React Native and Video Streaming: Building Video Player Apps
Video streaming has become an integral part of our digital lives. Whether it’s watching movies, streaming live events, or sharing user-generated content, videos are everywhere. As a developer, you may want to harness this trend by creating your own video player app using React Native. In this comprehensive guide, we’ll walk you through the process of building a robust video player app with React Native, complete with code samples, best practices, and tips for optimizing the streaming experience.
Table of Contents
1. Why React Native for Video Streaming?
Before diving into the development process, let’s discuss why React Native is an excellent choice for building video player apps.
1.1. Cross-Platform Compatibility
React Native is renowned for its ability to develop cross-platform applications, meaning you can write code once and run it on both iOS and Android devices. This makes it a cost-effective and efficient solution for reaching a broader audience with your video player app.
1.2. Reusable Components
React Native encourages the use of reusable components, which can significantly speed up the development process. You can create a library of components for common video player features, such as play, pause, rewind, and volume control, and reuse them across your app.
1.3. Native Performance
React Native bridges the gap between native code and JavaScript, providing near-native performance. This is crucial for video streaming, where smooth playback and minimal latency are essential.
Now that we understand why React Native is a solid choice let’s get started on building our video player app.
2. Setting Up Your Development Environment
Before you can start building your video player app, you need to set up your development environment. Here are the steps to follow:
2.1. Install Node.js and npm
If you don’t already have Node.js and npm installed, download and install them from the official website (https://nodejs.org/). These are essential for running React Native projects.
2.2. Install React Native CLI
Install the React Native command-line interface (CLI) globally by running the following command:
bash npm install -g react-native-cli
2.3. Create a New React Native Project
Use the following command to create a new React Native project:
bash react-native init VideoPlayerApp
2.4. Navigate to Your Project Directory
Change your working directory to the project folder:
bash cd VideoPlayerApp
2.5. Start Your Development Server
Start the React Native development server:
bash react-native start
2.6. Launch Your App
Open a new terminal window and run your app on an emulator or a physical device:
2.6.1. For iOS:
bash react-native run-ios
2.6.2. For Android:
bash react-native run-android
With your development environment set up, you’re ready to start building your video player app.
3. Building the Video Player Component
The core of our video player app is the video player component. We’ll create a reusable component that can be easily integrated into various parts of our app. Let’s start by installing the necessary libraries and creating the component.
3.1. Install Dependencies
First, install the required dependencies for video playback:
bash npm install react-native-video npm install react-native-vector-icons npm install react-navigation
The react-native-video library provides a customizable video player component, while react-native-vector-icons allows us to add icons for play, pause, volume, etc. react-navigation is used for navigation within our app.
3.2. Create the Video Player Component
Now, let’s create the video player component. In your project directory, navigate to the src folder and create a new file called VideoPlayer.js.
jsx // src/VideoPlayer.js import React from 'react'; import { View, StyleSheet } from 'react-native'; import Video from 'react-native-video'; import Icon from 'react-native-vector-icons/FontAwesome'; const VideoPlayer = ({ source }) => { return ( <View style={styles.container}> <Video source={source} style={styles.video} controls={true} resizeMode="contain" /> <View style={styles.controls}> <Icon name="play" size={30} color="white" /> <Icon name="pause" size={30} color="white" /> <Icon name="volume-up" size={30} color="white" /> </View> </View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, video: { width: 300, height: 200, }, controls: { flexDirection: 'row', justifyContent: 'center', alignItems: 'center', marginTop: 10, }, }); export default VideoPlayer;
This component includes the video player from react-native-video and basic playback controls using react-native-vector-icons. You can customize the appearance and functionality as needed.
4. Integrating Video Playback into Your App
With the VideoPlayer component ready, let’s integrate it into your app’s navigation structure and load a video for playback.
4.1. Create Navigation Stack
In your project directory, navigate to the src folder and create a new file called App.js. This is where we’ll set up our app’s navigation stack.
jsx // src/App.js import React from 'react'; import { NavigationContainer } from '@react-navigation/native'; import { createNativeStackNavigator } from '@react-navigation/native-stack'; import VideoPlayer from './VideoPlayer'; const Stack = createNativeStackNavigator(); const App = () => { return ( <NavigationContainer> <Stack.Navigator initialRouteName="VideoPlayer"> <Stack.Screen name="VideoPlayer" component={VideoPlayer} options={{ title: 'Video Player' }} initialParams={{ source: { uri: 'YOUR_VIDEO_URL' } }} /> </Stack.Navigator> </NavigationContainer> ); }; export default App;
In this code, we use the @react-navigation/native and @react-navigation/native-stack libraries to create a basic navigation stack with a single screen that displays the VideoPlayer component. Make sure to replace ‘YOUR_VIDEO_URL’ with the actual URL of the video you want to play.
4.2. Initialize the App
To initialize your app, modify your index.js file as follows:
jsx // index.js import { AppRegistry } from 'react-native'; import App from './src/App'; import { name as appName } from './app.json'; AppRegistry.registerComponent(appName, () => App);
4.3. Test Your App
Now, run your app again to see the video player in action:
bash react-native run-ios
or
bash react-native run-android
You should be able to play the video using the custom video player component we created. You can further enhance this component by adding features like full-screen mode, video playlists, and custom styling to match your app’s design.
5. Optimizing Video Streaming
While building a video player app is one thing, optimizing video streaming is equally crucial. Here are some tips to enhance the video streaming experience for your users:
5.1. Adaptive Streaming
Implement adaptive streaming protocols like HLS (HTTP Live Streaming) and DASH (Dynamic Adaptive Streaming over HTTP) to ensure smooth playback across various network conditions and devices.
5.2. Quality Switching
Allow users to switch between different video quality options (e.g., 720p, 1080p) based on their network speed and preferences.
5.3. Caching
Implement video caching to reduce buffering and provide a seamless viewing experience, especially for users with limited bandwidth.
5.4. Analytics
Integrate analytics tools to monitor user engagement, track video performance, and gather insights for further improvements.
5.5. Video Preloading
Preload videos intelligently to minimize load times when a user selects a video for playback.
5.6. Error Handling
Handle errors gracefully by providing meaningful error messages and suggestions for troubleshooting common issues like network interruptions.
Conclusion
Building a video player app with React Native is a rewarding endeavor that allows you to tap into the ever-growing demand for video content. By following the steps outlined in this guide, you can create a robust video player component and integrate it into your mobile app, offering users a seamless video streaming experience.
Remember that video streaming optimization is an ongoing process, so continuously monitor your app’s performance and gather user feedback to make necessary improvements. With dedication and creativity, you can create a video player app that stands out in the competitive world of mobile applications. Happy coding!
Table of Contents
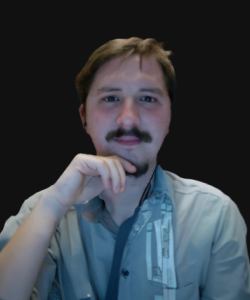
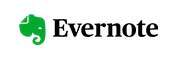