How to connect to a MySQL database?
Connecting to a MySQL database in PHP is a fundamental step when building dynamic web applications that require data storage and retrieval. Here’s a step-by-step guide on how to establish a connection:
- Install and Set Up MySQL:
– Before connecting to a MySQL database, ensure that you have MySQL installed and configured on your server. You’ll also need to create a database and a user with appropriate permissions.
- PHP MySQL Extension:
– PHP offers several extensions for MySQL, with the most commonly used one being MySQLi (MySQL Improved). To use it, make sure the `mysqli` extension is enabled in your PHP configuration. You can check this using the `phpinfo()` function.
- Connection Parameters:
– To connect to a MySQL database, you’ll need the following information:
– Database host (usually “localhost” or an IP address)
– Database username
– Database password
– Database name
- Establish a Connection:
– Use the `mysqli_connect()` function to establish a connection to the MySQL database. Provide the connection parameters as arguments to the function.
```php $host = "localhost"; $username = "your_username"; $password = "your_password"; $database = "your_database"; $conn = mysqli_connect($host, $username, $password, $database); if (!$conn) { die("Connection failed: " . mysqli_connect_error()); } ```
- Query Execution:
– Once the connection is established, you can execute SQL queries using `mysqli_query()`. Make sure to handle errors gracefully by checking the result of each query.
- Closing the Connection:
– It’s essential to close the database connection when you’re done with it using `mysqli_close($conn)` to free up server resources.
Connecting to a MySQL database in PHP is a crucial step in building data-driven web applications. By following these steps, you can establish a secure and efficient connection to your MySQL database and interact with it using PHP scripts.
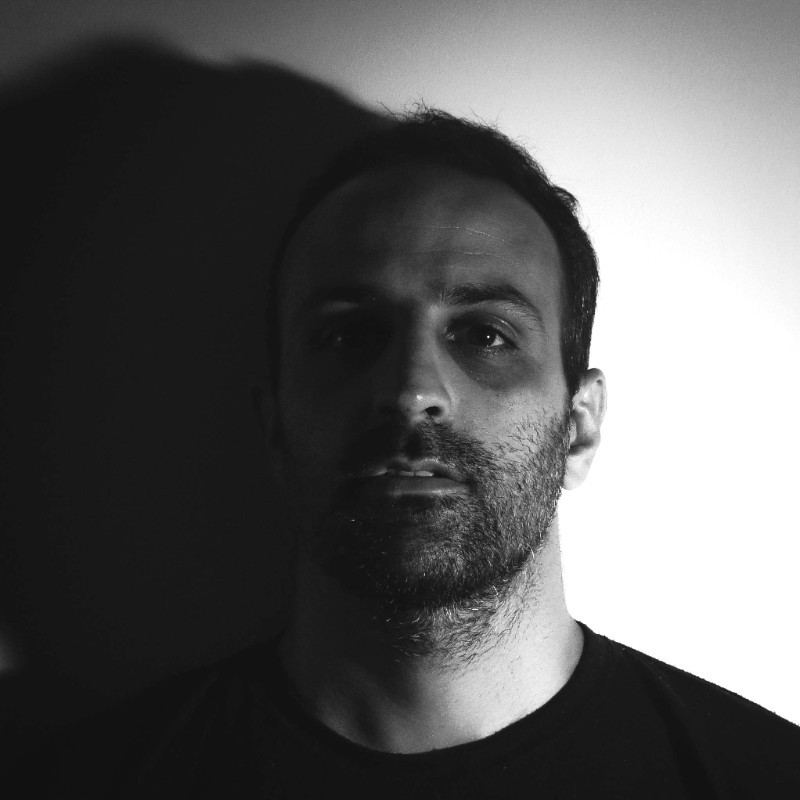
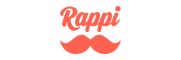