Animations in React Native: Bringing Life to Your Mobile App
In the fast-paced world of mobile app development, creating visually appealing and engaging user interfaces is key to capturing users’ attention and providing a memorable experience. This is where animations come into play. Animations have the power to add a touch of magic, making your app feel dynamic, responsive, and polished. React Native, a popular framework for building mobile apps using JavaScript, provides a range of tools and techniques to integrate stunning animations seamlessly. In this blog post, we’ll dive into the fascinating realm of animations in React Native and explore how you can breathe life into your mobile app.
1. Why Are Animations Important?
Before we delve into the technical aspects, let’s understand why animations hold such significance in mobile app development. Animations enhance user experience by making interactions feel more intuitive and natural. They provide visual cues that guide users through different actions, improving usability and reducing cognitive load. Animations also add personality and branding to your app, setting it apart from others and creating a memorable identity. Moreover, animations can convey information effectively, helping users understand changes in the interface, such as loading states, navigation transitions, or data updates. Overall, animations are a powerful tool to engage users, improve usability, and leave a lasting impression.
2. Animation Techniques in React Native
React Native offers various techniques to implement animations, catering to different use cases and preferences. Let’s explore some of the key animation techniques and how to use them effectively.
2.1. Animated API
The Animated API is a core component in React Native that allows you to create smooth animations through declarative syntax. It provides a way to interpolate animated values over time, controlling properties like opacity, position, and scale. Here’s a simple example of fading in an element using the Animated API:
javascript import React, { Component } from 'react'; import { View, Animated } from 'react-native'; class FadingExample extends Component { constructor(props) { super(props); this.state = { fadeAnim: new Animated.Value(0), // Initial value for opacity: 0 }; } componentDidMount() { Animated.timing( this.state.fadeAnim, { toValue: 1, duration: 1000, useNativeDriver: true, // Improve performance on native thread } ).start(); } render() { const { fadeAnim } = this.state; return ( <Animated.View style={{ opacity: fadeAnim, // Bind opacity to animated value }} > {/* Your content here */} </Animated.View> ); } } export default FadingExample;
2.2. Gesture-based Animations
Interactive gestures can take your app’s animations to the next level. React Native’s PanResponder provides a way to capture touch gestures and translate them into animations. This is especially useful for creating draggable elements, swiping actions, and other touch-based interactions. Below is a simplified example of creating a draggable element:
javascript import React, { Component } from 'react'; import { View, PanResponder, Animated } from 'react-native'; class DraggableElement extends Component { constructor(props) { super(props); this.state = { pan: new Animated.ValueXY(), }; this.panResponder = PanResponder.create({ onMoveShouldSetPanResponder: () => true, onPanResponderMove: Animated.event( [null, { dx: this.state.pan.x, dy: this.state.pan.y }], { useNativeDriver: false } ), onPanResponderRelease: () => { // Add your release logic here }, }); } render() { const { pan } = this.state; return ( <Animated.View style={{ transform: [{ translateX: pan.x }, { translateY: pan.y }], }} {...this.panResponder.panHandlers} > {/* Your draggable content here */} </Animated.View> ); } } export default DraggableElement;
2.3. Layout Animations
React Native’s LayoutAnimation API lets you create smooth transitions for layout changes, such as adding or removing components. It automatically animates the changes in position and size, providing a polished feel to your app. Here’s a basic example of using LayoutAnimation to animate the addition of a new element:
javascript import React, { Component } from 'react'; import { View, Text, TouchableOpacity, LayoutAnimation } from 'react-native'; class LayoutAnimationExample extends Component { constructor(props) { super(props); this.state = { showElement: false, }; } toggleElement = () => { LayoutAnimation.configureNext(LayoutAnimation.Presets.spring); this.setState({ showElement: !this.state.showElement }); }; render() { return ( <View> <TouchableOpacity onPress={this.toggleElement}> <Text>Show/Hide Element</Text> </TouchableOpacity> {this.state.showElement && <View style={{ height: 100, width: 100, backgroundColor: 'blue' }} />} </View> ); } } export default LayoutAnimationExample;
3. Best Practices for Effective Animations
While adding animations can greatly enhance your app, it’s essential to follow some best practices to ensure a smooth and delightful user experience:
3.1. Performance Considerations
Animations can impact app performance, especially on older devices. To mitigate this, use the useNativeDriver option whenever possible, which offloads the animation processing to the native thread, improving performance. Additionally, avoid overloading your app with too many animations running simultaneously, as this can lead to janky behavior.
3.2. Subtle and Intuitive Animations
The goal of animations is to enhance usability, not to distract users. Opt for subtle animations that provide context and feedback. For instance, animate button presses to acknowledge the action or use micro-interactions to guide users through the interface.
3.3. Consistency in Branding
Maintain consistency in your animation style to reinforce your app’s branding. Whether it’s the easing curve, animation speed, or visual elements, a consistent animation language contributes to a polished and professional feel.
3.4. User Feedback and Accessibility
Consider accessibility when designing animations. Provide alternative ways to convey information for users who may not perceive animations. Moreover, ensure that animations don’t hinder user understanding or impede the user’s ability to interact with the app.
Conclusion
In the world of mobile app development, animations are a potent tool for creating memorable, engaging, and user-friendly experiences. React Native offers a range of animation techniques, from the basic Animated API to gesture-based animations and layout transitions. By following best practices and using animations judiciously, you can bring your app to life and leave a lasting impact on your users. So, dive into the realm of animations, experiment with different techniques, and watch your app transform into an interactive work of art.
Remember, animations aren’t just embellishments; they’re the bridge between your app and its users, making interactions delightful and intuitive. Embrace the power of animations and let your creativity shine in the world of mobile app development.
Table of Contents
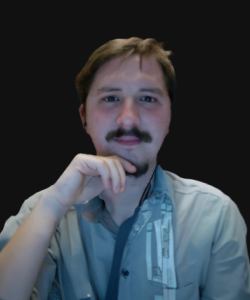
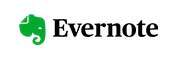