PHP’s str_split() Function: Splitting Strings into Arrays
PHP provides a rich set of functions for string manipulation, one of which is str_split(). This function is particularly useful when you need to divide a string into an array of smaller substrings. In this blog, we’ll explore the str_split() function, its syntax, and practical use cases. We’ll also provide code samples to illustrate how to use it effectively.
What is the str_split() Function?
The str_split() function is a built-in PHP function that splits a string into an array. It takes two arguments: the string to be split and an optional length parameter that defines the size of each chunk. If the length parameter is not specified, the function splits the string into individual characters.
Syntax
```php str_split(string $string, int $length = 1): array ```
- $string: The input string that you want to split.
- $length: Optional. The length of each chunk. The default value is 1, meaning the string will be split into individual characters.
Basic Usage of str_split()
Let’s start with a simple example to understand the basic usage of str_split().
```php <?php $string = "Hello, World!"; $array = str_split($string); print_r($array); ?> ```
Output:
``` Array ( [0] => H [1] => e [2] => l [3] => l [4] => o [5] => , [6] => [7] => W [8] => o [9] => r [10] => l [11] => d [12] => ! ) ```
In this example, the string “Hello, World!” is split into an array of individual characters.
Splitting Strings into Specific Lengths
You can also specify the length of each chunk. For instance, if you want to split the string into chunks of two characters, you can set the length parameter to 2.
Example with Length Parameter
```php <?php $string = "abcdefg"; $array = str_split($string, 2); print_r($array); ?> ```
Output:
``` Array ( [0] => ab [1] => cd [2] => ef [3] => g ) ```
In this example, the string “abcdefg” is split into chunks of two characters each.
Handling Multibyte Strings
It’s important to note that str_split() does not work well with multibyte strings, as it may not properly handle characters outside the ASCII range. For multibyte string handling, consider using the mb_str_split() function available in PHP 7.4 and above.
Example with mb_str_split()
```php <?php $string = "?????"; $array = mb_str_split($string); print_r($array); ?> ```
Output:
``` Array ( [0] => ? [1] => ? [2] => ? [3] => ? [4] => ? ) ```
In this case, the Japanese string “?????” is split into individual characters correctly.
Use Cases and Applications
The str_split() function can be particularly useful in various scenarios, such as:
- Text Analysis: Breaking down text into individual characters or groups for analysis.
- Data Sanitization: Splitting and analyzing user input to ensure it meets certain criteria.
- Encoding and Decoding: Processing data that needs to be split into smaller chunks for encoding or decoding purposes.
Conclusion
The str_split() function is a powerful tool in PHP for splitting strings into arrays. Whether you’re working with simple ASCII strings or multibyte characters, understanding how to effectively use str_split() can simplify many string manipulation tasks. Just be cautious with multibyte strings and consider using mb_str_split() when necessary.
Further Reading
Table of Contents
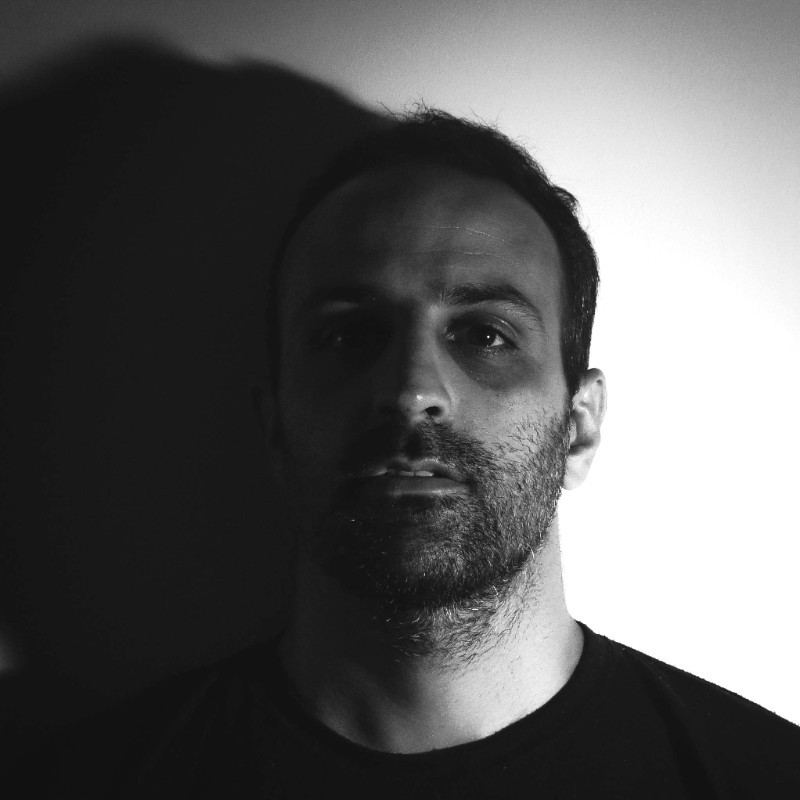
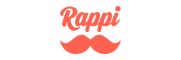