Securing React Native Apps: Implementing Authentication and Authorization
In the digital age, security is paramount, especially when it comes to mobile applications that handle sensitive user data. As the popularity of React Native continues to grow for developing cross-platform mobile apps, ensuring the security of these apps becomes a critical concern. One of the fundamental aspects of app security is implementing robust authentication and authorization mechanisms. In this article, we’ll delve into the essential steps, strategies, and best practices for securing your React Native apps.
Table of Contents
1. Why Authentication and Authorization Matter
Before we delve into the technical details, let’s understand why authentication and authorization are vital for the security of your React Native apps.
Authentication is the process of verifying the identity of users attempting to access your app. It ensures that only legitimate users can log in and use the app’s functionalities. Without proper authentication, unauthorized individuals could gain access to sensitive user information or perform actions they shouldn’t be able to.
Authorization, on the other hand, determines what actions authenticated users are allowed to perform within the app. It sets up access controls to ensure that users can only interact with the parts of the app that they are authorized to use. Proper authorization prevents users from accessing or modifying data beyond their permissions.
2. Implementing Authentication
2.1. User Registration and Login
The first step is to implement a user registration and login system. This involves creating screens for users to sign up with their credentials and log in with their registered accounts. Here’s a simplified code snippet using React Native elements:
jsx import React, { useState } from 'react'; import { View, TextInput, Button } from 'react-native'; const RegistrationScreen = () => { const [email, setEmail] = useState(''); const [password, setPassword] = useState(''); const handleRegistration = () => { // Perform user registration logic here }; return ( <View> <TextInput placeholder="Email" value={email} onChangeText={setEmail} /> <TextInput placeholder="Password" value={password} onChangeText={setPassword} secureTextEntry /> <Button title="Register" onPress={handleRegistration} /> </View> ); }; export default RegistrationScreen;
2.2. Secure Storage of Credentials
Storing user credentials securely is crucial to prevent unauthorized access to sensitive information. Utilize secure storage mechanisms such as React Native’s AsyncStorage or third-party libraries like react-native-keychain.
javascript import { AsyncStorage } from 'react-native'; // Storing credentials const storeCredentials = async (email, password) => { try { await AsyncStorage.setItem('email', email); await AsyncStorage.setItem('password', password); } catch (error) { console.log('Error storing credentials:', error); } }; // Retrieving credentials const getEmailAndPassword = async () => { try { const email = await AsyncStorage.getItem('email'); const password = await AsyncStorage.getItem('password'); return { email, password }; } catch (error) { console.log('Error retrieving credentials:', error); return null; } };
2.3. Token-based Authentication
Token-based authentication is a widely used method where users receive a token upon successful login. This token is included in the headers of subsequent requests to authenticate the user. The server validates the token and grants access if it’s valid.
javascript // Example of token-based authentication using Axios import axios from 'axios'; const login = async (email, password) => { try { const response = await axios.post('https://api.example.com/login', { email, password, }); const { token } = response.data; // Store the token securely return token; } catch (error) { console.log('Login error:', error); return null; } }; const fetchProtectedData = async (token) => { try { const response = await axios.get('https://api.example.com/data', { headers: { Authorization: `Bearer ${token}`, }, }); return response.data; } catch (error) { console.log('Data fetching error:', error); return null; } };
3. Implementing Authorization
3.1. Role-Based Authorization
Role-based authorization involves assigning different roles to users based on their privileges. For instance, you might have roles like “user,” “admin,” and “moderator.” Users with different roles can access different parts of the app.
javascript // Example of role-based authorization const userRoles = { USER: 'user', ADMIN: 'admin', MODERATOR: 'moderator', }; const checkUserRole = (userRole, requiredRole) => { return userRole === requiredRole; }; // In your component or route if (checkUserRole(currentUser.role, userRoles.ADMIN)) { // Render admin-specific content } else { // Render standard user content }
3.2. Protected Routes
In a React Native app, navigation is essential. Protect your routes to ensure that only authenticated users can access certain screens.
jsx import { NavigationContainer } from '@react-navigation/native'; import { createNativeStackNavigator } from '@react-navigation/native-stack'; const Stack = createNativeStackNavigator(); const AppNavigator = () => { return ( <NavigationContainer> <Stack.Navigator> {userAuthenticated ? ( <> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Profile" component={ProfileScreen} /> </> ) : ( <Stack.Screen name="Login" component={LoginScreen} /> )} </Stack.Navigator> </NavigationContainer> ); }; export default AppNavigator;
4. Best Practices for Security
- Use HTTPS: Always ensure that your app communicates over HTTPS to encrypt the data exchanged between the app and the server.
- Keep Libraries Updated: Regularly update your app’s dependencies, including React Native and third-party libraries, to patch security vulnerabilities.
- Sanitize Inputs: Prevent common security issues like SQL injection and cross-site scripting (XSS) by sanitizing and validating user inputs.
- Implement Two-Factor Authentication (2FA): For an extra layer of security, consider implementing 2FA, which requires users to provide a second form of verification.
- Regular Security Audits: Conduct regular security audits and penetration testing to identify and address potential vulnerabilities.
- Minimal User Data: Only collect and store the data that is necessary for your app’s functionality. Minimizing stored data reduces the potential impact of a data breach.
- Error Handling: Provide informative error messages to users without revealing sensitive information that could be exploited by attackers.
Conclusion
Securing React Native apps goes beyond just building beautiful and functional interfaces. It requires careful consideration of authentication and authorization mechanisms to protect user data and maintain the integrity of your app. By implementing robust authentication and authorization strategies and adhering to best practices, you can create a secure environment for your users and establish trust in your app’s capabilities. Remember, app security is an ongoing process, so stay updated with the latest security trends and continuously improve your app’s defenses against potential threats.
Table of Contents
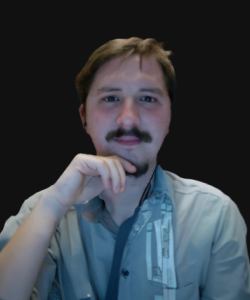
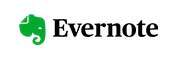