An In-Depth Look at PHP’s array_sum() Function
PHP’s array_sum() function is a straightforward yet powerful tool for summing the values in an array. Whether you’re working with simple arrays of numbers or need to handle more complex scenarios, understanding how to use array_sum() can greatly simplify your coding tasks. In this blog, we’ll explore the function in detail, including its syntax, usage, and practical examples.
What is the array_sum() Function?
The array_sum() function is a built-in PHP function that calculates the sum of all the values in an array. It is particularly useful when you need to quickly total numerical values stored in an array.
Syntax
```php int array_sum(array $array) ```
- $array: The input array containing the values to be summed.
- Returns: The sum of all values in the array as an integer or float, depending on the values present.
Basic Usage
Let’s start with a simple example. Suppose we have an array of integers and want to find their sum:
```php $numbers = [1, 2, 3, 4, 5]; $sum = array_sum($numbers); echo $sum; // Output: 15 ```
In this example, array_sum() takes the array $numbers and returns the sum of its elements.
Working with Different Data Types
One of the strengths of array_sum() is its ability to handle arrays with mixed data types. It automatically converts elements to numbers as needed, skipping non-numeric values.
Example with Mixed Data Types
```php $mixed = [1, "2", 3.5, "four", 5]; $sum = array_sum($mixed); echo $sum; // Output: 11.5 ```
In this case, the function ignores the string “four” and sums the remaining numeric values.
Handling Associative Arrays
array_sum() can also be used with associative arrays. The function only considers the values, ignoring the keys.
Example with Associative Arrays
```php $associative = ["a" => 1, "b" => 2, "c" => 3]; $sum = array_sum($associative); echo $sum; // Output: 6 ```
Here, array_sum() totals the values without regard to the keys.
Practical Applications
The array_sum() function can be particularly useful in a variety of real-world scenarios. Let’s explore a couple of practical applications.
Calculating Total Sales
Imagine you have an array representing daily sales amounts. You can easily calculate the total sales using array_sum():
```php $sales = [120.50, 340.75, 560.25]; $total_sales = array_sum($sales); echo "Total Sales: $" . $total_sales; // Output: Total Sales: $1021.5 ```
Summing Up Scores
In a game, you might need to calculate the total score of a player:
```php $scores = [10, 20, 30, 25]; $total_score = array_sum($scores); echo "Total Score: " . $total_score; // Output: Total Score: 85 ```
Best Practices and Considerations
While array_sum() is easy to use, there are some best practices to keep in mind:
Input Validation
Ensure that the array contains only the types of data you intend to sum. Although array_sum() skips non-numeric values, it’s good practice to validate your input data to avoid unexpected results.
Performance Considerations
For very large arrays, consider the performance implications. While array_sum() is efficient, processing extremely large datasets can still impact performance. Optimize your array management and consider alternatives if necessary.
Conclusion
PHP’s array_sum() function is a versatile tool for summing array values. Its ability to handle various data types and array structures makes it invaluable for many applications. By understanding its capabilities and limitations, you can leverage array_sum() to write cleaner, more efficient code.
Further Reading
Table of Contents
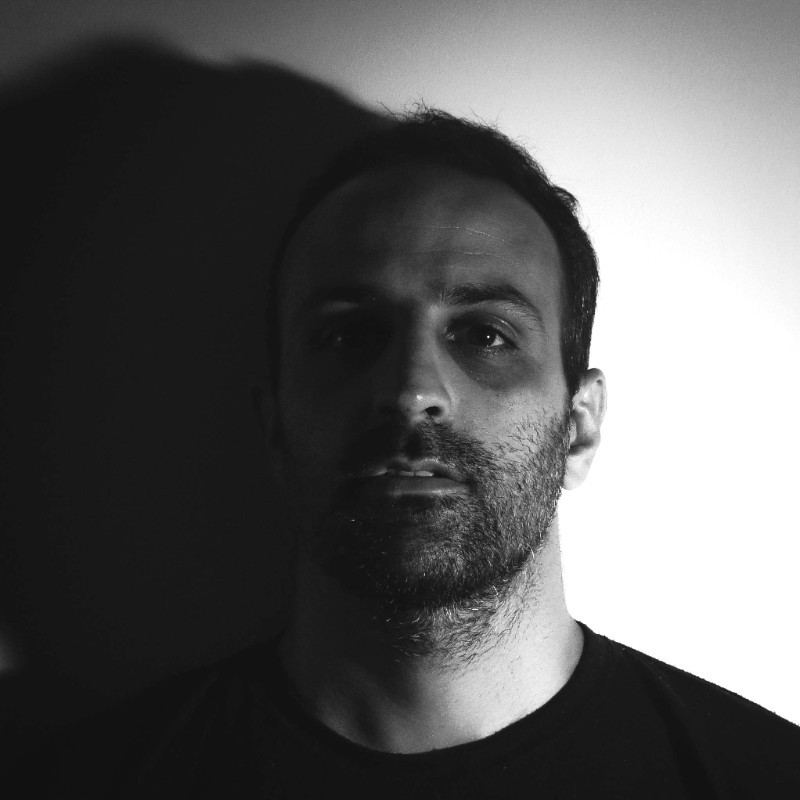
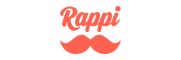