Building Offline-First Apps with React Native and Redux Persist
In today’s fast-paced digital world, mobile applications have become an integral part of our lives. However, a significant challenge that app developers face is ensuring a smooth user experience even when the user is offline or has intermittent network connectivity. Imagine a scenario where your app loses all the data the moment it goes offline or crashes – not a pleasant thought for users. This is where building offline-first apps becomes crucial, and React Native along with Redux Persist provides a powerful combination to achieve this goal. In this blog post, we’ll delve into the world of offline-first apps and explore how to build them using React Native and Redux Persist.
1. Understanding Offline-First Apps
1.1 Why Offline-First?
Offline-first apps are designed to function seamlessly even when the device has limited or no network connectivity. They prioritize delivering core features and content to users, regardless of whether they are connected to the internet. This approach is especially important because network outages, slow connections, or areas with poor reception are common occurrences. By prioritizing offline functionality, you provide users with a consistent and reliable experience, increasing user satisfaction and engagement.
1.2 Benefits of Offline-First Apps
Building offline-first apps using React Native and Redux Persist offers several advantages:
- Enhanced User Experience: Users can continue using the app without interruption, leading to higher user retention and satisfaction.
- Reduced Data Usage: Offline-first apps use locally cached data, reducing the need for continuous data fetching and minimizing data usage.
- Faster Performance: By relying on cached data, app performance improves as loading times decrease.
- Increased Availability: Users can access important features regardless of network availability, broadening your app’s user base.
2. Introduction to React Native and Redux Persist
2.1 What is React Native?
React Native is an open-source framework that allows developers to build cross-platform mobile applications using JavaScript and React. It enables you to create native-like user interfaces and experiences for both iOS and Android platforms, while still maintaining a single codebase. React Native’s component-based architecture and hot-reloading capabilities expedite development, making it an excellent choice for building offline-first apps.
2.2 What is Redux Persist?
Redux Persist is a library that works seamlessly with Redux to enable persistent storage of the application state. It allows you to save the Redux store’s state to a storage engine, such as AsyncStorage in React Native. This means that even if the app is closed or the device is restarted, the stored state can be rehydrated when the app is reopened, ensuring continuity and a consistent experience.
2.3 Why Combine React Native and Redux Persist?
The combination of React Native and Redux Persist provides a powerful toolkit for building offline-first apps. React Native simplifies the process of creating cross-platform apps with native-like performance, while Redux Persist ensures that the app’s state is retained across sessions, even when offline. This combination enables developers to create apps that gracefully handle offline scenarios and seamlessly sync data when connectivity is restored.
3. Setting Up Your React Native Project
3.1 Installing Required Dependencies
To get started, make sure you have Node.js and npm (Node Package Manager) installed on your system. Create a new React Native project using the following command:
bash npx react-native init OfflineFirstApp
Navigate to your project directory:
bash cd OfflineFirstApp
Now, install Redux and Redux Persist dependencies:
bash npm install redux react-redux redux-persist
3.2 Initializing Your Redux Store
In your project, create a folder named store to store your Redux-related files. Inside the store folder, create a file named index.js to set up your Redux store:
jsx import { createStore } from 'redux'; import { persistStore, persistReducer } from 'redux-persist'; import AsyncStorage from '@react-native-async-storage/async-storage'; // Use AsyncStorage for React Native // Import your reducers here import rootReducer from '../reducers'; const persistConfig = { key: 'root', storage: AsyncStorage, }; const persistedReducer = persistReducer(persistConfig, rootReducer); export const store = createStore(persistedReducer); export const persistor = persistStore(store);
4. Implementing Offline-First Functionality
4.1 Handling Network Status
To build an offline-first app, start by detecting the network status. The NetInfo module from React Native helps you monitor network connectivity. Here’s how you can use it:
jsx import NetInfo from '@react-native-community/netinfo'; // ... // Add this to your component or Redux action NetInfo.addEventListener(state => { if (state.isConnected) { // Handle data synchronization } else { // Handle offline mode } });
4.2 Using Redux Persist for Persistent Storage
Integrating Redux Persist into your app is straightforward. You’ve already set up the store with Redux Persist in the previous step. Now you can use it in your components:
jsx import { PersistGate } from 'redux-persist/integration/react'; // ... // Wrap your app with the PersistGate component const App = () => { return ( <Provider store={store}> <PersistGate loading={null} persistor={persistor}> {/* Your app components */} </PersistGate> </Provider> ); };
4.3 Dealing with Data Sync
When the app is back online, you’ll want to sync any locally cached data with the server. Implementing data synchronization can vary based on your backend architecture, but the general idea is to track changes while offline and push them to the server when connectivity is reestablished.
5. Optimizing User Experience
5.1 Displaying Cached Data
When the app is offline, you can display cached data to users instead of leaving the screen blank. This requires handling asynchronous data loading and rendering it when the app is offline:
jsx import { useSelector } from 'react-redux'; // ... // Inside your component const OfflineScreen = () => { const cachedData = useSelector(state => state.cachedData); return ( <View> {/* Display your cached data */} </View> ); };
5.2 Providing Offline Feedback
Keep users informed about their network status. You can provide visual feedback, such as displaying an offline banner or changing the app’s color scheme to indicate offline mode.
5.3 Syncing Data in the Background
To ensure a seamless user experience, consider implementing background data synchronization. When the app is online, periodically check for new data or changes and update the cached state accordingly. Tools like React Native’s BackgroundFetch can be helpful for this purpose.
6. Testing Your Offline-First App
6.1 Emulating Offline Scenarios
During development, it’s essential to test your app’s behavior in offline scenarios. You can simulate offline mode in the Android Emulator or iOS Simulator by toggling airplane mode or disconnecting the network connection.
6.2 Unit Testing Redux Persist Integration
Write unit tests to ensure that Redux Persist is working as expected. Test scenarios where the app is closed and reopened, verifying that the state is correctly rehydrated from storage.
Conclusion
Building offline-first apps with React Native and Redux Persist is a smart approach to providing a seamless user experience in today’s unpredictable network conditions. By prioritizing offline functionality, you create apps that remain functional even when users are offline, leading to higher engagement, user satisfaction, and a broader user base. Combining the power of React Native’s cross-platform capabilities with Redux Persist’s state persistence, you can confidently develop apps that conquer the challenges of an ever-connected world. So, dive into the world of offline-first development, and create apps that keep your users engaged, regardless of their network status.
Table of Contents
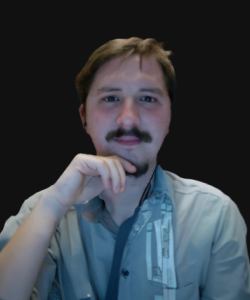
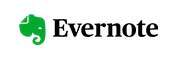