How to create a RESTful API using Symfony?
Creating a RESTful API using Symfony, a robust PHP framework, provides developers with a structured and efficient way to build web services. Here’s a step-by-step guide on how to create a RESTful API with Symfony:
- Install Symfony:
Ensure that Symfony is installed on your system. You can use Composer, the PHP package manager, to create a new Symfony project:
``` composer create-project symfony/skeleton api-project ```
- Define Routes:
In Symfony, routes are defined in the `config/routes/routes.yaml` file. Create routes for your API endpoints using YAML configuration. For example:
```yaml api_resource_collection: path: '/api/resources' controller: 'App\Controller\ResourceController::index' methods: ['GET'] ```
- Create a Controller:
Generate a controller that will handle API requests. Symfony’s MakerBundle simplifies this process:
``` php bin/console make:controller ResourceController ```
- Implement Controller Actions:
In the generated controller, create methods to handle API endpoints. For instance, the `index` method could retrieve a list of resources and return them as JSON:
```php public function index(ResourceRepository $resourceRepository): JsonResponse { $resources = $resourceRepository->findAll(); return $this->json($resources); } ```
- Database Models and Doctrine:
Symfony integrates with Doctrine, an ORM (Object-Relational Mapping) tool. Define your database models as entities and create migrations to generate your database schema:
``` php bin/console make:entity php bin/console make:migration php bin/console doctrine:migrations:migrate ```
- Serialization and Deserialization:
Symfony provides tools like Symfony Serializer to serialize data into JSON for responses and deserialize JSON into objects for requests.
- Authentication and Authorization:
Implement authentication and authorization using Symfony’s security component. You can use various authentication methods, including API tokens or OAuth2.
- Validation:
Ensure data integrity by validating incoming requests with Symfony’s built-in validation tools.
- Testing:
Symfony’s testing framework allows you to write functional and unit tests for your API endpoints.
- API Documentation:
Consider using tools like API Platform or Swagger to document your API and make it easier for developers to understand and use.
- Deployment:
Deploy your Symfony-based RESTful API to a web server or a cloud hosting platform of your choice.
By following these steps, you can create a powerful and scalable RESTful API using Symfony. Symfony’s flexibility and extensive ecosystem of bundles and libraries make it an excellent choice for building APIs that meet the demands of modern web applications.
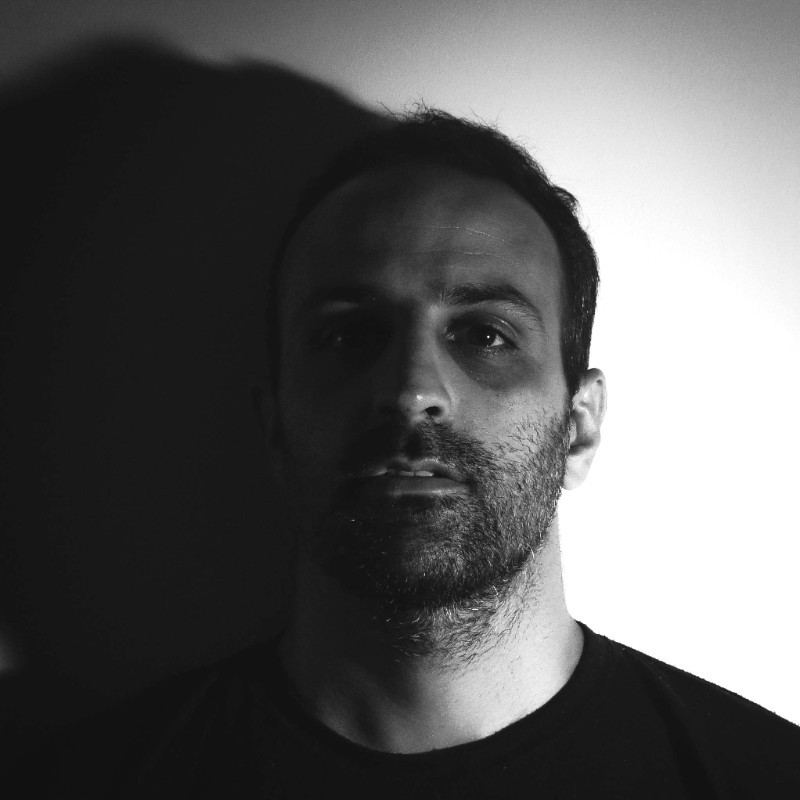
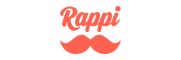