Building Attractive User Interfaces in React Native
React Native is a powerful, open-source framework that enables developers to build impressive mobile applications. Created by Facebook, this tool has been a game-changer for many businesses who choose to hire React Native developers, as it allows for the creation of both iOS and Android applications using a common codebase, saving significant time and resources.
Harnessing the power of JavaScript and React, a popular front-end library for building user interfaces, React Native offers access to native platform capabilities. This functionality enables developers to build beautiful, engaging, and high-performance applications with a truly native feel.
In this article, we’ll explore how you can, or your hired React Native developers can, create stunning user interfaces using this dynamic framework. We’ll provide practical examples and delve into an array of components, styles, and tools that can significantly enhance the look and feel of your mobile application.
Understanding React Native Components
To build interfaces in React Native, we use components. These are modular, reusable parts of the code that represent a part of the user interface.
React Native has several built-in components, such as `<View>`, `<Text>`, and `<Image>`. You can also create custom components for more specific use cases. Let’s look at some basic examples.
Basic Example:
```jsx import React from 'react'; import { View, Text } from 'react-native'; const HelloWorld = () => { return ( <View> <Text>Hello, World!</Text> </View> ); } export default HelloWorld; ```
Here, we’ve created a basic HelloWorld component using two built-in React Native components: `<View>` and `<Text>`. `<View>` is like the `<div>` element in HTML, a container that supports layout with flexbox, style, some touch handling, and accessibility controls. `<Text>` is used to display text.
Styling in React Native
Styling in React Native is quite similar to CSS in web development but uses a JavaScript object to declare style properties. Here’s an example:
```jsx import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; const StyledComponent = () => { return ( <View style={styles.container}> <Text style={styles.text}>Styled Component</Text> </View> ); } const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', backgroundColor: '#F5FCFF', }, text: { fontSize: 20, textAlign: 'center', margin: 10, color: '#333333', }, }); export default StyledComponent; ```
Here, we’ve introduced the `StyleSheet` object from React Native, which is used to abstract similar to CSS stylesheets. The styles are applied to the components using the `style` prop.
Flexbox Layout
React Native uses Flexbox for its layout, which is a well-known standard for web development. It allows us to position our components on the screen and build more complex layouts.
Here’s an example:
```jsx import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; const FlexboxExample = () => { return ( <View style={styles.container}> <View style={styles.box1} /> <View style={styles.box2} /> <View style={styles.box3} /> </View> ); } const styles = StyleSheet.create({ container: { flex: 1, flexDirection: 'row', justifyContent: 'center', alignItems: 'center', }, box1: { width: 50, height: 50, backgroundColor: 'skyblue', }, box2: { width: 50, height: 50, backgroundColor: 'steelblue', }, box3: { width: 50, height: 50, backgroundColor: 'blue', }, }); export default FlexboxExample; ```
In the above example, we’ve created a row of colored boxes using Flexbox. The `flexDirection` property controls the direction of the children items. If you want them in a column, you can use ‘column’, which is the default.
Building a Card Component
Now, let’s try to build a card component, a common UI element found in many mobile apps.
```jsx import React from 'react'; import { View, Text, Image, StyleSheet } from 'react-native'; const Card = ({ title, imageUrl }) => { return ( <View style={styles.card}> <Image style={styles.image} source={{ uri: imageUrl }} /> <View style={styles.textContainer}> <Text style={styles.title}>{title}</Text> </View> </View> ); } const styles = StyleSheet.create({ card: { borderRadius: 6, backgroundColor: '#fff', shadowColor: "#000", shadowOffset: { width: 0, height: 1 }, shadowOpacity: 0.3, shadowRadius: 2, elevation: 5, // for android marginVertical: 5, marginHorizontal: 10, }, image: { width: '100%', height: 200, borderRadius: 6, }, textContainer: { padding: 10, }, title: { fontSize: 18, fontWeight: 'bold', }, }); export default Card; ```
The `Card` component receives `title` and `imageUrl` as props and uses them to display the content. The `StyleSheet` provides the styles for the card layout and appearance.
This is a simple example of how we can create beautiful UIs using React Native. The possibilities are endless, and as we become more comfortable with the framework, we can create more complex and engaging user interfaces.
Conclusion
React Native also supports third-party libraries for creating beautiful user interfaces. Libraries like React Native Elements, NativeBase, and React Native Paper provide pre-built components that you or your team of hired React Native developers can use to create beautiful interfaces faster.
Creating beautiful UIs with React Native is a matter of understanding the basics and then applying creativity, and leveraging the vast resources available in the React Native ecosystem. Whether you’re a solo developer or you choose to hire React Native developers, the examples provided in this article only scratch the surface of what’s possible. But with these foundations, you’ll be well on your way to creating stunning mobile applications. Happy coding!
Table of Contents
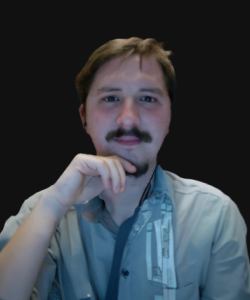
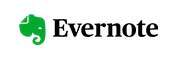