Getting Started with PHP’s dirname() Function
PHP’s dirname() function is a fundamental tool for managing directory paths. Whether you’re working on a simple script or a complex web application, understanding how to use this function can streamline your development process. In this blog, we’ll delve into the dirname() function, exploring its syntax, common use cases, and practical examples.
What is the dirname() Function?
The dirname() function in PHP returns the directory path of a given file path. It’s a handy way to extract the parent directory from a full file path, making it easier to manage file systems and navigate directories.
Syntax
The basic syntax of the dirname() function is as follows:
```php string dirname ( string $path [, int $levels = 1 ] ) ```
- $path: The full path of the file.
- $levels: Optional. Specifies the number of parent directories to go up. Default is 1.
Basic Usage of dirname()
The dirname() function is straightforward to use. Let’s start with a simple example:
Example 1: Getting the Parent Directory
```php <?php $filePath = '/var/www/html/index.php'; $directory = dirname($filePath); echo $directory; // Output: /var/www/html ?> ```
In this example, dirname() extracts the parent directory /var/www/html from the full file path /var/www/html/index.php.
Example 2: Navigating Multiple Levels Up
You can also specify the number of levels to go up in the directory structure by using the $levels parameter:
```php <?php $filePath = '/var/www/html/project/index.php'; $directory = dirname($filePath, 2); echo $directory; // Output: /var/www ?> ```
Here, dirname($filePath, 2) navigates two levels up from /var/www/html/project/index.php, resulting in /var/www.
Practical Applications of dirname()
The dirname() function is not just for extracting directory names. It’s also useful in various practical scenarios, such as organizing file structures, including files dynamically, and managing configuration paths.
Example 3: Dynamic File Inclusion
One common use case for dirname() is dynamically including files. For example, you can use it to include a configuration file located in the parent directory:
```php <?php // Define the base directory $baseDir = dirname(__FILE__); // Include the configuration file include $baseDir . '/config.php'; ?> ```
In this script, dirname(__FILE__) returns the directory of the current file, allowing you to include config.php from the parent directory.
Example 4: Secure File Path Handling
When dealing with user inputs for file paths, using dirname() can help prevent directory traversal attacks by ensuring that paths are resolved securely. For example:
```php <?php $userInput = '../uploads/file.txt'; $securePath = dirname($userInput) . '/' . basename($userInput); // Prevents paths like /etc/passwd from being accessed echo $securePath; // Output: ../uploads/file.txt ?> ```
By breaking down the path and reassembling it with dirname() and basename(), you can mitigate potential security risks.
Conclusion
The dirname() function is a powerful tool for managing file paths in PHP. Its simplicity and versatility make it an essential function for developers. Whether you’re including files dynamically or securing user inputs, understanding how to leverage dirname() can enhance your PHP applications.
Further Reading
Table of Contents
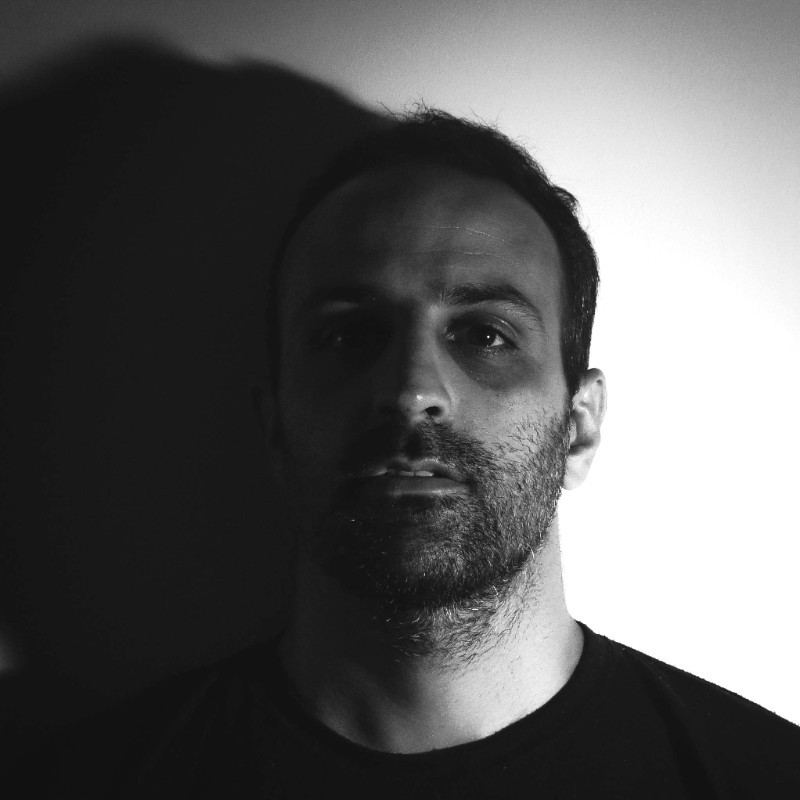
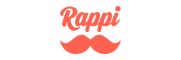