Exploring the Magic of PHP’s htmlspecialchars() Function
When working with PHP, ensuring that your web application is secure and resilient to attacks is crucial. One of the fundamental tools in this regard is the htmlspecialchars() function. This function plays a vital role in protecting your application from cross-site scripting (XSS) attacks by converting special characters to HTML entities. In this blog, we’ll delve into how htmlspecialchars() works, why it’s essential, and how to use it effectively.
What is htmlspecialchars()?
The htmlspecialchars() function is a built-in PHP function that converts special characters to HTML entities. This is particularly useful when displaying user-generated content on a webpage. The function is essential in preventing XSS attacks, where an attacker injects malicious scripts into web pages viewed by other users.
How htmlspecialchars() Works
The htmlspecialchars() function takes a string and converts predefined characters to their respective HTML entities. This process helps ensure that the string is safely displayed in the browser without being interpreted as executable code. The main characters that htmlspecialchars() converts are:
- & (ampersand) to &
- < (less than) to <
- > (greater than) to >
- “ (double quote) to "
- ‘ (single quote) to ' (optional, based on flags)
Here’s a simple example to illustrate its usage:
```php <?php $user_input = '<script>alert("XSS Attack!");</script>'; $safe_output = htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8'); echo $safe_output; ?> ```
In this example, the htmlspecialchars() function converts the script tags into HTML entities, rendering them harmless when displayed on a web page.
Why Use htmlspecialchars()?
Using htmlspecialchars() is essential for several reasons:
1. Preventing XSS Attacks
By converting special characters to HTML entities, htmlspecialchars() prevents any embedded scripts from being executed in the browser. This helps protect users from malicious code injected through forms or URLs.
2. Displaying User-Generated Content Safely
When you allow users to input data, such as comments or forum posts, it’s crucial to display this data safely. Without using htmlspecialchars(), user inputs could contain harmful code that affects other users or compromises your site.
3. Maintaining Data Integrity
The function ensures that data remains intact and displayed as intended. It prevents characters from being misinterpreted as HTML or JavaScript code.
Using htmlspecialchars() in Different Contexts
Displaying User Inputs in HTML
When you display user inputs in HTML content, htmlspecialchars() is typically used to ensure safety. For example:
```php <?php $user_input = $_POST['user_input']; $safe_output = htmlspecialchars($user_input, ENT_QUOTES, 'UTF-8'); echo "<p>User says: $safe_output</p>"; ?> ```
Encoding for JavaScript
When passing data to JavaScript, you might need to use htmlspecialchars() to avoid issues with special characters. Here’s an example:
```php <?php $data = '<div onclick="alert(\'Hello\')">Click me</div>'; $safe_data = htmlspecialchars($data, ENT_QUOTES, 'UTF-8'); echo "<script>var data = '$safe_data'; console.log(data);</script>"; ?> ```
Form Handling
When processing form submissions, use htmlspecialchars() to safely display the submitted data:
```php <?php if ($_SERVER["REQUEST_METHOD"] == "POST") { $name = htmlspecialchars($_POST['name'], ENT_QUOTES, 'UTF-8'); echo "Hello, $name!"; } ?> ```
Conclusion
The htmlspecialchars() function is a powerful tool for ensuring web application security and data integrity. By converting special characters to HTML entities, it helps protect against XSS attacks and ensures that user-generated content is displayed safely. Incorporating htmlspecialchars() into your PHP applications is a crucial step in maintaining a secure and reliable web environment.
Further Reading
Table of Contents
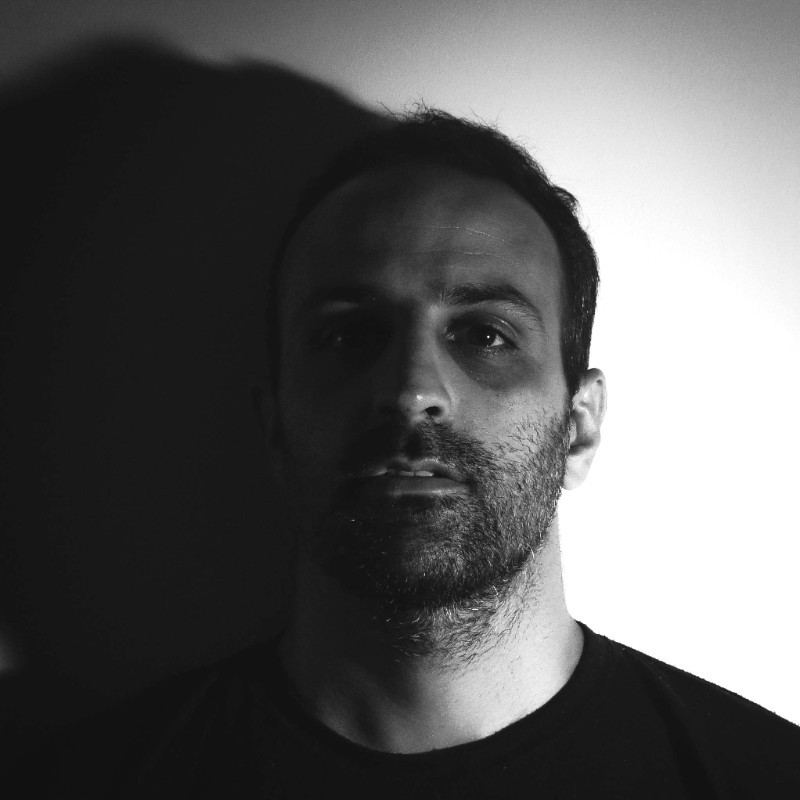
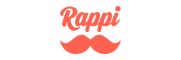