How to send emails through Python?
Sending emails through Python can be achieved using the `smtplib` and `email` modules available in the standard library. Here’s a succinct explanation:
- `smtplib` Module:
This module defines an `SMTP` class that you can use to connect to an SMTP (Simple Mail Transfer Protocol) mail server and send emails.
- `email` Module:
This helps to construct the email body, including multipart emails with attachments or HTML content.
To send an email using Python:
- Connect to the Server:
Initialize a connection to the SMTP server using `smtplib.SMTP(server_address, port)`. If using a provider like Gmail, you’d use `smtp.gmail.com` and port `587`.
- Login (If Required):
Some SMTP servers require authentication. You can log in using the `login` method provided by the `SMTP` class.
- Create the Email:
Use the `email` module to craft your message. You can set the “from”, “to”, subject, and body of the email.
- Send the Email:
Utilize the `sendmail` method of the `SMTP` class to dispatch the email.
- Close the Connection:
Terminate the connection using the `quit` method.
Example:
```python import smtplib from email.message import EmailMessage msg = EmailMessage() msg.set_content('Hello, this is a test email from Python!') msg['Subject'] = 'Test Email' msg['From'] = 'youremail@example.com' msg['To'] = 'recipient@example.com' with smtplib.SMTP('smtp.example.com', 587) as server: server.starttls() # Secure the connection server.login('youremail@example.com', 'password') server.send_message(msg) ```
A couple of things to note:
– If you’re using a provider like Gmail, you’ll need to allow “less secure apps” in your settings to send emails through scripts.
– Always be careful with your credentials. Avoid hardcoding them directly into your scripts; instead, consider using environment variables or secure vaults.
In real-world applications, there are libraries like `yagmail` that make the process even simpler, but the aforementioned method is native to Python’s standard library.
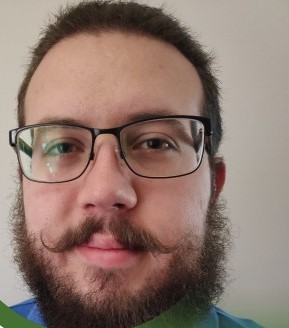
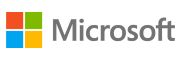