How to use dependency injection?
Dependency injection is a design pattern in PHP (and many other programming languages) used to manage the dependencies of objects more efficiently and make the code more maintainable, flexible, and testable. It’s a crucial concept in modern PHP development, especially in the context of object-oriented programming.
Here’s a concise guide on how to use dependency injection in PHP:
- Understanding Dependency Injection:
Dependency injection involves injecting objects or values that a class depends on (its dependencies) from the outside, rather than creating them internally. This reduces a class’s coupling to its dependencies and makes it easier to change or test.
- Constructor Injection:
The most common form of dependency injection is through a class’s constructor. You pass the required dependencies as arguments when creating an instance of the class. For example:
```php class UserService { private $userRepository; public function __construct(UserRepository $userRepository) { $this->userRepository = $userRepository; } // ... } ```
- Setter Injection:
Another approach is setter injection, where you provide setter methods for dependencies. This allows you to set dependencies after the object is constructed. For example:
```php class UserService { private $userRepository; public function setUserRepository(UserRepository $userRepository) { $this->userRepository = $userRepository; } // ... } ```
- Interface and Container:
Often, you’ll use interfaces to define contracts for your dependencies. Dependency injection containers or service containers like Symfony’s DependencyInjection component can manage the instantiation and injection of dependencies for you, based on the configured services.
- Benefits of Dependency Injection:
– Testability: It’s easier to write unit tests for classes with injected dependencies because you can provide mock objects or stubs.
– Flexibility: You can easily change dependencies or switch implementations without modifying the class that depends on them.
– Reusability: Injected dependencies can be reused across multiple classes, promoting the DRY (Don’t Repeat Yourself) principle.
- Best Practices:
When using dependency injection, follow best practices like adhering to the Single Responsibility Principle, favoring composition over inheritance, and using meaningful interfaces and class names.
- Dependency Injection Containers:
For larger projects, consider using a dedicated dependency injection container library or framework component to manage and automate the injection of dependencies.
Dependency injection is a powerful technique in PHP that enhances code maintainability and testability by reducing tight coupling between classes. By following best practices and using dependency injection containers, you can simplify the management of dependencies in your PHP applications.
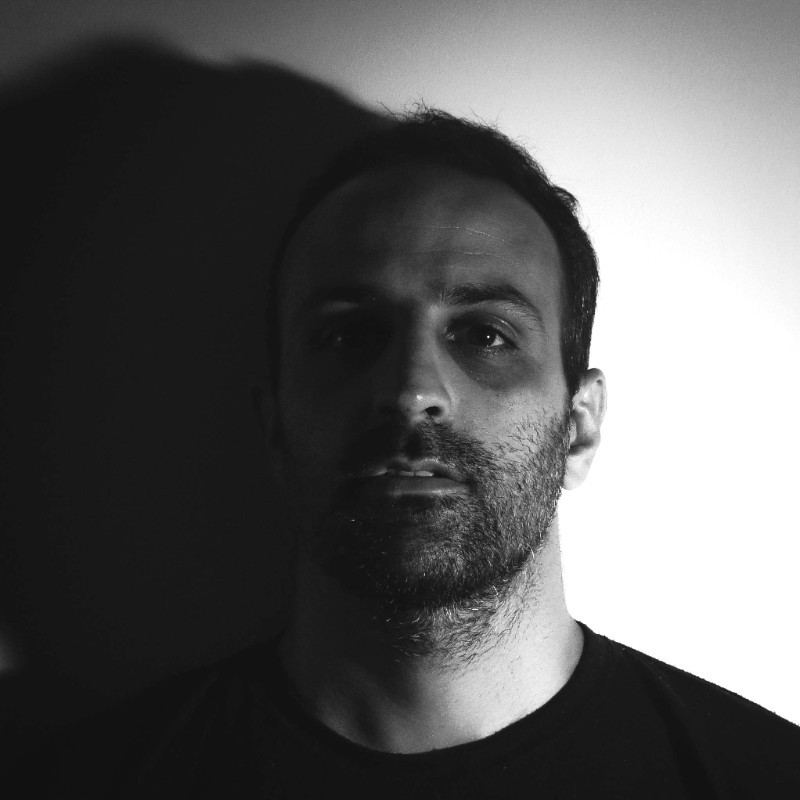
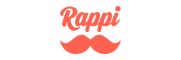