React Native Accessibility: Making Apps Inclusive for All Users
In today’s digital age, creating software that caters to a diverse audience is not just a nicety – it’s a necessity. As developers, it’s our responsibility to ensure that our apps are accessible to everyone, including users with disabilities. Accessibility isn’t an afterthought; it should be an integral part of the development process from the very beginning. In this blog post, we’ll explore the world of React Native accessibility and how you can make your apps more inclusive for all users.
Table of Contents
1. Understanding Accessibility in Mobile Apps
Accessibility, in the context of software development, refers to the practice of designing and coding apps in a way that allows people with disabilities to use them effectively. Disabilities can range from visual and auditory impairments to motor and cognitive limitations. Ensuring accessibility means removing barriers that might prevent individuals with disabilities from using your app with the same level of ease as those without disabilities.
In the realm of mobile app development, React Native has gained immense popularity due to its ability to create cross-platform apps with a single codebase. However, building accessible apps requires more than just knowing how to write React Native code; it requires a deeper understanding of accessibility principles and the tools React Native offers.
2. The Core Principles of Accessibility
2.1. Perceivable: Provide Alternative Ways to Consume Information
A visually impaired user should be able to understand the content of your app just like any other user. This means using proper text labels for images, providing captions for videos, and ensuring that all content is presented in a meaningful order that makes sense when read aloud by a screen reader.
jsx // Adding an accessible image with a text label <Image source={require('./image.png')} accessibilityLabel="A colorful sunset over the ocean" />
2.2. Operable: Make UI Elements Easily Navigable
Users might navigate your app using various input methods – touch, keyboard, or voice commands. Ensure that all interactive elements, such as buttons and input fields, are easily navigable and operable through different means.
jsx // Creating a touchable and keyboard-accessible button <TouchableOpacity onPress={handlePress} accessible={true} accessibilityRole="button" > <Text>Press Me</Text> </TouchableOpacity>
2.3. Understandable: Create Clear and Predictable UI
Your app’s user interface should be easy to understand and use. Avoid jargon, use consistent navigation patterns, and provide informative error messages. Users should be able to predict how the app will respond to their actions.
jsx // Giving a form field a clear label and providing error message <TextInput label="Email" placeholder="Enter your email" errorMessage="Please enter a valid email address." />
2.4. Robust: Build for Diverse Technologies
Apps should work across different devices, operating systems, and assistive technologies. Test your app on multiple platforms and devices to ensure a consistent and reliable experience.
3. Implementing Accessibility in React Native Apps
3.1. Use Semantic UI Components
React Native provides a set of accessible components that are designed with semantic meanings. For instance, instead of using a <View> element to create a button-like appearance, you should use the <Button> component.
jsx // Using the Button component for an accessible button <Button title="Click Me" onPress={handlePress} />
3.2. Provide Descriptive Labels for Images
When using images, always include an accessibilityLabel to provide a textual description of the image’s content. Screen readers can then convey this information to visually impaired users.
3.3. Enable Larger Touch Areas
To make it easier for users with motor difficulties to interact with your app, ensure that touchable elements have a sufficient touch area. You can use the Touchable components’ hitSlop prop to increase the touchable area without changing the visual layout.
jsx // Increasing the touchable area for a button <TouchableOpacity hitSlop={{ top: 10, bottom: 10, left: 10, right: 10 }}> <Text>Touch Area</Text> </TouchableOpacity>
3.4. Use AccessibilityRole and AccessibilityState
The accessibilityRole and accessibilityState props are crucial for conveying the purpose and state of UI elements to assistive technologies. For example, using accessibilityRole=”heading” and accessibilityState={{ level: 2 }} for a header text helps screen readers understand its significance.
jsx // Making a header accessible with appropriate role and state <Text accessibilityRole="heading" accessibilityState={{ level: 2 }}> Important Information </Text>
4. Testing and Verifying Accessibility
Ensuring your app is accessible requires rigorous testing. Here are some tools and techniques to assist you:
4.1. Use Accessibility Inspector
Both iOS and Android platforms offer accessibility inspection tools that allow you to explore how your app is presented to accessibility services. This enables you to identify issues and verify proper labeling of UI elements.
4.2. Manual Testing with Screen Readers
Engage with screen readers like VoiceOver (iOS) and TalkBack (Android) to experience how your app is perceived by users with visual impairments. Navigate through your app using only audio cues and gestures.
4.3. Automated Testing
Integrate automated accessibility testing libraries like react-native-testing-library and jest-axe into your testing suite. These tools can help catch accessibility issues during development.
Conclusion
Incorporating accessibility into your React Native app development process is not just about meeting compliance standards; it’s about creating a more inclusive digital world for everyone. By understanding the core principles of accessibility and implementing them using React Native’s features, you can ensure that your app caters to a broader range of users, regardless of their abilities. So, let’s embrace accessibility from the start and make technology truly accessible for all.
Table of Contents
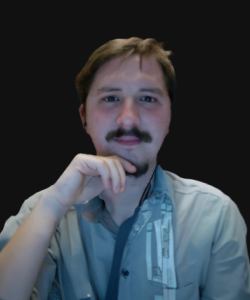
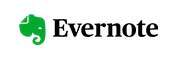