
Table of Contents
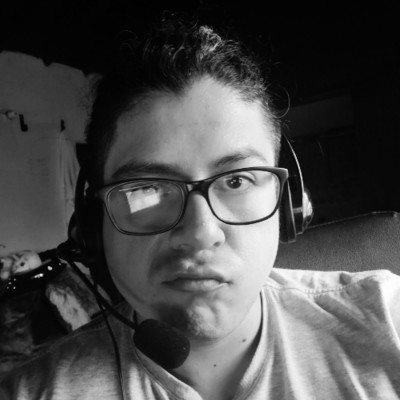
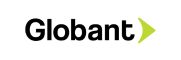