How to implement user authentication?
Implementing user authentication in a PHP application is crucial for securing user data and restricting access to certain parts of the application. Here’s a step-by-step guide on how to achieve user authentication in PHP:
- Database Setup:
– Start by creating a database table to store user information, including fields like username, email, password (hashed), and any additional user-related data. Hash the passwords using a strong hashing algorithm like bcrypt.
- User Registration:
– Create a registration form that allows users to sign up. Validate and sanitize user input to prevent SQL injection and other security vulnerabilities. When a user registers, insert their information into the database after hashing their password.
- Login System:
– Design a login form where users can enter their credentials. Upon submission, retrieve the user’s hashed password from the database and compare it to the entered password using password_verify(). If they match, create a session to keep the user logged in.
- Session Management:
– Implement session management to track user sessions. PHP provides built-in session handling functions for this purpose. Store user information (e.g., user ID) in the session to identify authenticated users.
- Access Control:
– Define which parts of your application require authentication. For example, you may have certain pages or functionality that should only be accessible to logged-in users. Implement access control checks to verify if a user is authenticated before granting access.
- Logout:
– Create a logout mechanism that destroys the user’s session, effectively logging them out. This prevents unauthorized access to protected areas.
- Password Reset:
– Implement a password reset mechanism that allows users to reset their passwords in case they forget them. This typically involves sending a reset link to the user’s email and handling the password update securely.
- Security Considerations:
– Pay attention to security best practices, such as using prepared statements for database queries, protecting against Cross-Site Request Forgery (CSRF) attacks, and implementing brute-force protection.
By following these steps, you can implement user authentication in your PHP application, enhancing its security and ensuring that only authorized users can access protected areas. Remember to keep your authentication system up to date with security patches and best practices to protect user data effectively.
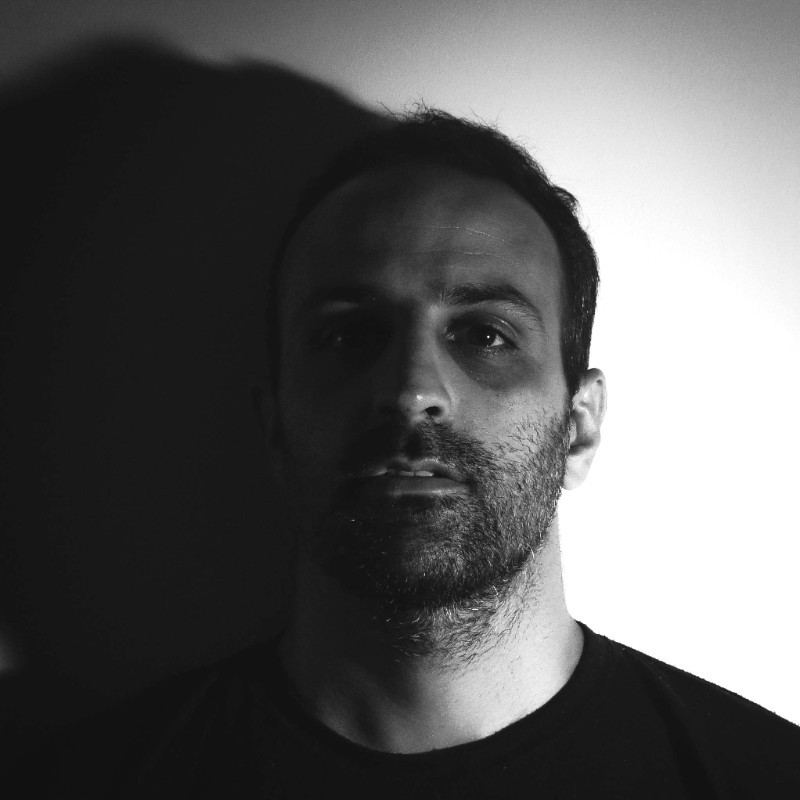
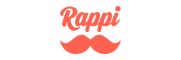