PHP Q & A
How to start and destroy sessions?
Starting and destroying sessions in PHP is a crucial part of managing user data and maintaining stateful information across web applications. Here’s a step-by-step guide on how to start and destroy sessions in PHP:
Starting a Session:
- Initialization: To start a session in PHP, you need to call the `session_start()` function. This function should be called at the beginning of every script where you intend to use session variables. It initializes or resumes the session.
```php session_start(); ```
- Session Variables: After starting the session, you can store and access data in the `$_SESSION` super global array. This array is used to persist data across multiple HTTP requests within the same session.
```php $_SESSION['username'] = 'John'; ```
Destroying a Session:
- Session Logout: To end a user’s session or log them out, you can use the `session_destroy()` function. This function destroys all session data associated with the user, effectively logging them out.
```php session_destroy(); ```
- Unsetting Variables: While `session_destroy()` destroys the session data on the server, it’s also a good practice to unset individual session variables before destroying the entire session.
```php unset($_SESSION['username']); ```
- Session Cookie: To ensure that the session cookie is deleted from the user’s browser, you should also set its expiration time to the past:
```php setcookie(session_name(), '', time() - 3600, '/'); ```
By following these steps, you can effectively start and destroy sessions in PHP. Starting a session is crucial for managing user data, while destroying a session is essential for user logout and ensuring data privacy. Proper session management helps create secure and stateful web applications.
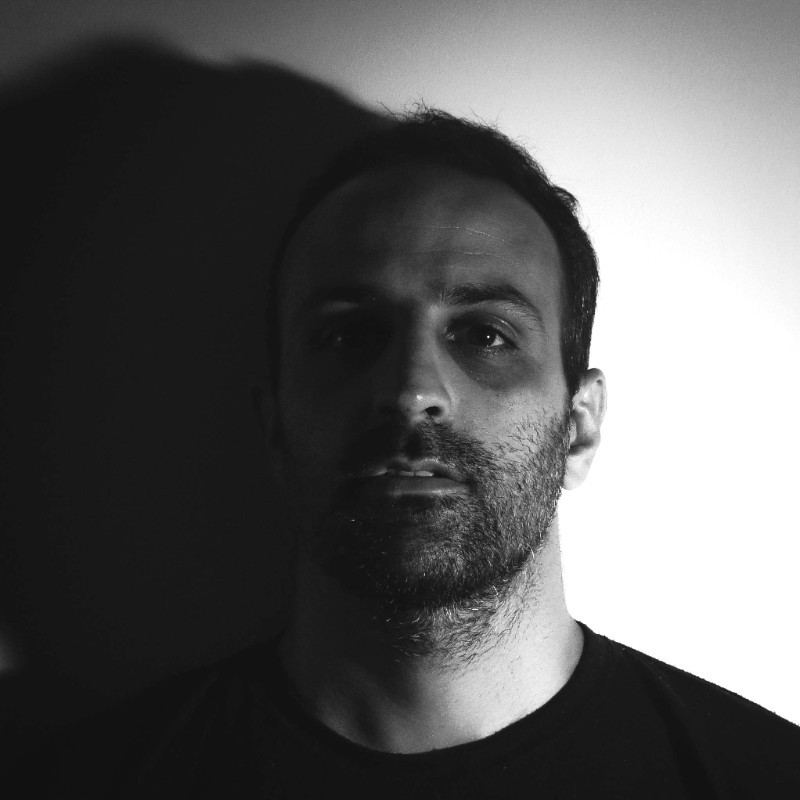
Previously at
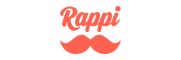
Full Stack Engineer with extensive experience in PHP development. Over 11 years of experience working with PHP, creating innovative solutions for various web applications and platforms.