React Native and Bluetooth Beacons: Proximity-Based App Features
In the world of mobile app development, providing users with contextual and location-based experiences has become increasingly important. React Native, a popular framework for building cross-platform mobile apps, provides a powerful toolset for creating such experiences. When combined with Bluetooth beacons, you can unlock a new realm of possibilities for proximity-based app features.
Table of Contents
This blog post will guide you through the integration of Bluetooth beacons with React Native, showcasing how you can leverage this technology to enhance your app with proximity-based features. We’ll cover the basics of Bluetooth beacons, explore the advantages they offer, and provide step-by-step instructions with code samples to get you started.
1. Understanding Bluetooth Beacons
1.1. What Are Bluetooth Beacons?
Bluetooth beacons are small, battery-powered devices that transmit data packets at regular intervals. These packets contain information such as a unique identifier, signal strength, and other relevant data. Mobile devices, equipped with Bluetooth capabilities, can detect and interpret these signals, allowing for proximity-based interactions.
1.2. Types of Bluetooth Beacons
There are different types of Bluetooth beacons available, each with its own use cases:
- iBeacon: Developed by Apple, iBeacon is one of the most well-known beacon protocols. It’s widely used for indoor positioning and location-based notifications.
- Eddystone: An open-source beacon protocol developed by Google, Eddystone is versatile and supports various frame types, including URL, UID, and TLM (telemetry).
- AltBeacon: AltBeacon is an open-source alternative to iBeacon, designed to offer a more flexible and customizable solution.
1.3. Advantages of Using Bluetooth Beacons
Bluetooth beacons offer several advantages that make them suitable for proximity-based app features:
- Precision: Beacons provide precise location data, making them ideal for applications where accuracy is crucial.
- Low Power Consumption: Beacons are energy-efficient and can operate for months or even years on a single battery.
- Compatibility: Most modern smartphones are equipped with Bluetooth, making them compatible with beacon technology.
- Versatility: Bluetooth beacons can be used in various industries, including retail, healthcare, and tourism.
2. Setting Up Your React Native Project
Before diving into the integration of Bluetooth beacons, ensure you have a React Native project up and running. If you don’t have one yet, follow these steps:
- Install Node.js: Ensure you have Node.js installed on your machine. You can download it from the official website.
- Install Expo CLI: Expo is a framework for building React Native applications. Install it globally by running the following command:
shell npm install -g expo-cli
3. Create a New React Native Project: Create a new React Native project using Expo by running the following command:
shell expo init MyProximityApp
4. Follow the prompts to set up your project, and choose a blank template.
5. Navigate to Your Project Directory: Change your current directory to your project folder:
shell cd MyProximityApp
6. Start the Development Server: Start the development server using Expo CLI:
shell expo start
This will open the Expo Developer Tools in your default web browser. You can run your app on an emulator or a physical device by following the instructions provided.
3. Integrating Bluetooth Beacons into React Native
Now that you have your React Native project set up, it’s time to integrate Bluetooth beacons. For this example, we’ll use the react-native-beacons-manager library, which simplifies working with beacons in React Native.
3.1. Installing the react-native-beacons-manager Library
To begin, install the react-native-beacons-manager library in your project. Open a new terminal window, navigate to your project directory, and run the following command:
shell npm install react-native-beacons-manager --save
3.2. Linking the Library
React Native modules often need to be linked to your project. Fortunately, Expo handles this automatically for managed projects. For bare React Native projects, you may need to link the module manually using react-native link.
3.3. Permissions and Configuration
Before you can detect and interact with Bluetooth beacons, you need to request the necessary permissions from the user. In the case of iOS, you’ll need to edit the Info.plist file, while for Android, you’ll need to make changes to the AndroidManifest.xml file.
3.3.1. iOS Configuration
Add the following keys to your Info.plist file to request the necessary permissions:
xml <key>NSLocationWhenInUseUsageDescription</key> <string>We need your location to detect nearby beacons.</string> <key>NSLocationAlwaysUsageDescription</key> <string>We need your location to detect nearby beacons.</string> <key>NSLocationAlwaysAndWhenInUseUsageDescription</key> <string>We need your location to detect nearby beacons.</string>
3.3.2. Android Configuration
Add the following permissions and features to your AndroidManifest.xml file:
xml <uses-permission android:name="android.permission.BLUETOOTH"/> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN"/> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION"/> <uses-feature android:name="android.hardware.bluetooth_le" android:required="true"/>
4. Beacon Scanning
With the library installed and permissions configured, you can now start scanning for Bluetooth beacons. Here’s a basic example of how to do this:
javascript import Beacons from 'react-native-beacons-manager'; // Initialize the library Beacons.detectIBeacons(); // Start scanning for beacons Beacons.startRangingBeaconsInRegion('MyRegion'); // Listen for beacon data Beacons .BluetoothBeaconsDidRange .addListener(data => { console.log('Detected beacons:', data.beacons); });
In the code above, we first import the react-native-beacons-manager library and initialize it to detect iBeacons. We then start ranging for beacons in a specific region, in this case, ‘MyRegion’. Finally, we set up a listener to capture beacon data when they are detected.
5. Using Beacon Data in Your App
Now that you’re receiving beacon data, let’s explore some practical use cases for proximity-based app features.
5.1. Location-Based Notifications
Imagine you’re building an event app for a museum. You can use Bluetooth beacons to trigger location-based notifications when visitors approach specific exhibits. Here’s how you can implement this feature:
javascript // Inside your beacon data listener Beacons .BluetoothBeaconsDidRange .addListener(data => { const detectedBeacons = data.beacons; // Check if a specific beacon is nearby const targetBeacon = detectedBeacons.find(beacon => beacon.uuid === 'your-beacon-uuid'); if (targetBeacon && targetBeacon.distance < 1.0) { // Beacon is nearby, show a notification // You can use a library like 'react-native-push-notification' for this // Example: sendNotification("You're near Exhibit A!"); } });
In this code, we check if a specific beacon (identified by its UUID) is nearby and within a certain distance (e.g., 1 meter). If the conditions are met, we can trigger a notification to inform the user about the nearby exhibit.
5.2. Indoor Navigation
Bluetooth beacons can also be used for indoor navigation. If you’re developing an app for a large shopping mall or an airport, you can provide users with turn-by-turn directions to their destination.
javascript // Inside your beacon data listener Beacons .BluetoothBeaconsDidRange .addListener(data => { const detectedBeacons = data.beacons; // Calculate the user's location based on nearby beacons const userLocation = calculateUserLocation(detectedBeacons); // Update the user's position on the map updateMapWithUserLocation(userLocation); });
In this example, we use the detected beacons to estimate the user’s location within the indoor space. You can then update the map display to show the user’s position and provide navigation instructions.
5.3. Proximity Marketing
Retailers can benefit from proximity marketing using Bluetooth beacons. By sending targeted offers or discounts to shoppers when they approach certain sections of a store, you can enhance the shopping experience and increase sales.
javascript // Inside your beacon data listener Beacons .BluetoothBeaconsDidRange .addListener(data => { const detectedBeacons = data.beacons; // Check if the user is near a beacon associated with a promotion const nearbyPromotion = detectedBeacons.find(beacon => beacon.uuid === 'promotion-beacon-uuid'); if (nearbyPromotion && nearbyPromotion.distance < 2.0) { // Beacon with a promotion is nearby, show a special offer // Example: displayPromotion("20% off all shoes!"); } });
In this code, we check if the user is near a beacon associated with a promotion and display the corresponding offer when they are in close proximity.
Conclusion
Integrating Bluetooth beacons with React Native can open up a world of possibilities for proximity-based app features. Whether you’re building a location-aware event app, providing indoor navigation, or implementing proximity marketing, Bluetooth beacons offer a powerful tool for enhancing the user experience.
In this guide, we covered the basics of Bluetooth beacons, explained their advantages, and walked you through the process of setting up a React Native project, integrating the react-native-beacons-manager library, and implementing practical use cases for proximity-based features.
Now, armed with this knowledge, you can explore creative ways to leverage Bluetooth beacons and React Native to create innovative and context-aware mobile applications. Start experimenting, and you’ll be on your way to building the next generation of proximity-based apps.
Table of Contents
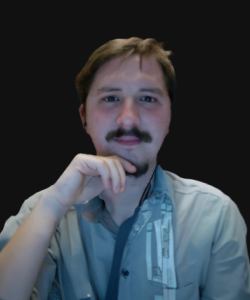
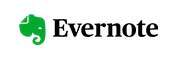