Best Practices for Building Scalable React Native Apps
React Native has gained immense popularity as a versatile framework for building cross-platform mobile applications. However, with the increasing complexity of apps and the growing user expectations for seamless experiences, scalability becomes a critical concern. Building scalable React Native apps requires careful planning, optimized coding practices, and a focus on performance. In this article, we’ll explore some of the best practices to ensure your React Native app can handle growth and maintain a high level of performance.
1. Component Modularization
1.1 Breaking Down into Smaller Components
One of the fundamental principles of building scalable React Native apps is to break down your UI into smaller, reusable components. This not only makes your codebase more organized but also allows for easier maintenance and updates. Instead of creating monolithic components, aim to build components that have a single responsibility and can be combined to create more complex UI elements.
jsx // Example of a reusable Button component import React from 'react'; import { TouchableOpacity, Text } from 'react-native'; const Button = ({ title, onPress }) => ( <TouchableOpacity onPress={onPress}> <Text>{title}</Text> </TouchableOpacity> ); export default Button;
1.2 Leveraging Container and Presentational Components
To further enhance the modularity of your React Native app, consider using the container and presentational component pattern. Container components handle the logic and state management, while presentational components focus solely on rendering UI elements. This separation of concerns not only makes your codebase more organized but also facilitates better testing and maintenance.
jsx // Example of a container and presentational component pair // Container Component import React, { useState } from 'react'; import { View } from 'react-native'; import Button from './Button'; const ContainerComponent = () => { const [count, setCount] = useState(0); const incrementCount = () => { setCount(count + 1); }; return ( <View> <Button title="Increment" onPress={incrementCount} /> {/* Other presentational components */} </View> ); }; export default ContainerComponent;
2. State Management
2.1 Using Redux for Centralized State
As your React Native app scales, managing the state efficiently becomes crucial. Redux offers a robust solution for centralized state management. It allows you to maintain a predictable state container that can be easily accessed and modified from different components. Redux also provides powerful debugging tools and middleware support.
jsx // Example of Redux store setup import { createStore } from 'redux'; import rootReducer from './reducers'; const store = createStore(rootReducer); export default store; jsx // Example of using Redux state in a component import React from 'react'; import { useSelector, useDispatch } from 'react-redux'; import { increment } from './actions'; const CounterComponent = () => { const count = useSelector(state => state.count); const dispatch = useDispatch(); const incrementCount = () => { dispatch(increment()); }; return ( <View> <Text>Count: {count}</Text> <Button title="Increment" onPress={incrementCount} /> </View> ); };
2.2 Exploring Context API for Lighter State Management
While Redux is excellent for complex state management, React’s Context API can be a lighter alternative for simpler scenarios. Context allows you to share state across components without prop drilling. However, keep in mind that Context might not be as performant as Redux for extremely large applications.
jsx // Example of using Context API for state management import React, { createContext, useContext, useState } from 'react'; const CountContext = createContext(); const CountProvider = ({ children }) => { const [count, setCount] = useState(0); return ( <CountContext.Provider value={{ count, setCount }}> {children} </CountContext.Provider> ); }; const useCount = () => { const context = useContext(CountContext); if (!context) { throw new Error('useCount must be used within a CountProvider'); } return context; }; export { CountProvider, useCount };
3. Performance Optimization
3.1 Virtualized Lists for Efficient Rendering
Scrollable lists are a common UI element in mobile apps, but rendering a large number of items can lead to performance issues. React Native provides a FlatList component that implements efficient rendering of large lists by only rendering the visible items. This is known as virtualization and helps maintain smooth scrolling and reduced memory consumption.
jsx // Example of using FlatList for rendering a list import React from 'react'; import { FlatList, View, Text } from 'react-native'; const Item = ({ title }) => ( <View> <Text>{title}</Text> </View> ); const ListComponent = ({ data }) => ( <FlatList data={data} renderItem={({ item }) => <Item title={item.title} />} keyExtractor={item => item.id} /> );
3.2 Code Splitting for Faster Load Times
As your React Native app grows, the bundle size can increase, leading to slower initial load times. Code splitting is a technique where you split your app’s codebase into smaller chunks that are loaded only when needed. React Native supports code splitting using dynamic imports.
jsx // Example of dynamic import for code splitting import React, { lazy, Suspense } from 'react'; import { View, Text } from 'react-native'; const LazyComponent = lazy(() => import('./LazyComponent')); const App = () => ( <View> <Text>Normal components...</Text> <Suspense fallback={<Text>Loading...</Text>}> <LazyComponent /> </Suspense> </View> );
4. Network Efficiency
4.1 Caching and Data Prefetching
In mobile apps, network requests can be a significant source of performance bottlenecks. To mitigate this, implement caching mechanisms to store frequently used data locally. Additionally, consider prefetching data that the user is likely to access, optimizing the user experience.
jsx // Example of caching using AsyncStorage import { AsyncStorage } from 'react-native'; const cacheData = async (key, data) => { try { await AsyncStorage.setItem(key, JSON.stringify(data)); } catch (error) { console.error('Error caching data:', error); } }; const getCachedData = async key => { try { const data = await AsyncStorage.getItem(key); return data ? JSON.parse(data) : null; } catch (error) { console.error('Error getting cached data:', error); return null; } };
4.2 Minimizing Network Requests with Batched Calls
Reducing the number of network requests can significantly improve your app’s performance. Instead of making multiple separate requests, consider batching requests using GraphQL or other technologies. This reduces overhead and optimizes data transfer.
jsx // Example of batching network requests using GraphQL import { useQuery } from '@apollo/client'; import { GET_USER, GET_POSTS } from './queries'; const UserProfile = ({ userId }) => { const { loading: userLoading, data: userData } = useQuery(GET_USER, { variables: { id: userId }, }); const { loading: postsLoading, data: postsData } = useQuery(GET_POSTS, { variables: { userId }, }); if (userLoading || postsLoading) { return <Text>Loading...</Text>; } // Render user profile and posts };
5. Navigation and Routing
5.1 React Navigation for Structured Navigation
Navigation is a critical aspect of mobile apps, and React Navigation provides a flexible and easy-to-use solution. It allows you to define navigation flows, handle deep linking, and transition between screens smoothly.
jsx // Example of using React Navigation Stack Navigator import { createAppContainer } from 'react-navigation'; import { createStackNavigator } from 'react-navigation-stack'; import HomeScreen from './HomeScreen'; import DetailsScreen from './DetailsScreen'; const AppNavigator = createStackNavigator({ Home: { screen: HomeScreen }, Details: { screen: DetailsScreen }, }); export default createAppContainer(AppNavigator);
5.2 Deep Linking for Better User Experience
Deep linking enables users to open specific screens within your app directly from URLs or external sources. This enhances the user experience and allows seamless transitions from other apps or web links.
jsx // Example of setting up deep linking using React Navigation import { Linking } from 'react-native'; import { NavigationActions } from 'react-navigation'; const handleDeepLink = (url) => { const route = url.replace(/.*?:\/\//g, ''); const routeName = route.split('/')[0]; if (routeName === 'details') { const id = route.split('/')[1]; const navigateAction = NavigationActions.navigate({ routeName: 'Details', params: { id }, }); this.navigator.dispatch(navigateAction); } }; // Add a listener to handle deep linking Linking.addEventListener('url', handleDeepLink);
6. Testing and Debugging
6.1 Automated Testing with Jest
Testing is crucial for maintaining the quality and scalability of your React Native app. Jest is a popular testing framework that provides tools for writing unit tests, integration tests, and snapshot tests.
jsx // Example of a Jest unit test import { sum } from './math'; test('adds 1 + 2 to equal 3', () => { expect(sum(1, 2)).toBe(3); });
6.2 Debugging with React Native Debugger
Debugging is an inevitable part of app development. React Native Debugger is a standalone debugging tool that offers a suite of features for inspecting components, state, and network requests. It’s an essential tool for identifying and resolving issues efficiently.
7. Continuous Integration and Deployment
7.1 Setting up CI/CD Pipelines
Continuous Integration (CI) and Continuous Deployment (CD) pipelines automate the process of building, testing, and deploying your app. Tools like Jenkins, CircleCI, and GitHub Actions can be configured to run tests and deploy your app whenever changes are pushed to the repository.
7.2 Over-the-Air (OTA) Updates for Seamless Deployment
As your app scales, updating it can become challenging. Over-the-Air (OTA) updates allow you to deploy updates without requiring users to manually update the app from the app store. Services like CodePush provide a way to push updates directly to users’ devices, ensuring a smooth deployment process.
Conclusion
In conclusion, building scalable React Native apps requires a combination of thoughtful architecture, optimized coding practices, and a keen focus on performance. By modularizing components, managing state efficiently, optimizing performance, and following best practices for network efficiency, navigation, testing, and deployment, you can create apps that can handle growth while delivering a seamless user experience. Stay updated with the latest developments in the React Native ecosystem to continue refining your skills and building apps that stand the test of scalability and time.
Table of Contents
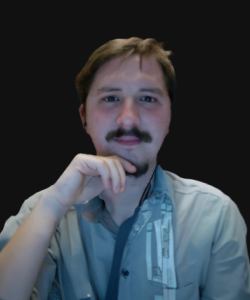
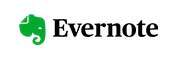