PhoneGap and User Notifications: Keeping Users Informed
In the ever-evolving landscape of mobile app development, keeping users informed and engaged is paramount. Imagine you’ve built a fantastic app using PhoneGap, a versatile framework for cross-platform mobile app development. You’ve nailed the design, functionalities, and user experience, but there’s one crucial element left: keeping your users in the loop.
User notifications are a powerful tool for enhancing user engagement and retention. They provide a way to communicate with users, deliver important updates, and keep them coming back to your app. In this comprehensive guide, we’ll explore how to implement user notifications in your PhoneGap app, covering various notification types and providing code samples for each.
1. Understanding User Notifications
Before diving into the technical aspects, let’s get a clear understanding of what user notifications are and why they matter in mobile app development.
1.1. What Are User Notifications?
User notifications are messages or alerts sent by an app to inform users about specific events, updates, or interactions. They can appear as banners, alerts, badges, or sounds on the user’s device. Notifications serve several purposes, such as:
- Information Delivery: Notify users about new messages, updates, or relevant content.
- User Engagement: Encourage users to interact with your app by reminding them of its presence.
- Retention: Keep users coming back to your app by providing valuable and timely information.
- Why Are User Notifications Important?
User notifications play a pivotal role in user engagement and app retention. Here’s why they are essential:
- Improved User Experience: Notifications provide real-time information and enhance the overall user experience.
- Increased User Retention: Regular notifications can remind users to revisit your app, leading to higher retention rates.
- Enhanced Communication: Notifications enable direct communication with users, fostering a stronger connection.
- Customization: You can tailor notifications to match user preferences, creating a personalized experience.
Now that we understand the significance of user notifications, let’s explore how to implement them effectively in your PhoneGap app.
2. Types of User Notifications
Before we delve into coding, it’s crucial to know the types of user notifications you can use in your PhoneGap app. Each type serves a specific purpose, so choose the one that best suits your app’s requirements.
2.1. Local Notifications
Local notifications are generated and triggered by your app itself. They are ideal for reminding users about events, tasks, or updates related to your app, even when the app is not actively running.
Code Sample:
javascript // Schedule a local notification cordova.plugins.notification.local.schedule({ id: 1, title: "Don't forget!", text: "You have an important meeting at 3 PM.", sound: null, data: { meetingId: 123 } });
2.2. Push Notifications
Push notifications are sent from a remote server to your app, notifying users about updates or new content. They are a powerful tool for re-engaging users and delivering real-time information.
Code Sample:
javascript // Configure push notification settings const push = PushNotification.init({ android: {}, ios: { alert: true, badge: true, sound: true } }); // Receive and handle push notifications push.on('notification', (data) => { console.log('Received Push Notification:', data); });
2.3. In-App Notifications
In-app notifications are messages displayed within your app’s interface. They are useful for guiding users through your app or providing context-specific information.
Code Sample:
javascript // Show an in-app notification cordova.plugins.inAppBrowser.open('https://example.com', '_blank', 'location=yes');
2.4. Badges
Badges are small numeric indicators that appear on your app’s icon, usually displaying the count of unread messages or notifications. They provide a visual cue to users.
Code Sample:
javascript // Set a badge count cordova.plugins.notification.badge.set(3);
Now that you have an overview of the types of user notifications available, let’s explore how to implement them in your PhoneGap app.
3. Implementing User Notifications in PhoneGap
Implementing user notifications in PhoneGap is relatively straightforward, thanks to plugins that simplify the process. Below, we’ll guide you through the steps to set up and use notifications in your PhoneGap app.
3.1. Setting Up Your PhoneGap Project
If you haven’t already, create a PhoneGap project using the CLI. Make sure you have Node.js and npm installed on your system.
shell npm install -g phonegap phonegap create my-app cd my-app
3.2. Installing Notification Plugins
To enable notifications, you’ll need to install appropriate plugins for your chosen notification types (local, push, in-app, badges). Use the Cordova CLI to install these plugins:
shell cordova plugin add cordova-plugin-local-notification cordova plugin add phonegap-plugin-push cordova plugin add cordova-plugin-inappbrowser cordova plugin add cordova-plugin-badge
3.3. Configuring Push Notifications
For push notifications, you’ll need to configure your app with the necessary credentials and permissions. Follow the documentation provided by your chosen push notification service (e.g., Firebase Cloud Messaging for Android or Apple Push Notification Service for iOS).
3.4. Implementing Notifications
Now, let’s implement notifications in your app. Below are code samples for each notification type:
3.4.1. Local Notifications
javascript cordova.plugins.notification.local.schedule({ id: 1, title: "Don't forget!", text: "You have an important meeting at 3 PM.", sound: null, data: { meetingId: 123 } });
3.4.2. Push Notifications
javascript const push = PushNotification.init({ android: {}, ios: { alert: true, badge: true, sound: true } }); push.on('notification', (data) => { console.log('Received Push Notification:', data); });
3.4.3. In-App Notifications
javascript cordova.plugins.inAppBrowser.open('https://example.com', '_blank', 'location=yes');
3.4.4. Badges
javascript cordova.plugins.notification.badge.set(3);
3.5. Handling User Interactions
It’s essential to handle user interactions with notifications. For push notifications, you can define actions when a user taps on a notification. For in-app notifications, ensure users can dismiss or interact with the message as needed.
3.6. Testing Notifications
Test your notifications thoroughly on both Android and iOS devices. Verify that notifications are displayed correctly, and user interactions are handled appropriately.
4. Best Practices for User Notifications
To ensure your user notifications are effective and well-received, consider the following best practices:
- Relevance: Send notifications that are timely and relevant to the user’s context or behavior.
- Frequency: Avoid overwhelming users with too many notifications. Be selective and send notifications sparingly.
- Personalization: Tailor notifications based on user preferences and behavior to make them feel more personal.
- Clear Messaging: Ensure notification messages are concise and convey the intended information clearly.
- Opt-Out Options: Provide users with the ability to opt out of notifications or customize their preferences.
- A/B Testing: Experiment with different notification strategies and measure their impact on user engagement.
Conclusion
User notifications are a powerful tool for keeping users informed and engaged in your PhoneGap app. By understanding the various types of notifications and following best practices, you can enhance the user experience and drive higher user retention rates.
Remember that effective notification strategies require careful planning and consideration of user preferences. By implementing notifications thoughtfully, you can create a more engaging and user-friendly app that keeps users coming back for more. So, go ahead, start implementing user notifications in your PhoneGap app, and watch your user engagement soar!
Table of Contents
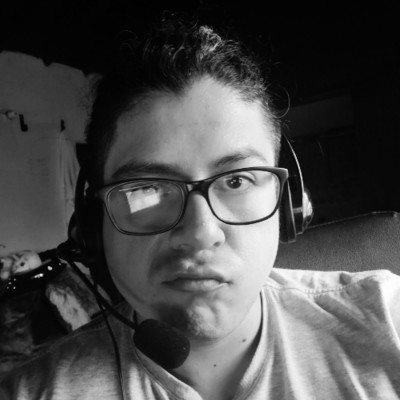
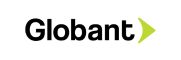