How to create a RESTful API using CodeIgniter?
Creating a RESTful API using CodeIgniter, a lightweight and user-friendly PHP framework, can be accomplished with a straightforward approach. Here’s a step-by-step guide on how to create a RESTful API using CodeIgniter:
- Install CodeIgniter:
First, ensure that CodeIgniter is installed on your system. You can download the latest version from the official website or use Composer to install it.
- Configure Routes:
CodeIgniter manages routes in the `application/config/routes.php` file. Define routes for your API endpoints using a simple and intuitive syntax:
```php $route['api/resource'] = 'ApiController/resource'; ```
- Create a Controller:
Generate a controller to handle API requests. CodeIgniter makes this easy with its CLI tool. Open your terminal and navigate to your project’s root directory:
``` php spark make:controller ApiController ```
- Implement Controller Methods:
In the generated controller, define methods to handle different API endpoints. For example, you can create an `index` method to fetch and return a list of resources:
```php public function resource() { $data = $this->resourceModel->getAllResources(); return $this->response->setJSON($data); } ```
- Database Models:
Create models for your database tables and configure them to interact with your database using CodeIgniter’s query builder or an ORM like Eloquent.
- Validation:
Ensure data integrity by implementing validation rules using CodeIgniter’s form validation library.
- Serialization and Deserialization:
Serialize data to JSON format for responses and deserialize JSON data into PHP objects for requests.
- Authentication and Authorization:
Implement authentication and authorization logic as per your API’s requirements, such as API tokens or OAuth2.
- Testing:
CodeIgniter supports unit testing, so write tests to ensure the functionality of your API endpoints.
- API Documentation:
Document your API endpoints for developers to understand how to use them efficiently.
- Deployment:
Deploy your CodeIgniter-based RESTful API to a web server or cloud hosting platform.
CodeIgniter’s simplicity and a small learning curve make it suitable for quickly building RESTful APIs. By following these steps and utilizing CodeIgniter’s built-in features and libraries, you can develop a robust and functional API to serve your application’s needs effectively.
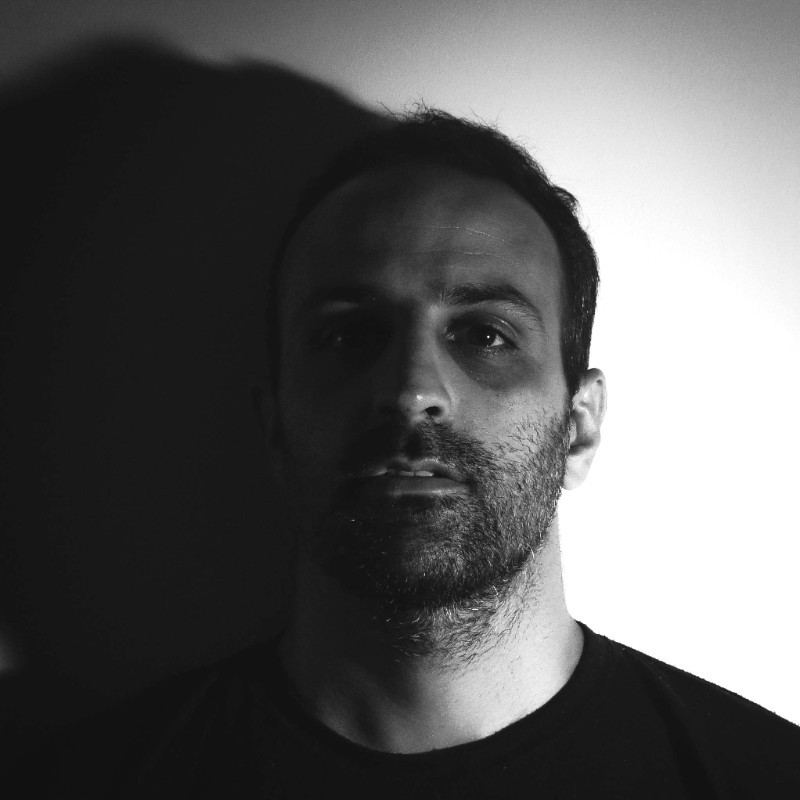
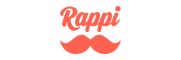