How to create and use traits?
PHP traits are a powerful feature that allows you to reuse code in multiple classes without the need for inheritance, making your code more modular and maintainable. Traits provide a way to share methods and properties among different classes in PHP. Here’s a guide on how to create and use PHP traits:
Creating a Trait:
- Define the Trait: To create a trait, use the `trait` keyword followed by the trait’s name. Inside the trait, you can define methods and properties that you want to reuse in other classes.
```php trait MyTrait { public function sharedMethod() { // Trait method logic } } ```
Using a Trait:
- Include the Trait in a Class: To use a trait in a class, you use the `use` statement followed by the trait’s name inside the class definition.
```php class MyClass { use MyTrait; // Other class properties and methods } ```
Now, the `sharedMethod` from `MyTrait` is available in `MyClass`. You can use it as if it were defined directly in `MyClass`.
Using Multiple Traits:
- Combining Traits: You can use multiple traits in a single class by separating them with commas in the `use` statement.
```php class AnotherClass { use MyTrait, AnotherTrait, YetAnotherTrait; // Other class properties and methods } ```
Method Resolution Order (MRO):
- Handle Trait Conflict: When multiple traits used in a class have methods with the same name, PHP resolves conflicts following a specific order called the Method Resolution Order (MRO). You can explicitly specify which method to use using the `insteadof` or `as` operators.
```php class ConflictClass { use MyTrait, AnotherTrait { MyTrait::sharedMethod insteadof AnotherTrait; AnotherTrait::conflictingMethod as aliasMethod; } } ```
Benefits of Traits:
Traits help you avoid issues related to single inheritance in PHP and promote code reuse. You can define common functionality once in a trait and use it across various classes without creating complex class hierarchies. This makes your code more modular and easier to maintain.
PHP traits are a valuable tool for code organization and reuse. They allow you to encapsulate and share functionality across multiple classes without the limitations of traditional inheritance, providing flexibility and maintainability in your PHP projects.
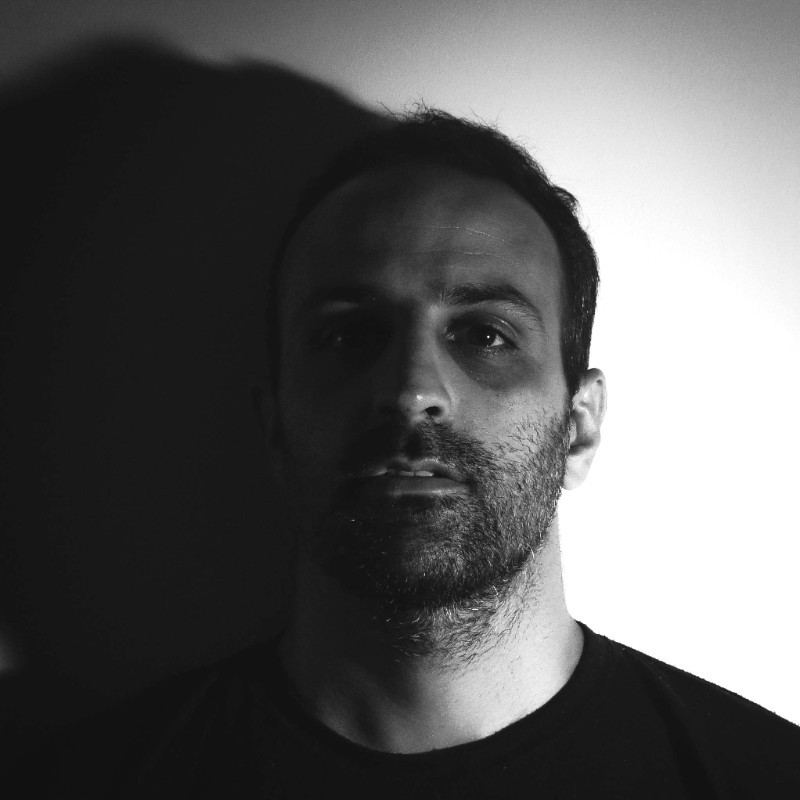
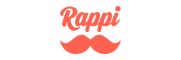