How to create a RESTful API using Laravel?
Creating a RESTful API using Laravel, a popular PHP framework, is a streamlined process that leverages Laravel’s built-in features for routing, controllers, and data handling. Here’s a step-by-step guide:
- Install Laravel:
First, make sure you have Laravel installed on your system. You can use Composer, a PHP package manager, to create a new Laravel project with the following command:
``` composer create-project --prefer-dist laravel/laravel api-project ```
- Set Up Routes:
Laravel uses routes to define API endpoints. In the `routes/api.php` file, you can define the routes for your API. For example, to create a route for retrieving a list of resources, you can use the `Route::get` method:
```php Route::get('/resources', 'ResourceController@index'); ```
- Create Controllers:
Next, generate a controller that will handle API requests. You can use the artisan command-line tool to create a controller:
``` php artisan make:controller ResourceController ```
- Define Controller Actions:
In the generated controller, define methods corresponding to the API endpoints you’ve created in your routes. For example, the `index` method in the `ResourceController` could retrieve a list of resources from your database and return them as JSON:
```php public function index() { $resources = Resource::all(); return response()->json($resources); } ```
- Set Up Database Models:
Laravel’s Eloquent ORM makes it easy to work with your database. Create a model for each resource and define relationships if necessary:
``` php artisan make:model Resource ```
- Migrate and Seed Database:
Use migrations to create your database schema and seed it with sample data if needed:
``` php artisan migrate php artisan db:seed ```
- Middleware and Authentication:
If your API requires authentication, Laravel provides middleware like Passport for implementing OAuth2-based authentication.
- Validation:
Implement data validation using Laravel’s validation rules to ensure that incoming requests are well-formed.
- Testing:
Laravel has built-in support for testing APIs. Write tests to verify that your API endpoints work correctly.
- API Documentation:
Consider using tools like Swagger or Laravel-based packages to document your API endpoints for developers.
- Deployment:
Deploy your Laravel-based RESTful API to a web server or cloud hosting platform of your choice.
By following these steps, you can create a RESTful API using Laravel quickly and efficiently. Laravel’s elegant syntax and powerful features make it an excellent choice for building robust APIs for your web applications.
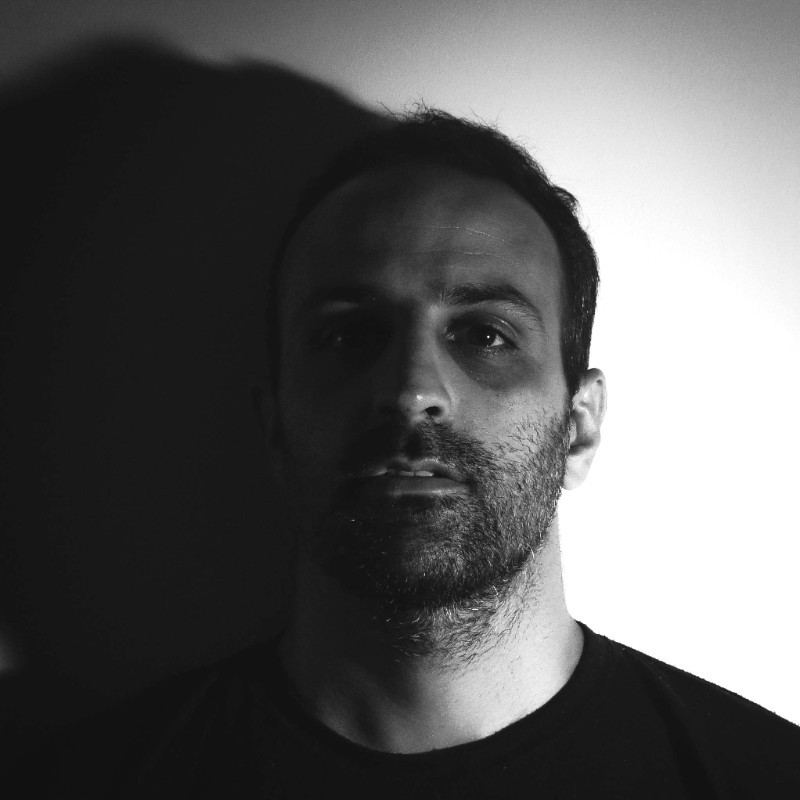
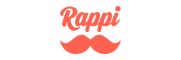