React Native and Amazon S3: Integrating Cloud Storage
In the realm of mobile app development, React Native has emerged as a powerful framework for building cross-platform applications. Its ability to streamline the development process while maintaining performance has made it a favorite among developers. However, as applications become more complex and data-intensive, the need for efficient cloud storage solutions becomes paramount. One such solution is Amazon S3, a scalable object storage service offered by Amazon Web Services (AWS). In this article, we’ll explore how to integrate Amazon S3 with React Native to enable seamless cloud storage functionality within your mobile applications.
Why Amazon S3?
Before diving into the integration process, let’s briefly discuss why Amazon S3 is a popular choice for cloud storage. Amazon S3 offers virtually unlimited storage capacity, high availability, and durability at a relatively low cost. Its simple and scalable architecture makes it suitable for a wide range of use cases, from hosting static website assets to storing large multimedia files.
Integration Steps
1. Set Up Amazon S3 Bucket
The first step is to create an Amazon S3 bucket to store your application’s data. Log in to the AWS Management Console, navigate to the S3 service, and create a new bucket. Configure the bucket settings according to your requirements, such as region and access permissions.
2. Install AWS SDK
Next, install the AWS SDK for JavaScript in your React Native project. You can do this using npm or yarn by running the following command:
npm install aws-sdk
This SDK provides a set of APIs for interacting with Amazon S3 services programmatically.
3. Configure AWS Credentials
To access your Amazon S3 bucket from your React Native application, you’ll need to configure AWS credentials. You can do this by creating an IAM user with appropriate permissions and generating access keys. Make sure to securely store these credentials and never hardcode them in your application code.
4. Implement Upload Functionality
With the AWS SDK installed and credentials configured, you can now implement upload functionality in your React Native app. This typically involves creating a form or interface for users to select files and initiate the upload process. Utilize the S3.putObject() method from the AWS SDK to upload files to your S3 bucket.
5. Handle Permissions and Security
Ensure that your Amazon S3 bucket is configured with the appropriate access control policies to restrict access and maintain data security. You can use IAM roles and policies to manage access permissions for different users and services interacting with your bucket.
Example Code
Here’s a simplified example demonstrating how to upload a file to Amazon S3 from a React Native app:
import { S3 } from 'aws-sdk'; const s3 = new S3({ region: 'your-bucket-region', credentials: { accessKeyId: 'your-access-key-id', secretAccessKey: 'your-secret-access-key', }, }); const uploadFile = async (fileUri, fileName) => { const file = { uri: fileUri, name: fileName, type: 'image/jpeg', }; const params = { Bucket: 'your-bucket-name', Key: fileName, Body: file, ACL: 'public-read', // Set appropriate access control }; try { const response = await s3.upload(params).promise(); console.log('Upload successful:', response.Location); } catch (error) { console.error('Upload failed:', error); } };
Conclusion
Integrating Amazon S3 with React Native can significantly enhance the functionality and scalability of your mobile applications. By leveraging the power of cloud storage, you can efficiently manage and access large volumes of data while providing a seamless user experience. With the steps outlined in this article, you’ll be well-equipped to incorporate Amazon S3 into your React Native projects and take your apps to the next level.
External Links:
Table of Contents
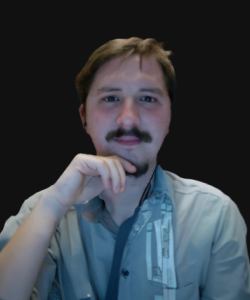
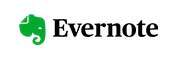