What is a context manager in Python?
A context manager in Python is a design pattern that facilitates the allocation and deallocation of resources, ensuring that certain tasks are performed before and after a section of code. The most common use case is file handling, where a file needs to be opened, then eventually closed, whether the operations in between were successful or not.
Understanding Context Managers:
- The `with` Statement:
Context managers are typically used in combination with the `with` statement. The primary advantage is that it ensures resources are efficiently managed. For example, when working with files:
```python with open('file.txt', 'r') as file: content = file.read() ```
Here, after executing the block of code inside the `with` statement, the file is automatically closed, even if an exception occurs within the block.
- How They Work:
At the heart of a context manager are two magic methods: `__enter__()` and `__exit__()`.
– `__enter__()` is called when the `with` block is entered. It can return an object that will be used within the block.
– `__exit__()` is called when the `with` block is exited. It handles cleanup operations, like closing a file or releasing a lock.
- Creating Custom Context Managers:
You can create your own context manager by defining a class with `__enter__()` and `__exit__()` methods:
```python class MyContextManager: def __enter__(self): # Initialization or resource allocation return self def __exit__(self, exc_type, exc_value, traceback): # Cleanup or resource deallocation pass ```
- The `contextlib` Module:
Python’s standard library provides a module named `contextlib` which offers utilities to create context managers without always defining a new class. The `@contextmanager` decorator allows you to build a context manager using a generator function:
```python from contextlib import contextmanager @contextmanager def managed_resource(): resource = allocate_resource() yield resource resource.deallocate() ```
Context managers in Python provide a structured and safe way to manage resources, ensuring that initialization and cleanup tasks are reliably executed. By leveraging context managers, developers can write cleaner, more maintainable, and error-resistant code, especially when dealing with external resources or operations that require precise setup and teardown procedures.
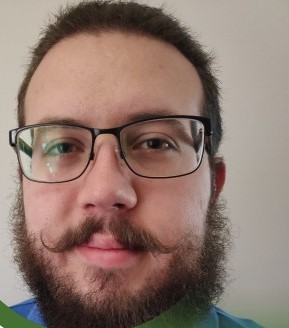
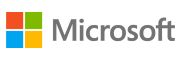