React Native and Background Tasks: Running Tasks in the Background
In the fast-paced world of mobile app development, React Native has emerged as a powerful tool for building cross-platform applications with ease. However, as app functionality becomes increasingly complex, developers often encounter the need to perform tasks in the background while maintaining a smooth user experience. In this article, we’ll delve into the world of background tasks in React Native, exploring techniques and best practices for running tasks in the background seamlessly.
Understanding Background Tasks in React Native
Background tasks refer to processes that continue to execute even when the application is not in the foreground. Common use cases for background tasks include fetching data from remote servers, syncing app data, and performing periodic updates or notifications.
In React Native, background tasks are typically handled using libraries or native modules that provide APIs for executing tasks in the background. These tasks can range from simple asynchronous operations to more complex operations requiring access to device-specific features.
Techniques for Running Background Tasks
1. Using AsyncStorage for Simple Background Operations
One straightforward approach for running background tasks in React Native involves utilizing AsyncStorage, a built-in asynchronous storage system. By storing data asynchronously, developers can perform lightweight background operations without impacting the app’s performance.
Example:
import AsyncStorage from '@react-native-async-storage/async-storage'; // Storing data in the background const storeDataInBackground = async (key, value) => { await AsyncStorage.setItem(key, value); }; // Retrieving data in the background const retrieveDataInBackground = async (key) => { const value = await AsyncStorage.getItem(key); return value; };
Learn more: AsyncStorage Documentation
2. Using Native Modules for Complex Background Tasks
For more advanced background operations requiring access to native APIs, developers can leverage native modules in React Native. Native modules allow seamless integration with device-specific features, enabling tasks such as geolocation tracking, sensor data collection, or media processing in the background.
Example:
import { NativeModules } from 'react-native'; const { BackgroundTaskModule } = NativeModules; // Initiating a background task BackgroundTaskModule.startBackgroundTask();
Learn more: React Native Native Modules Documentation
3. Using Third-Party Libraries for Task Scheduling
Several third-party libraries offer comprehensive solutions for managing background tasks in React Native. These libraries often provide features such as task scheduling, retry mechanisms, and error handling, simplifying the implementation of background operations in complex applications.
Example:
import BackgroundTimer from 'react-native-background-timer'; // Scheduling a background task BackgroundTimer.runBackgroundTimer(() => { // Perform background operation }, interval);
Learn more: React Native Background Timer Library
Best Practices for Background Tasks
- Keep Tasks Lightweight: Ensure that background tasks are lightweight and do not consume excessive resources, as this can impact the device’s performance and battery life.
- Handle Errors Gracefully: Implement error handling mechanisms to gracefully handle failures during background task execution and provide appropriate feedback to users.
- Optimize Battery Usage: Minimize the frequency of background task execution and optimize resource usage to reduce battery consumption and improve overall device efficiency.
- Test Across Platforms: Test background tasks across various platforms and device configurations to ensure consistent behavior and performance in real-world scenarios.
Conclusion
In conclusion, background tasks play a crucial role in enhancing the functionality and user experience of React Native applications. By leveraging the techniques and best practices outlined in this article, developers can effectively implement background tasks to perform a wide range of operations while maintaining optimal performance and usability.
Remember, whether you’re storing data asynchronously with AsyncStorage, integrating native modules for complex tasks, or utilizing third-party libraries for task scheduling, thoughtful implementation and adherence to best practices are key to success in running tasks in the background in React Native.
Table of Contents
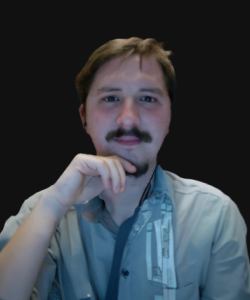
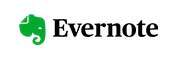