React Native and ARKit: Exploring Apple’s AR Framework
Augmented Reality (AR) has rapidly emerged as a game-changer in the world of mobile app development. With the ability to overlay digital content onto the real world, AR offers endless possibilities for creating immersive and interactive user experiences. Apple’s ARKit is a powerful framework that makes it easier than ever to integrate AR features into your iOS applications. In this blog, we’ll delve into the world of ARKit and discover how to leverage its capabilities with React Native, a popular JavaScript framework for building cross-platform mobile apps.
Table of Contents
1. What is ARKit?
Before we dive into the integration of ARKit with React Native, let’s start with a brief overview of what ARKit is and what it brings to the table.
2. Understanding ARKit
ARKit is Apple’s augmented reality framework introduced in iOS 11. It provides developers with a set of tools and APIs to create immersive AR experiences for iPhone and iPad devices. Some of the key features of ARKit include:
2.1. World Tracking
ARKit can track the device’s movement and orientation in the real world. It utilizes the device’s camera and motion sensors to create a virtual map of the environment, allowing digital objects to be placed accurately in the physical space.
2.2. Scene Understanding
ARKit can recognize and understand the geometry of the real world, such as walls, floors, and objects. This information enables developers to create AR experiences that interact with the physical environment.
2.3. 3D Object Detection
ARKit is capable of detecting and tracking 3D objects, making it possible to integrate virtual objects seamlessly into the real world. This feature is particularly useful for gaming and interactive applications.
2.4. Face Tracking
With ARKit’s face tracking capabilities, developers can create AR experiences that respond to facial expressions and movements. This is commonly used in applications like Animoji and face filters.
Now that we have a basic understanding of ARKit, let’s explore how we can integrate it with React Native to build AR-powered apps.
3. Setting Up the Development Environment
To get started with React Native and ARKit, you’ll need a Mac running macOS and Xcode installed. You’ll also need Node.js and npm (Node Package Manager) for managing React Native dependencies. Follow these steps to set up your development environment:
3.1. Installing Node.js and npm
If you don’t already have Node.js and npm installed, you can download and install them from the official Node.js website.
3.2. Installing React Native CLI
You’ll need the React Native command-line interface (CLI) to create and manage React Native projects. Install it globally using npm:
bash npm install -g react-native-cli
3.3. Creating a New React Native Project
Now, let’s create a new React Native project. Replace “MyARApp” with your preferred project name:
bash react-native init MyARApp
3.4. Adding ARKit Support
To use ARKit in your React Native project, you’ll need a third-party library called react-native-arkit. Install it using npm:
bash npm install react-native-arkit --save
With the development environment set up, you’re ready to start building AR experiences with React Native and ARKit.
4. Building Your First AR App
Let’s create a simple AR app that displays a 3D object in the real world using React Native and ARKit. Follow these steps to build your first AR app:
4.1. Importing ARKit
In your React Native project, open the main component file (usually App.js), and import the ARKit module at the top of the file:
javascript import ARKit from 'react-native-arkit';
4.2. Creating the AR Scene
Next, create a component that will serve as the AR scene. This component will contain the AR view and any AR objects you want to display. Here’s a simple example:
javascript import React, { Component } from 'react'; import { View } from 'react-native'; import ARKit from 'react-native-arkit'; class ARScene extends Component { render() { return ( <View style={{ flex: 1 }}> <ARKit style={{ flex: 1 }} debug planeDetection lightEstimation > {/* Add AR objects here */} </ARKit> </View> ); } } export default ARScene;
In this code, we’ve created an ARScene component that contains an ARKit element. We’ve enabled debug mode, plane detection, and light estimation, which are common AR features.
4.3. Adding 3D Objects
Now, let’s add a 3D object to the AR scene. You’ll need a 3D model in a format supported by ARKit, such as .scn or .dae. You can find free 3D models online or create your own.
4.3.1. Importing the 3D Model
In your project directory, create a folder named models and place your 3D model file (e.g., myObject.dae) inside it.
4.3.2. Loading the 3D Model
In your ARScene component, import the 3D model file and add it to the AR scene:
javascript import React, { Component } from 'react'; import { View } from 'react-native'; import ARKit from 'react-native-arkit'; class ARScene extends Component { render() { return ( <View style={{ flex: 1 }}> <ARKit style={{ flex: 1 }} debug planeDetection lightEstimation > <ARKit.Model position={{ x: 0, y: 0, z: -1 }} scale={0.02} model={{ file: 'models/myObject.dae', }} /> </ARKit> </View> ); } } export default ARScene;
In this code, we’ve added an ARKit.Model component inside the ARKit element. We’ve specified the position and scale of the model and provided the path to the 3D model file.
4.4. Running the AR App
Now that you’ve created your AR scene with a 3D object, you can run the app on your iOS device or simulator. Open your terminal and navigate to your project directory, then run the following command:
bash react-native run-ios
This command will build and launch your React Native app on an iOS simulator or connected device. You should see the AR scene with the 3D object displayed in the real world.
5. Enhancing Your AR App
Congratulations on building your first AR app with React Native and ARKit! However, the possibilities for enhancing your AR experiences are limitless. Here are some ideas for taking your AR app to the next level:
5.1. Interactivity
Add interactivity to your AR objects. You can allow users to tap or swipe on objects to trigger actions or animations.
javascript <ARKit.Model // ... onClick={() => { // Handle object click event }} />
5.2. Scene Detection
Use ARKit’s scene understanding capabilities to create experiences that react to the physical environment. For example, you can make virtual objects appear on tables or interact with real-world objects.
5.3. Face Tracking
Implement face tracking to create AR effects that respond to the user’s facial expressions and movements.
javascript <ARKit.Face // ... onUpdate={(anchor) => { // Handle face tracking data }} />
5.4. AR Navigation
Develop AR navigation apps that guide users through indoor spaces using augmented reality wayfinding.
5.5. Multiplayer AR
Create multiplayer AR games where users can interact and collaborate in the same AR environment.
Conclusion
React Native and ARKit offer a powerful combination for building immersive augmented reality experiences on iOS devices. With the integration of ARKit into your React Native projects, you can create apps that blend the digital and physical worlds in exciting and innovative ways. Whether you’re building games, educational apps, or productivity tools, ARKit opens up a world of possibilities for your mobile app development endeavors. So, dive in, experiment, and bring your AR app ideas to life!
In this blog post, we’ve covered the basics of setting up your development environment, creating a simple AR app, and exploring ways to enhance your AR experiences. Now, it’s your turn to explore the endless opportunities that ARKit and React Native offer. Happy coding, and may your AR adventures be extraordinary!
Table of Contents
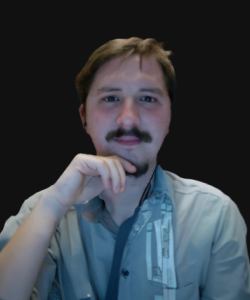
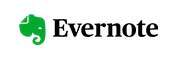