How to handle date and time in Python?
Handling date and time is a common requirement in programming, and Python offers robust tools to manage, manipulate, and format date and time values seamlessly.
- The `datetime` Module:
Central to Python’s date and time handling is the `datetime` module in the Python Standard Library. This module provides several classes to deal with dates, times, and intervals, including:
– `datetime.date`: Represents a date (year, month, day) without a time component.
– `datetime.time`: Represents a time of day, independent of any specific date.
– `datetime.datetime`: Combines both date and time information.
– `datetime.timedelta`: Represents the difference between two dates or times.
- Getting Current Date and Time:
The `datetime.datetime.now()` method returns the current date and time, allowing developers to capture timestamps for events or logs.
- Formatting Date and Time:
The `strftime` method allows for formatting date and time objects into readable strings, using various format codes to represent components like day, month, year, hour, and so forth. Conversely, the `strptime` method enables parsing strings into date and time objects based on a provided format.
- Time Zones:
Handling time zones can be tricky. The `pytz` library, although not part of the standard library, is an essential tool for managing date and time data across different time zones.
- The `dateutil` Library:
Another invaluable external library is `dateutil`. It simplifies many date operations, like parsing dates from strings without specifying a format, and handling relative deltas.
- The `time` Module:
Besides `datetime`, Python’s standard library also includes the `time` module, which is more oriented towards time-related functions. It provides functionalities like `sleep` (for introducing delays) and accessing Unix timestamps.
Python provides a comprehensive suite of tools for working with date and time. The `datetime` module in the standard library covers most common needs, while external libraries like `pytz` and `dateutil` offer additional, specialized functionalities. By leveraging these tools, developers can effectively manage and manipulate date and time data, ensuring accuracy and readability across diverse applications.
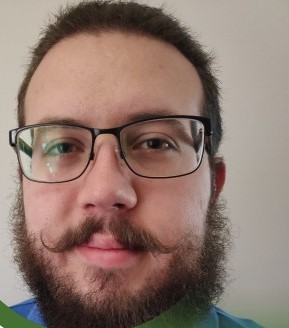
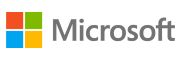