How to create classes and objects?
Creating classes and objects in PHP is fundamental to object-oriented programming (OOP). Classes serve as blueprints or templates for creating objects, while objects represent instances of those classes. Here’s a step-by-step guide on how to create classes and objects in PHP:
- Class Declaration:
To create a class in PHP, use the `class` keyword followed by the class name. Class names conventionally start with an uppercase letter. Inside the class, you can define properties (variables) and methods (functions) that characterize the class.
```php class Car { // Properties public $brand; public $model; // Methods public function startEngine() { return "Engine started!"; } } ```
- Object Creation:
To create an object from a class, use the `new` keyword followed by the class name, and assign it to a variable. This variable represents the object and can be used to access its properties and methods.
```php $myCar = new Car(); ```
- Accessing Properties and Methods:
Once you have an object, you can access its properties and methods using the arrow `->` operator.
```php $myCar->brand = "Toyota"; $myCar->model = "Camry"; echo $myCar->startEngine(); // Outputs: "Engine started!" ```
- Constructors:
You can create constructors, special methods with the name `__construct()`, to initialize object properties when an object is created. Constructors are called automatically when an object is instantiated.
```php class Car { public $brand; public $model; public function __construct($brand, $model) { $this->brand = $brand; $this->model = $model; } } ```
- Multiple Objects:
You can create multiple objects from the same class, each with its own set of properties and values.
```php $car1 = new Car("Toyota", "Camry"); $car2 = new Car("Honda", "Civic"); ```
Creating classes and objects in PHP allows you to encapsulate data and behavior, enabling you to model real-world entities and create reusable and structured code. This is a fundamental concept in object-oriented programming and is widely used in PHP application development.
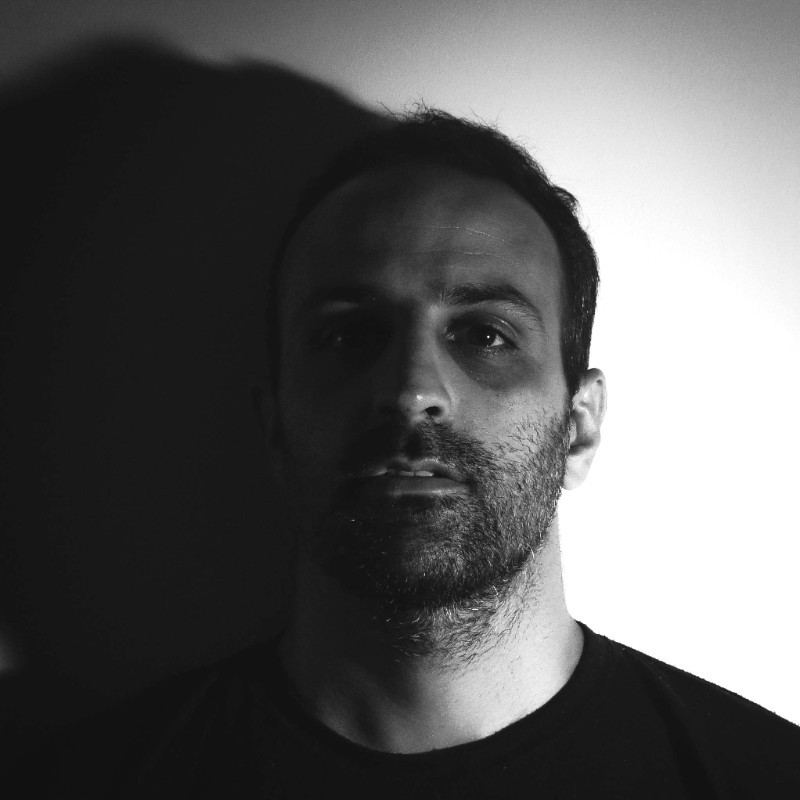
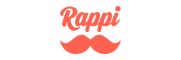