Building Facial Recognition in React Native Apps
In today’s digital landscape, integrating facial recognition technology into mobile applications has become increasingly prevalent. Whether it’s for enhancing security measures, improving user experience, or adding innovative features, facial recognition can offer a multitude of benefits. If you’re a developer diving into the world of React Native, incorporating facial recognition capabilities into your app can seem daunting at first. However, with the right tools and guidance, you can seamlessly integrate this advanced functionality into your React Native projects.
Understanding Facial Recognition Technology
Facial recognition technology works by analyzing and identifying unique facial features from an image or video frame. It involves several key steps, including face detection, feature extraction, and matching against existing templates or databases. Thanks to advancements in machine learning and computer vision algorithms, facial recognition has become highly accurate and efficient, making it an attractive choice for various applications.
Getting Started with React Native
Before delving into facial recognition implementation, ensure you have a basic understanding of React Native development. If you’re new to React Native, I recommend starting with the official documentation and tutorials provided by React Native’s development team. Familiarize yourself with the fundamentals of building React Native apps, including components, state management, and navigation.
Integrating Facial Recognition Libraries
To incorporate facial recognition functionality into your React Native app, you’ll need to leverage specialized libraries and tools. One popular choice is the React Native Camera package, which provides robust camera access and enables capturing images or video frames for facial analysis. Additionally, you can utilize third-party facial recognition libraries such as Microsoft Azure Face API or Amazon Rekognition for advanced facial detection and recognition capabilities.
Example: Implementing Facial Detection with React Native Camera
Let’s walk through a simple example of integrating facial detection using the React Native Camera package:
1. Install the React Native Camera package using npm or yarn:
npm install react-native-camera
2. Import the necessary components in your React Native app:
import { RNCamera } from 'react-native-camera';
3. Set up the camera component in your app’s render method:
<RNCamera style={styles.camera} type={RNCamera.Constants.Type.front} captureAudio={false} ref={ref => { this.camera = ref; }} />
4. Implement facial detection logic using the camera’s onFacesDetected event:
<RNCamera ... onFacesDetected={faces => { if (faces.faces.length > 0) { // Facial detection logic here } }} />
5. Customize the facial detection logic to suit your application’s requirements, such as displaying bounding boxes around detected faces or triggering actions based on detected emotions.
External Resources:
Conclusion
By following this guide and leveraging the power of React Native along with specialized facial recognition libraries, you can elevate your mobile app development projects to new heights. Whether you’re building a security-focused application, enhancing user experience, or exploring innovative features, integrating facial recognition can open up a world of possibilities for your React Native apps. Experiment with different libraries, explore advanced functionalities, and stay updated with the latest developments in facial recognition technology to create cutting-edge mobile experiences that captivate and delight users.
Start your journey into the exciting realm of facial recognition in React Native today, and unlock the full potential of your mobile app projects. Happy coding!
Table of Contents
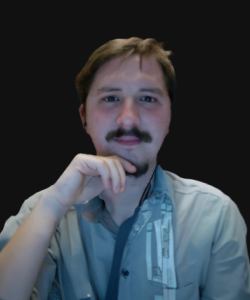
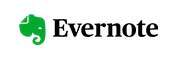