10 Python Libraries for Data Manipulation
In today’s data-driven world, effective data manipulation is a crucial skill for any data scientist, analyst, or researcher. Python, with its rich ecosystem of libraries, offers an array of tools that simplify data manipulation tasks and allow professionals to efficiently process, clean, and analyze data. In this article, we will explore 10 essential Python libraries for data manipulation, each serving a unique purpose in the data workflow.
Table of Contents
1. Pandas: Data Manipulation Powerhouse
1.1. Introduction to Pandas
Pandas is perhaps the most popular Python library for data manipulation and analysis. It introduces two primary data structures, Series and DataFrame, which allow users to store and manipulate data effectively.
1.2. Reading and Writing Data
python import pandas as pd # Reading a CSV file data = pd.read_csv('data.csv') # Writing to a CSV file data.to_csv('new_data.csv', index=False)
1.3. Data Selection and Filtering
python # Selecting specific columns selected_data = data[['column1', 'column2']] # Filtering data filtered_data = data[data['column1'] > 10]
1.4. Handling Missing Data
python # Checking for missing values missing_values = data.isnull().sum() # Dropping missing values cleaned_data = data.dropna() # Filling missing values filled_data = data.fillna(0)
1.5. Aggregation and Grouping
python # Grouping data and calculating mean grouped_data = data.groupby('category')['value'].mean() # Aggregating multiple statistics aggregated_data = data.groupby('category').agg({'value': ['mean', 'std']})
2. NumPy: Fundamental for Numerical Computations
2.1. Array Creation and Manipulation
NumPy provides a powerful array object that enables efficient numerical computations.
python import numpy as np # Creating an array arr = np.array([1, 2, 3, 4, 5]) # Array operations result = arr * 2
2.2. Mathematical Operations
python # Element-wise operations squared = np.square(arr) # Dot product of arrays dot_product = np.dot(arr, arr2)
2.3. Broadcasting
python # Broadcasting example broadcasted = arr + 10
2.4. Array Indexing and Slicing
python # Indexing and slicing value = arr[2] subset = arr[1:4]
3. Dask: Scalable Parallel Computing
3.1. Introduction to Dask
Dask is designed for parallel computing and out-of-core execution of larger-than-memory datasets.
3.2. Parallel Processing with Dask
python import dask.dataframe as dd # Load data using Dask DataFrame data = dd.read_csv('large_data.csv') # Parallel computation result = data['column1'].mean().compute()
3.3. Dask DataFrames and Arrays
python # Convert Dask DataFrame to Pandas DataFrame pandas_df = data.compute() # Dask Arrays for parallel numerical computations dask_array = da.from_array(np_array, chunks=(1000,))
3.4. Handling Larger-than-Memory Data
python # Process data larger than memory data = dd.read_csv('big_data.csv', blocksize=1e6)
4. SciPy: Scientific Computing
4.1. Introduction to SciPy
SciPy is built on top of NumPy and provides additional functionality for scientific computing tasks.
4.2. Mathematical Functions
python import scipy.constants as const # Calculate sine function sine_value = scipy.sin(45) # Physical constants speed_of_light = const.speed_of_light
4.3. Optimization and Integration
python from scipy.optimize import minimize from scipy.integrate import quad # Optimization result = minimize(objective_function, initial_guess) # Numerical integration integral, error = quad(function, lower_limit, upper_limit)
4.4. Statistical Functions
python from scipy.stats import norm # Probability density function pdf = norm.pdf(x, mean, std) # Statistical tests t_statistic, p_value = scipy.stats.ttest_ind(group1, group2)
5. Arrow: Efficient DateTime and Fixed-Size Data
5.1. Introduction to Arrow
Arrow is designed for handling efficient datetime and fixed-size data.
5.2. Working with DateTime
python import arrow # Current datetime now = arrow.now() # Formatting datetime formatted = now.format('YYYY-MM-DD HH:mm:ss')
5.3. Fixed-Size Data Management
python import pyarrow as pa # Create fixed-size data array data_array = pa.array([1, 2, 3, 4, 5], type=pa.int32())
6. Vaex: Out-of-Core DataFrames
6.1. Introduction to Vaex
Vaex is designed for lazy and out-of-core data processing, enabling analysis of large datasets.
6.2. Lazy and Out-of-Core DataFrames
python import vaex # Create a lazy DataFrame df = vaex.from_csv('large_data.csv') # Filtering and computing on the fly result = df[df['column1'] > 10]['column2'].mean()
6.3. Performance and Memory Efficiency
python # Memory-efficient aggregations agg_df = df.groupby('category').agg({'value': vaex.agg.mean('value')})
7. Petl: ETL Operations Simplified
7.1. Introduction to Petl
Petl is a library for simplifying ETL (Extract, Transform, Load) operations on data.
7.2. Data Transformation and Cleaning
python import petl as etl # Load data from CSV table = etl.fromcsv('data.csv') # Transformation cleaned_table = etl.cutout(table, 'column_to_remove')
7.3. ETL (Extract, Transform, Load) Operations
python # Joining tables joined_table = etl.join(table1, table2, key='common_column') # Loading data etl.todb(joined_table, 'database', 'table')
8. Fuzzywuzzy: String Matching and Text Similarity
8.1. Introduction to Fuzzywuzzy
Fuzzywuzzy is a library for approximate string matching and text similarity.
8.2. String Matching
python from fuzzywuzzy import fuzz # Compare string similarity similarity_ratio = fuzz.ratio('apple', 'apples')
8.3. Text Similarity Scoring
python from fuzzywuzzy import process # Get best match best_match = process.extractOne('pineapple', ['apple', 'banana', 'pear'])
9. Cudf: GPU-Accelerated DataFrames
9.1. Introduction to Cudf
Cudf is a GPU-accelerated library for data manipulation, compatible with Pandas.
9.2. GPU-Accelerated Data Manipulation
python import cudf # Create a GPU DataFrame gdf = cudf.DataFrame({'column1': [1, 2, 3, 4, 5]}) # Perform GPU-accelerated operations result = gdf['column1'].mean()
9.3. Performance Advantages
python # Compare CPU and GPU performance %timeit pandas_mean = pdf['column1'].mean() %timeit gpu_mean = gdf['column1'].mean()
10. Polars: Fast DataFrame Library
10.1. Introduction to Polars
Polars is designed for fast data manipulation and analysis, resembling Pandas and Dask.
10.2. DataFrame Operations
python import polars as pl # Create a Polars DataFrame df = pl.DataFrame({'column1': [1, 2, 3, 4, 5]}) # Filtering and aggregation filtered_df = df.filter(df['column1'] > 2) aggregated_df = df.groupby('category').agg(pl.col('value').mean())
10.3. Performance Features
python # Efficient string operations upper_case = df.with_column(df['name'].str.upper()) # Parallel execution result = df.lazy().filter(df['value'] > 10).collect()
Conclusion
In conclusion, these 10 Python libraries form a toolkit that empowers data professionals to efficiently manipulate and analyze data. From the versatile Pandas for general data manipulation to specialized libraries like Fuzzywuzzy for text similarity, these tools cater to a wide range of data manipulation needs. Whether you’re dealing with small datasets or large ones that require out-of-core processing, these libraries provide the necessary capabilities to streamline your data workflow and unlock valuable insights.
Table of Contents
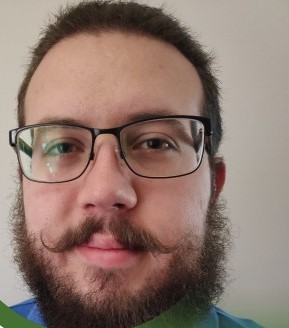
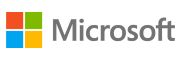