Implementing Biometric Authentication in React Native Apps
In the ever-evolving landscape of mobile app development, security remains a top concern for both users and developers. Traditional username and password authentication systems are prone to vulnerabilities and often result in poor user experiences due to the hassle of remembering complex passwords. To address these challenges, biometric authentication has emerged as a powerful solution. In this blog post, we will explore how to implement biometric authentication in React Native apps, providing users with a secure and seamless way to access your application.
Table of Contents
1. Why Choose Biometric Authentication?
Before delving into the technical aspects of implementing biometric authentication in React Native, let’s understand why this feature is worth incorporating into your app:
1.1. Enhanced Security
Biometric authentication methods, such as fingerprint recognition and facial recognition, offer a higher level of security compared to traditional password-based systems. This is because biometric data is unique to each individual, making it extremely difficult for unauthorized users to gain access.
1.2. Improved User Experience
Users appreciate the convenience of biometric authentication. It eliminates the need to remember and enter passwords, enhancing the overall user experience and reducing friction during login.
1.3. Compliance with Modern Standards
Many modern mobile devices come equipped with biometric sensors, making it a standard feature that users expect in applications. Implementing biometric authentication ensures your app is in line with current industry trends and user expectations.
Now that we understand the advantages of biometric authentication, let’s dive into the implementation process for React Native apps.
2. Setting Up Your React Native Project
To get started, make sure you have React Native installed on your system. If not, you can follow the official React Native documentation for installation instructions.
2.1. Create a New React Native Project
Open your terminal and run the following command to create a new React Native project:
bash npx react-native init BiometricAuthDemo
This command will create a new React Native project named “BiometricAuthDemo.”
2.2. Install Required Dependencies
Navigate to your project directory and install the necessary dependencies:
bash cd BiometricAuthDemo npm install @react-native-biometrics/react-native-biometrics
The @react-native-biometrics/react-native-biometrics package provides a convenient way to integrate biometric authentication into your React Native app.
3. Implementing Biometric Authentication
With your project set up and dependencies installed, it’s time to implement biometric authentication.
3.1. Import the Biometrics Module
In your React Native JavaScript code, import the biometrics module as follows:
javascript import { NativeModules } from 'react-native'; const { Biometrics } = NativeModules;
This module will allow you to interact with the biometric authentication APIs provided by the device.
3.2. Check Biometric Availability
Before enabling biometric authentication, you should check if the user’s device supports it. Use the isSensorAvailable() method to determine the availability of biometric sensors on the device:
javascript Biometrics.isSensorAvailable() .then((result) => { if (result === 'TouchID' || result === 'FaceID' || result === 'Biometrics') { // Biometric authentication is available on this device. } else { // Biometric authentication is not available. } }) .catch((error) => { console.error(error); });
This code snippet checks for the availability of Touch ID, Face ID, or generic biometric authentication on the device. If any of these options are available, you can proceed with implementing biometric authentication.
3.3. Authenticate with Biometrics
To authenticate the user with biometrics, you can use the authenticate() method. Here’s an example of how to use it:
javascript const promptMessage = 'Authenticate with your fingerprint or face'; Biometrics.authenticate(promptMessage) .then((result) => { if (result.success) { // Authentication successful, grant access to the app. } else { // Authentication failed or was canceled by the user. } }) .catch((error) => { console.error(error); });
In this code, we display a prompt message to the user, asking them to authenticate with their fingerprint or face. If the authentication is successful, you can grant access to the app. If it fails or the user cancels the operation, you can handle that accordingly.
3.4. Error Handling and Edge Cases
Biometric authentication may fail for various reasons, such as incorrect biometric data or a device error. It’s essential to implement proper error handling and provide clear feedback to the user.
Here’s an example of handling different authentication results:
javascript Biometrics.authenticate(promptMessage) .then((result) => { if (result.success) { // Authentication successful, grant access to the app. } else { switch (result.error) { case 'UserCanceled': // Authentication was canceled by the user. break; case 'BiometryNotEnrolled': // Biometric authentication is not set up on the device. break; case 'BiometryLockedOut': // Biometric authentication is locked due to too many failed attempts. break; default: // Other errors. console.error(result.error); } } }) .catch((error) => { console.error(error); });
By handling different error cases, you can provide a more user-friendly experience and guide users on how to resolve authentication issues.
4. Enhancing Security
While biometric authentication offers a high level of security, it’s essential to follow best practices to ensure the safety of user data:
4.1. Use Secure Storage
Store sensitive user data, such as tokens or authentication states, securely. React Native provides libraries like react-native-keychain and react-native-secure-key-store for this purpose.
4.2. Implement Session Management
Implement session management to control access to your app after successful biometric authentication. Ensure that sessions expire after a certain period of inactivity.
4.3. Regularly Update Dependencies
Keep your project’s dependencies, including the biometrics library, up to date to address security vulnerabilities and compatibility issues.
Conclusion
Incorporating biometric authentication into your React Native app is a proactive step towards enhancing security and improving the user experience. Users appreciate the convenience of biometrics, and it aligns your app with modern industry standards.
By following the steps outlined in this guide, you can seamlessly integrate biometric authentication, making your app more secure and user-friendly. Remember to handle errors gracefully and follow security best practices to ensure the protection of user data.
Biometric authentication is a powerful tool, and when implemented correctly, it can contribute significantly to your app’s success in the competitive mobile app market.
Start enhancing your React Native app’s security and user experience today by implementing biometric authentication! Your users will thank you for it.
Now that you have the knowledge and code samples to get started, take the next step in securing your React Native app with biometrics. Happy coding!
Table of Contents
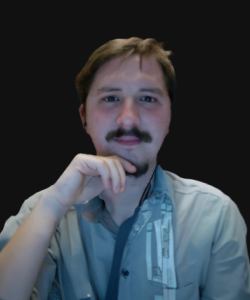
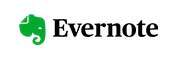