10 Python Libraries for Deep Learning
Deep learning has revolutionized the field of artificial intelligence by enabling computers to learn and make decisions from vast amounts of data. Python, with its user-friendly syntax and extensive library ecosystem, has become the go-to programming language for implementing deep learning projects. In this article, we’ll delve into the top 10 Python libraries that play a crucial role in building and deploying deep learning models.
Table of Contents
1. TensorFlow
Empowering Deep Learning at Scale
TensorFlow, developed by Google Brain, is arguably the most popular deep learning library. Its extensive capabilities include automatic differentiation, support for GPUs and TPUs, and a high-level API (Keras) for rapid prototyping. Here’s a simple example of creating a neural network using TensorFlow:
python import tensorflow as tf model = tf.keras.Sequential([ tf.keras.layers.Dense(128, activation='relu', input_shape=(784,)), tf.keras.layers.Dropout(0.2), tf.keras.layers.Dense(10, activation='softmax') ]) model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
2. PyTorch
Flexibility and Research-Oriented Approach
PyTorch, developed by Facebook’s AI Research lab (FAIR), is favored by researchers for its dynamic computation graph. It provides greater flexibility in model building and debugging. Here’s an example of creating a simple neural network in PyTorch:
python import torch import torch.nn as nn class Net(nn.Module): def __init__(self): super(Net, self).__init__() self.fc1 = nn.Linear(784, 128) self.fc2 = nn.Linear(128, 10) def forward(self, x): x = torch.relu(self.fc1(x)) x = self.fc2(x) return x model = Net()
3. Keras
High-Level Neural Networks API
Keras acts as an interface for TensorFlow and other deep learning libraries. It offers an intuitive way to design and train neural networks. Below is an example of building a simple convolutional neural network (CNN) using Keras:
python from keras.models import Sequential from keras.layers import Conv2D, MaxPooling2D, Flatten, Dense model = Sequential([ Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)), MaxPooling2D(pool_size=(2, 2)), Flatten(), Dense(10, activation='softmax') ])
4. CNTK (Microsoft Cognitive Toolkit)
Efficient and Scalable Deep Learning
Microsoft Cognitive Toolkit (CNTK) emphasizes efficiency and scalability. It’s known for supporting complex network architectures and large datasets. Here’s an example of building a deep neural network using CNTK:
python import cntk as C x = C.input_variable(784) y = C.layers.Dense(10, activation=None)(x)
5. Theano
Precursor to Modern Deep Learning Libraries
While Theano is no longer actively developed, it played a significant role in shaping the deep learning landscape. Here’s an example of creating a simple neural network using Theano:
python import theano import theano.tensor as T x = T.matrix('x') y = T.vector('y') W = theano.shared(np.random.randn(784, 128), name='W') b = theano.shared(np.zeros(128), name='b') output = T.nnet.relu(T.dot(x, W) + b)
6. Caffe
Deep Learning Framework for Image Classification
Caffe is a deep learning framework well-suited for image classification tasks. It’s known for its speed and memory efficiency. Below is an example of defining a simple neural network in Caffe:
python import caffe net = caffe.NetSpec() net.data, net.label = caffe.layers.Data(...) net.fc1 = caffe.layers.InnerProduct(...) net.softmax = caffe.layers.Softmax(...)
7. MXNet
Scalable Deep Learning for All Platforms
MXNet is designed for both efficiency and flexibility. It’s highly scalable and supports distributed computing. Here’s an example of creating a basic neural network using MXNet’s Gluon API:
python from mxnet import nd, gluon net = gluon.nn.Sequential() with net.name_scope(): net.add(gluon.nn.Dense(128, activation='relu')) net.add(gluon.nn.Dense(10))
8. Chainer
Dynamic and Intuitive Deep Learning Framework
Chainer’s dynamic computation graph allows for more intuitive model construction and debugging. It suits researchers and practitioners alike. Here’s an example of building a simple neural network in Chainer:
python import chainer import chainer.functions as F import chainer.links as L class Net(chainer.Chain): def __init__(self): super(Net, self).__init__() with self.init_scope(): self.fc1 = L.Linear(None, 128) self.fc2 = L.Linear(128, 10) def __call__(self, x): h = F.relu(self.fc1(x)) return self.fc2(h)
9. Fastai
Simplifying Deep Learning for Practitioners
Fastai is known for its simplicity and ease of use. It provides high-level abstractions for complex deep learning tasks. Here’s an example of using Fastai to create a simple image classification model:
python from fastai.vision.all import * dls = ImageDataLoaders.from_folder('data', train='train', valid='valid') learn = cnn_learner(dls, resnet18, metrics=accuracy) learn.fine_tune(4)
10. Gluon
Intuitive Interface for Neural Network Construction
Gluon, part of the MXNet ecosystem, offers a user-friendly interface for constructing deep learning models. It supports both symbolic and imperative programming styles. Here’s an example of using Gluon to build a neural network:
python from mxnet import gluon, nd net = gluon.nn.Sequential() with net.name_scope(): net.add(gluon.nn.Dense(128, activation='relu')) net.add(gluon.nn.Dense(10))
Conclusion
These 10 Python libraries for deep learning cater to different needs, from research to application development. Whether you’re building image classifiers, natural language processing models, or even experimenting with new architectures, these libraries provide a diverse toolbox to explore the fascinating world of deep learning. By combining these libraries with your creativity and domain expertise, you’ll be well-equipped to tackle complex AI challenges and contribute to the ever-evolving field of deep learning.
Table of Contents
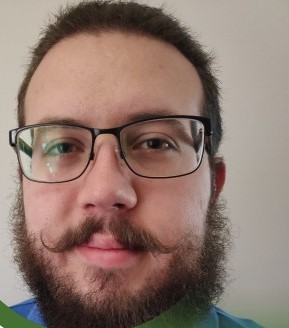
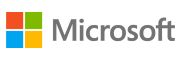